Python发送HTTP请求可以通过多种方式实现,包括使用标准库中的http.client
和urllib
模块,以及更高级的第三方库如requests
、httpx
等。这些库提供了发送GET、POST、PUT、DELETE等HTTP请求的功能,支持处理复杂的请求参数和响应数据。其中,requests
库因其简单易用和功能强大而被广泛使用。在本文中,我们将详细介绍如何使用这些库发送HTTP请求,并探讨如何处理请求参数、响应、异常及HTTP认证等场景。
一、使用标准库发送HTTP请求
Python标准库提供了基本的HTTP请求功能,通过http.client
和urllib
模块可以实现简单的请求。
1. http.client
模块
http.client
模块是Python标准库中用于HTTP协议客户端的模块,适合在需要直接控制HTTP请求细节时使用。
import http.client
def send_http_request():
conn = http.client.HTTPConnection("www.example.com")
conn.request("GET", "/")
response = conn.getresponse()
print(response.status, response.reason)
data = response.read()
print(data)
conn.close()
在上述代码中,我们创建了一个HTTP连接,并发送了一个GET请求。使用getresponse()
方法可以获取响应对象,其中包含状态码和响应数据。
2. urllib
模块
urllib
模块是Python标准库提供的另一种发送HTTP请求的方法。它提供了更高级的接口,适合处理简单的HTTP请求。
from urllib import request
def send_http_request():
response = request.urlopen('http://www.example.com')
data = response.read()
print(data)
使用urllib.request.urlopen()
方法可以轻松发送HTTP请求,并通过read()
方法读取响应数据。
二、使用requests
库发送HTTP请求
requests
库是Python中最流行的HTTP库,因其简洁的API和强大的功能而备受推崇。安装requests
库可以通过以下命令:
pip install requests
1. 发送GET请求
GET请求用于从服务器获取数据。在使用requests
库发送GET请求时,只需调用requests.get()
方法。
import requests
def send_get_request():
response = requests.get('http://www.example.com')
print(response.status_code)
print(response.text)
2. 发送POST请求
POST请求用于向服务器发送数据。在发送POST请求时,可以通过data
参数传递表单数据,或通过json
参数传递JSON数据。
import requests
def send_post_request():
data = {'key': 'value'}
response = requests.post('http://www.example.com', data=data)
print(response.status_code)
print(response.text)
def send_post_json_request():
json_data = {'key': 'value'}
response = requests.post('http://www.example.com', json=json_data)
print(response.status_code)
print(response.text)
3. 处理请求参数和响应
在发送HTTP请求时,我们可能需要传递查询参数、请求头等。requests
库提供了灵活的方式来处理这些需求。
import requests
def send_request_with_params():
params = {'key1': 'value1', 'key2': 'value2'}
headers = {'User-Agent': 'my-app/0.0.1'}
response = requests.get('http://www.example.com', params=params, headers=headers)
print(response.url)
print(response.headers)
4. 异常处理
在发送HTTP请求时,可能会遇到网络问题、请求超时等异常情况。requests
库提供了异常处理机制。
import requests
from requests.exceptions import HTTPError, Timeout
def send_request_with_exception_handling():
try:
response = requests.get('http://www.example.com', timeout=5)
response.raise_for_status()
except HTTPError as http_err:
print(f'HTTP error occurred: {http_err}')
except Timeout as timeout_err:
print(f'Timeout error occurred: {timeout_err}')
except Exception as err:
print(f'Other error occurred: {err}')
else:
print('Success!')
5. 处理HTTP认证
在某些情况下,访问的API需要进行HTTP认证。requests
库支持多种认证方式,包括Basic Auth、Digest Auth等。
import requests
from requests.auth import HTTPBasicAuth
def send_request_with_auth():
response = requests.get('http://www.example.com', auth=HTTPBasicAuth('user', 'pass'))
print(response.status_code)
三、使用httpx
库发送HTTP请求
httpx
是一个用于Python的异步HTTP客户端库,支持HTTP/1.1和HTTP/2。它提供了与requests
库类似的接口,且支持异步请求。
1. 安装httpx
可以通过以下命令安装httpx
:
pip install httpx
2. 发送异步GET请求
使用httpx
库发送异步GET请求,可以提高请求的并发性和效率。
import httpx
import asyncio
async def send_async_get_request():
async with httpx.AsyncClient() as client:
response = await client.get('http://www.example.com')
print(response.status_code)
print(response.text)
运行异步任务
asyncio.run(send_async_get_request())
3. 发送异步POST请求
与同步POST请求类似,异步POST请求也可以通过data
或json
参数发送数据。
import httpx
import asyncio
async def send_async_post_request():
async with httpx.AsyncClient() as client:
data = {'key': 'value'}
response = await client.post('http://www.example.com', data=data)
print(response.status_code)
print(response.text)
asyncio.run(send_async_post_request())
4. 异步请求的异常处理
httpx
库提供了异常处理机制,帮助开发者捕获和处理异步请求中的异常。
import httpx
import asyncio
async def send_async_request_with_exception_handling():
try:
async with httpx.AsyncClient() as client:
response = await client.get('http://www.example.com', timeout=5.0)
response.raise_for_status()
except httpx.HTTPStatusError as http_err:
print(f'HTTP error occurred: {http_err}')
except httpx.RequestError as req_err:
print(f'Request error occurred: {req_err}')
else:
print('Success!')
