在Python中查找行序数的方式有多种,常见方法包括使用for循环和枚举、利用pandas库读取数据、通过正则表达式定位行、以及使用itertools库等。其中,使用for循环和枚举是最基本的方法,可以快速实现行序数查找。
详细描述:在Python中,可以通过for循环结合enumerate函数来查找行序数。具体实现方法如下:
# 假设我们有一个文本文件,文件名为'example.txt'
with open('example.txt', 'r') as file:
for line_number, line_content in enumerate(file, start=1):
# 打印行序数和行内容
print(f'Line {line_number}: {line_content.strip()}')
在上述代码中,enumerate()
函数能够为每一行提供一个索引,即行序数。通过这种方式,便可以轻松获取每一行的行序数。接下来,我们将深入探讨其他几种方法,帮助你在不同场景下查找行序数。
一、使用FOR循环和ENUMERATE
使用for循环结合enumerate函数是查找行序数的基础方法。此方法简单直接,适用于处理较小的文本文件或数据集。
- 基本实现
with open('example.txt', 'r') as file:
for line_number, line_content in enumerate(file, start=1):
print(f'Line {line_number}: {line_content.strip()}')
在这个例子中,enumerate()
函数为每行提供一个索引,该索引即为行序数。start=1
参数让索引从1开始,而不是默认的0。
- 查找特定行内容
如果需要查找特定内容所在的行序数,可以结合条件语句:
target_content = "search_text"
with open('example.txt', 'r') as file:
for line_number, line_content in enumerate(file, start=1):
if target_content in line_content:
print(f'Target content found at line {line_number}')
这种方法适合于快速定位文本文件中某一内容的位置。
二、利用PANDAS库读取数据
Pandas是一个强大的数据处理库,适用于处理结构化数据文件,如CSV、Excel等。使用Pandas可以更方便地处理较大的数据集。
- 读取CSV文件
import pandas as pd
df = pd.read_csv('data.csv')
for index, row in df.iterrows():
print(f'Row {index}: {row.to_dict()}')
iterrows()
函数提供了行索引和行数据,行索引即为行序数。
- 筛选特定行
Pandas还支持通过布尔索引筛选特定行:
filtered_rows = df[df['column_name'] == 'search_value']
for index, row in filtered_rows.iterrows():
print(f'Matching row at index {index}: {row.to_dict()}')
这种方法适合于从大型数据集中筛选并定位特定内容。
三、通过正则表达式定位行
正则表达式是一种强大的文本匹配工具,适用于复杂的模式查找。Python的re
模块可以帮助实现这一功能。
- 基本实现
import re
pattern = re.compile(r'your_pattern')
with open('example.txt', 'r') as file:
for line_number, line_content in enumerate(file, start=1):
if pattern.search(line_content):
print(f'Matching pattern found at line {line_number}')
使用re.compile()
编译正则表达式,然后在循环中使用search()
方法匹配行内容。
- 复杂模式匹配
正则表达式支持复杂的匹配模式,可以识别多种文本特征:
pattern = re.compile(r'\b\d{3}-\d{2}-\d{4}\b') # 匹配社会安全号码格式
with open('example.txt', 'r') as file:
for line_number, line_content in enumerate(file, start=1):
if pattern.search(line_content):
print(f'Social Security Number found at line {line_number}')
这种方法适用于查找特定格式或模式的数据。
四、使用ITERTOOLS库
itertools
库提供了高效的迭代器工具,可以在大数据集上实现高效的行序数查找。
- 使用islice迭代器
islice
函数可以创建一个切片迭代器,适用于大型文件的部分读取:
from itertools import islice
with open('example.txt', 'r') as file:
for line_number, line_content in enumerate(islice(file, 0, None), start=1):
print(f'Line {line_number}: {line_content.strip()}')
- 处理大文件
itertools
库可以帮助处理非常大的文件,避免将整个文件加载到内存中:
from itertools import islice
def find_line_in_large_file(filename, target_content):
with open(filename, 'r') as file:
for line_number, line_content in enumerate(islice(file, 0, None), start=1):
if target_content in line_content:
return line_number
return -1
line_number = find_line_in_large_file('large_file.txt', 'search_text')
print(f'Target content found at line {line_number}' if line_number != -1 else 'Content not found')
这种方法在处理大型文本文件时特别有效,节省内存并提高性能。
综上所述,Python提供了多种查找行序数的方法,每种方法都有其特定的应用场景。根据实际需求选择合适的方法,可以帮助提高代码的效率和可读性。无论是简单的文本文件查找,还是复杂的数据处理,Python都能提供强大的支持。
相关问答FAQs:
如何在Python中查找特定行的序号?
在Python中,可以使用文件操作和循环来查找特定行的序号。读取文件内容时,可以逐行遍历并使用enumerate函数来获取行号。示例代码如下:
with open('yourfile.txt', 'r') as file:
for line_number, line in enumerate(file, start=1):
if '特定内容' in line:
print(f'找到内容在第{line_number}行')
使用Pandas库如何快速查找行的序号?
如果你在处理数据文件(如CSV),Pandas库提供了更加便捷的方法。你可以将数据加载到DataFrame中,然后使用条件筛选快速找到行序号。示例代码如下:
import pandas as pd
df = pd.read_csv('yourfile.csv')
result = df[df['列名'] == '特定值'].index.tolist()
print(f'找到的行序号为: {result}')
在大型文本文件中查找行序号时,有什么优化方法?
处理大型文本文件时,效率至关重要。可以考虑逐块读取文件内容,而不是一次性加载所有行。这样可以减少内存消耗,同时加快搜索速度。使用生成器和文件迭代器可以有效提高性能。示例代码如下:
def find_line_number(file_path, search_term):
with open(file_path, 'r') as file:
for line_number, line in enumerate(file, start=1):
if search_term in line:
yield line_number
for number in find_line_number('largefile.txt', '特定内容'):
print(f'找到内容在第{number}行')
这些方法能够帮助你高效地查找行序号,根据不同需求选择合适的实现方式。
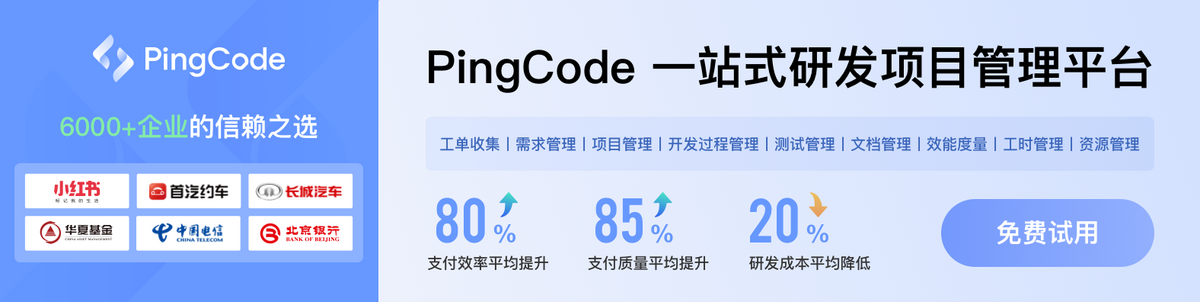