在VB程序中调用Python,可以通过使用COM接口、通过命令行调用Python脚本、使用管道进行进程间通信等方法来实现。其中,使用COM接口是一种较为简便和直接的方法,可以通过Python for Windows扩展(pywin32)来实现。接下来,我将详细介绍这三种方法。
一、使用COM接口
使用COM接口可以让VB程序直接调用Python脚本。这种方法的优点是简单易用,而且不需要进行复杂的进程间通信。
1.1 配置Python COM服务器
首先,需要确保在Python中安装了pywin32扩展,可以通过以下命令进行安装:
pip install pywin32
安装完成后,可以编写一个Python脚本,定义一个COM服务器。例如:
import win32com.server.util
class PythonCOMServer:
_public_methods_ = ['AddNumbers', 'GetGreeting']
_reg_progid_ = "PythonCOMServer.Example"
_reg_clsid_ = "{8C3F7B2C-3F57-4E5E-8A5A-5B2F3F5E3A5E}"
def AddNumbers(self, a, b):
return a + b
def GetGreeting(self, name):
return f"Hello, {name}!"
if __name__ == '__main__':
import win32com.server.register
win32com.server.register.UseCommandLine(PythonCOMServer)
运行此脚本以注册COM服务器。
1.2 在VB中调用Python COM对象
在VB中,可以通过创建COM对象来调用Python脚本中的方法。示例如下:
Sub CallPythonCOM()
Dim pythonObj As Object
Set pythonObj = CreateObject("PythonCOMServer.Example")
Dim result As Integer
result = pythonObj.AddNumbers(5, 10)
MsgBox "The sum is: " & result
Dim greeting As String
greeting = pythonObj.GetGreeting("VB User")
MsgBox greeting
End Sub
二、通过命令行调用Python脚本
2.1 使用Shell命令
另一种简单的方法是通过VB的Shell命令来执行Python脚本。这种方法适合于简单的任务或不需要与Python脚本进行复杂交互的场景。
Sub RunPythonScript()
Dim pythonExe As String
Dim scriptPath As String
pythonExe = "C:\Path\To\Python\python.exe"
scriptPath = "C:\Path\To\Script\script.py"
Shell pythonExe & " " & scriptPath, vbNormalFocus
End Sub
2.2 捕获Python脚本输出
为了捕获Python脚本的输出,可以通过重定向输出到文件,然后在VB中读取文件内容。
# script.py
print("This is the output from Python script.")
VB代码:
Sub RunPythonAndCaptureOutput()
Dim pythonExe As String
Dim scriptPath As String
Dim outputFile As String
pythonExe = "C:\Path\To\Python\python.exe"
scriptPath = "C:\Path\To\Script\script.py"
outputFile = "C:\Path\To\Output\output.txt"
Shell pythonExe & " " & scriptPath & " > " & outputFile, vbNormalFocus
' Wait for the script to complete execution
Application.Wait (Now + TimeValue("0:00:05"))
Dim fileNumber As Integer
fileNumber = FreeFile
Dim outputContent As String
Open outputFile For Input As fileNumber
Do While Not EOF(fileNumber)
Line Input #fileNumber, outputContent
MsgBox outputContent
Loop
Close fileNumber
End Sub
三、使用管道进行进程间通信
管道是一种更为高级的进程间通信方式,适用于需要在VB程序和Python脚本之间传递数据的场景。
3.1 在Python中设置管道
可以使用Python的subprocess
模块创建一个管道,用于在VB和Python之间传递数据。
# script.py
import sys
def main():
for line in sys.stdin:
data = line.strip()
if data == "exit":
break
print(f"Received from VB: {data}")
if __name__ == "__main__":
main()
3.2 在VB中通过管道调用Python
在VB中,可以使用WshShell.Exec
方法创建一个管道,并与Python脚本进行通信。
Sub RunPythonWithPipe()
Dim wshShell As Object
Set wshShell = CreateObject("WScript.Shell")
Dim pythonExe As String
Dim scriptPath As String
pythonExe = "C:\Path\To\Python\python.exe"
scriptPath = "C:\Path\To\Script\script.py"
Dim execObj As Object
Set execObj = wshShell.Exec(pythonExe & " " & scriptPath)
Dim inputStream As Object
Set inputStream = execObj.StdIn
Dim outputStream As Object
Set outputStream = execObj.StdOut
' Send data to Python script
inputStream.WriteLine "Hello from VB"
inputStream.WriteLine "exit"
' Read output from Python script
Do While Not outputStream.AtEndOfStream
MsgBox outputStream.ReadLine
Loop
End Sub
总结
通过以上三种方法,VB程序可以有效地调用Python脚本,每种方法都有其优缺点和适用场景。使用COM接口适合于需要频繁调用和复杂交互的场景,命令行调用适用于简单任务,管道适合于需要实时数据传递的场景。根据具体需求选择合适的方法,可以提高程序的效率和可维护性。无论选择哪种方法,都需要注意数据格式和错误处理,以确保程序的稳定性和可靠性。
相关问答FAQs:
如何在VB程序中与Python进行交互?
VB程序可以通过多种方式与Python进行交互。常见的方法包括使用COM接口、通过命令行调用Python脚本或利用HTTP请求与Python后端服务进行通信。选择适合的方式取决于应用程序的具体需求和架构设计。
调用Python脚本时需要注意哪些事项?
在调用Python脚本时,确保Python环境已正确安装并设置好路径。此外,脚本的权限设置也很重要,确保VB程序有权访问和执行Python脚本。最后,检查脚本的输出格式,以便VB程序能够正确解析和处理返回的数据。
有什么工具或库可以帮助VB与Python的集成?
可以考虑使用如PyWin32等库来创建COM对象,从而实现VB与Python的无缝集成。此外,使用IronPython也可以将Python代码嵌入到VB.NET应用程序中。通过这些工具,开发者可以轻松实现两者之间的功能调用和数据传输。
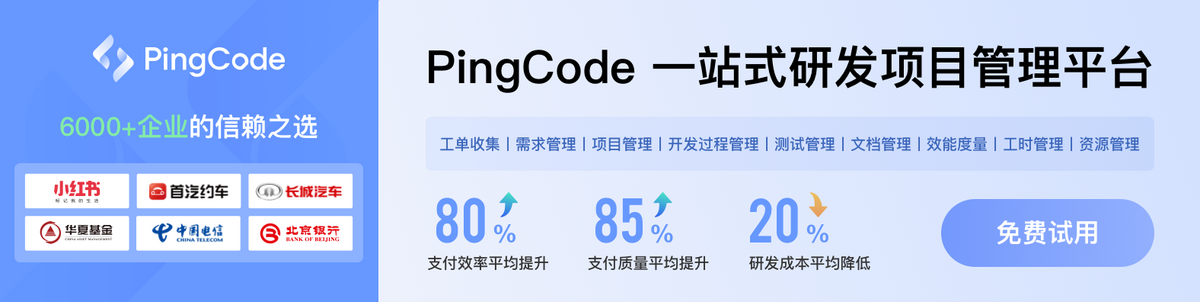