Python生成中文Unicode的方法主要有以下几种:使用内置的ord()
和chr()
函数、利用unicodedata
库、直接使用Unicode编码。其中,ord()
和chr()
函数是最常用的方法,因为它们简单易用且不需要额外的库支持。ord()
可以将字符转换为Unicode码点,而chr()
则可以将Unicode码点转换回字符。这对于处理中文字符非常有用,因为中文字符在Unicode中有一段连续的范围。接下来,我们将详细介绍这些方法的使用,并提供一些代码示例。
一、使用ord()
和chr()
函数
Python内置的ord()
和chr()
函数是处理Unicode字符的基础工具。ord()
函数用于获取字符的Unicode码点,而chr()
函数用于将Unicode码点转换为字符。
-
获取字符的Unicode码点
ord()
函数可以用来获取单个字符的Unicode码点。在Python中,中文字符的Unicode码点范围通常在\u4e00
到\u9fff
之间。通过ord()
,我们可以轻松获取中文字符的Unicode值。char = '中'
unicode_code_point = ord(char)
print(f"The Unicode code point of '{char}' is {unicode_code_point}")
上述代码将输出:
The Unicode code point of '中' is 20013
。 -
从Unicode码点生成字符
chr()
函数可以将Unicode码点转换为相应的字符。对于中文字符,只需提供对应的Unicode码点,即可生成字符。unicode_code_point = 20013
char = chr(unicode_code_point)
print(f"The character for Unicode code point {unicode_code_point} is '{char}'")
输出结果为:
The character for Unicode code point 20013 is '中'
。
二、利用unicodedata
库
Python的unicodedata
库提供了对Unicode字符的高级支持,包括获取字符名称、查找字符等功能。这对于需要处理大量Unicode字符的场景非常有用。
-
获取字符名称
unicodedata.name()
函数可用于获取给定字符的Unicode名称。import unicodedata
char = '中'
char_name = unicodedata.name(char)
print(f"The name of the character '{char}' is {char_name}")
输出结果为:
The name of the character '中' is CJK UNIFIED IDEOGRAPH-4E2D
。 -
查找字符
unicodedata.lookup()
函数可以通过名称查找对应的字符。char_name = 'CJK UNIFIED IDEOGRAPH-4E2D'
char = unicodedata.lookup(char_name)
print(f"The character for the name '{char_name}' is '{char}'")
输出结果为:
The character for the name 'CJK UNIFIED IDEOGRAPH-4E2D' is '中'
。
三、直接使用Unicode编码
在Python中,可以直接使用Unicode转义序列来表示中文字符。这种方法对于需要在代码中硬编码特定字符的场景非常方便。
-
使用Unicode转义序列
在Python字符串中,可以使用
\u
后跟四位十六进制数来表示Unicode字符。char = '\u4e2d'
print(f"The character represented by '\\u4e2d' is '{char}'")
输出结果为:
The character represented by '\u4e2d' is '中'
。 -
处理多个字符
可以将多个Unicode转义序列组合在一起,形成字符串。
string = '\u4e2d\u6587'
print(f"The string represented by '\\u4e2d\\u6587' is '{string}'")
输出结果为:
The string represented by '\u4e2d\u6587' is '中文'