asyncio.run(send_async_request_with_exception_handling())
5. 处理异步HTTP认证
httpx
库同样支持异步HTTP认证,使用方式与同步请求类似。
import httpx
import asyncio
async def send_async_request_with_auth():
async with httpx.AsyncClient() as client:
response = await client.get('http://www.example.com', auth=('user', 'pass'))
print(response.status_code)
asyncio.run(send_async_request_with_auth())
四、选择合适的HTTP请求库
在选择HTTP请求库时,考虑项目需求和开发环境是很重要的。
1. 标准库 vs 第三方库
- 标准库:适合小型项目或需要简单HTTP请求的场景,不需要额外安装依赖。
- 第三方库:如
requests
和httpx
,提供更高级和易用的接口,适合复杂的HTTP请求场景。
2. 同步 vs 异步
- 同步请求:适合简单、对性能要求不高的场景。
- 异步请求:适合高并发、高性能需求的场景,
httpx
库的异步功能可以显著提升性能。
3. 功能需求
- 如果需要支持HTTP/2、异步请求、流式请求等高级特性,
httpx
是更好的选择。 - 如果需要简单易用的API和广泛的社区支持,
requests
是理想的选择。
五、总结
Python提供了多种方式发送HTTP请求,从标准库的基本实现到第三方库的高级功能,满足不同场景的需求。在选择和使用HTTP请求库时,应根据项目需求、复杂度和性能要求做出决策。无论是使用http.client
、urllib
这样的标准库,还是选择requests
、httpx
这样的第三方库,理解HTTP请求的基本概念和处理方式都是必不可少的。希望本文能够帮助你更好地掌握Python发送HTTP请求的技巧和方法。
相关问答FAQs:
如何使用Python发送GET请求?
在Python中,发送GET请求最常用的方法是使用requests
库。首先,需要安装该库:pip install requests
。然后,可以通过以下代码发送GET请求并获取响应数据:
import requests
response = requests.get('https://api.example.com/data')
print(response.status_code) # 输出响应状态码
print(response.text) # 输出响应内容
此方法简单易用,适合快速获取数据。
Python中如何发送POST请求?
发送POST请求也可以通过requests
库实现,通常用于提交数据。使用方法如下:
import requests
data = {'key': 'value'}
response = requests.post('https://api.example.com/submit', data=data)
print(response.status_code) # 输出响应状态码
print(response.json()) # 如果响应为JSON格式,可以直接解析
POST请求适合于需要上传数据的场景。
如何处理HTTP请求中的异常情况?
在发送HTTP请求时,可能会遇到网络问题或服务器错误。为了提高程序的健壮性,可以使用try-except
语句捕获异常。例如:
import requests
try:
response = requests.get('https://api.example.com/data')
response.raise_for_status() # 检查是否返回了错误状态
except requests.exceptions.HTTPError as err:
print(f'HTTP error occurred: {err}')
except requests.exceptions.RequestException as e:
print(f'Error occurred: {e}')
这种方式可以帮助您更好地处理各种请求可能出现的问题。
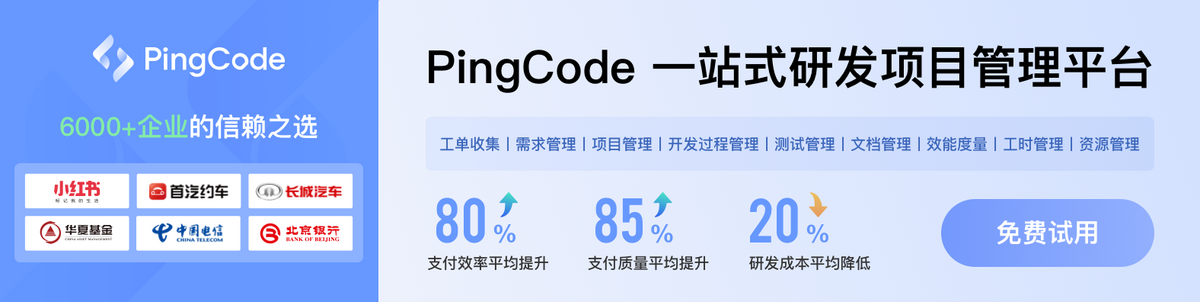