。
四、使用str.encode()
和bytes.decode()
在Python中,字符串是Unicode编码的,而字节串是特定编码(如UTF-8、GBK等)的。在处理中文字符时,str.encode()
和bytes.decode()
函数可以在字符串和字节串之间进行转换。
-
字符串到字节串
使用
str.encode()
函数可以将Unicode字符串转换为字节串。常用编码包括UTF-8和GBK。string = '中文'
bytes_utf8 = string.encode('utf-8')
print(f"The UTF-8 encoded bytes of '{string}' are {bytes_utf8}")
输出结果为:
The UTF-8 encoded bytes of '中文' are b'\xe4\xb8\xad\xe6\x96\x87'
。 -
字节串到字符串
使用
bytes.decode()
函数可以将字节串转换为Unicode字符串。bytes_utf8 = b'\xe4\xb8\xad\xe6\x96\x87'
string = bytes_utf8.decode('utf-8')
print(f"The string decoded from UTF-8 bytes is '{string}'")
输出结果为:
The string decoded from UTF-8 bytes is '中文'
。
五、使用第三方库
在某些情况下,可能需要使用第三方库来处理Unicode字符。这些库通常提供了更高级的功能,如正则表达式匹配、字符集转换等。
-
使用
regex
库Python的内置正则表达式库
re
在处理Unicode字符时可能会有一些限制。regex
库提供了更强大的Unicode支持。import regex
pattern = r'\p{Han}+'
string = '这是一些中文字符'
matches = regex.findall(pattern, string)
print(f"Found Chinese characters: {matches}")
输出结果为:
Found Chinese characters: ['这是一些中文字符']
。 -
使用
chardet
库chardet
库可以自动检测文本的编码,这在处理未知编码的文本时非常有用。import chardet
bytes_unknown = b'\xe4\xb8\xad\xe6\x96\x87'
encoding_info = chardet.detect(bytes_unknown)
print(f"Detected encoding: {encoding_info['encoding']}")
输出结果可能为:
Detected encoding: utf-8
。
总结起来,Python提供了多种方法来生成和处理中文Unicode字符。根据具体需求,可以选择使用内置函数、标准库、第三方库或直接使用Unicode转义序列。对于大多数简单的场景,ord()
和chr()
函数足以满足需求,而在复杂场景下,可以借助unicodedata
或其他第三方库来实现更高级的功能。无论选择哪种方法,理解Unicode字符的编码与解码过程对于正确处理中文字符至关重要。
相关问答FAQs:
如何在Python中生成中文字符的Unicode编码?
在Python中,可以使用内置的ord()
函数来获取中文字符的Unicode编码。例如,使用ord('汉')
会返回字符“汉”的Unicode编码值。为了将这个值以十六进制格式显示,可以使用hex()
函数,如hex(ord('汉'))
,这将返回“0x6c49”,对应于“汉”的Unicode。
在Python中如何将Unicode编码转换为中文字符?
可以使用chr()
函数来将Unicode编码转换为对应的中文字符。例如,若有Unicode编码0x6c49
,可以通过chr(0x6c49)
来获取对应的字符“汉”。这对于处理Unicode编码时非常有用,特别是在需要将编码转换回可读字符时。
是否可以直接在Python字符串中使用中文字符的Unicode表示?
确实可以。在Python字符串中,可以使用\u
后接四个十六进制数字来表示Unicode字符。例如,字符串"\u6c49"
代表字符“汉”。这种方式对于在代码中插入特定字符时非常便利,尤其是在处理多语言文本时。
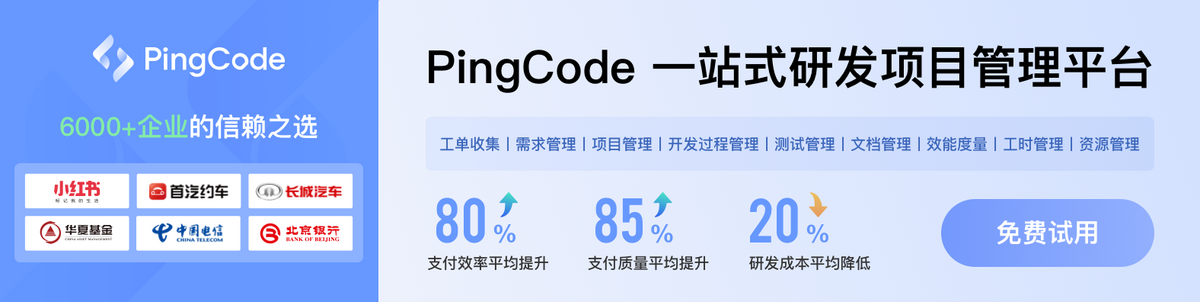