在Python程序中输出总价的方式取决于具体的应用场景和数据结构。通常,计算总价可以通过累加各个商品或服务的单价乘以其数量的结果、处理可能的折扣或税收、并最后格式化输出。在具体实现中,你可以选择使用循环、列表、字典等数据结构来存储和计算价格。以下将详细描述使用Python进行总价计算的方法。
一、使用列表和循环计算总价
在Python中,列表是一种常用的数据结构,可以用于存储商品价格和数量。通过循环遍历列表中的每个元素,计算每个商品的总价,并将其累加得到总价。
# 商品价格列表
prices = [10.99, 5.99, 3.49, 12.99]
商品数量列表
quantities = [2, 1, 4, 3]
初始化总价为0
total_price = 0
通过循环计算总价
for price, quantity in zip(prices, quantities):
total_price += price * quantity
输出总价
print(f"Total Price: ${total_price:.2f}")
在这个例子中,我们使用了两个列表,prices
和quantities
,分别存储商品的单价和数量。通过zip
函数,我们可以同时遍历两个列表,并在循环中计算每个商品的总价,然后累加到total_price
中。最后,使用格式化字符串输出总价。
二、使用字典计算总价
字典是一种灵活的数据结构,适合存储商品与其相应的价格和数量。通过字典,我们可以更方便地管理和访问数据,并且在处理复杂的数据关系时更加高效。
# 商品字典,键是商品名称,值是一个包含价格和数量的元组
items = {
"apple": (10.99, 2),
"banana": (5.99, 1),
"orange": (3.49, 4),
"grape": (12.99, 3)
}
初始化总价为0
total_price = 0
通过循环计算总价
for item, (price, quantity) in items.items():
total_price += price * quantity
输出总价
print(f"Total Price: ${total_price:.2f}")
在这个示例中,字典items
中的键是商品名称,值是一个包含价格和数量的元组。通过遍历字典的items()
方法,我们可以同时获取商品名称及其价格和数量,并在循环中计算并累加总价。
三、考虑折扣和税收
在实际应用中,商品的总价通常会受到折扣和税收的影响。为了使程序更贴近现实,我们需要在计算总价时考虑这些因素。我们可以通过定义一个函数来计算折扣和税后总价。
def calculate_total_with_discount_and_tax(prices, quantities, discount_rate=0.1, tax_rate=0.08):
# 初始化总价为0
total_price = 0
# 计算总价
for price, quantity in zip(prices, quantities):
total_price += price * quantity
# 计算折扣
discount = total_price * discount_rate
# 计算税收
tax = (total_price - discount) * tax_rate
# 计算最终总价
final_total_price = total_price - discount + tax
return final_total_price
输出总价
final_price = calculate_total_with_discount_and_tax(prices, quantities)
print(f"Total Price after discount and tax: ${final_price:.2f}")
在这个例子中,我们定义了一个函数calculate_total_with_discount_and_tax
,它接受商品价格、数量、折扣率和税率作为参数。函数首先计算初始总价,然后基于折扣率计算折扣金额,并基于税率计算税额。最后,返回扣除折扣并加上税后的最终总价。
四、使用类和方法进行更复杂的总价计算
在大型应用中,使用面向对象编程来组织代码可以提高可读性和可维护性。通过定义类和方法,我们可以将商品和价格计算逻辑封装在一起,便于复用和扩展。
class ShoppingCart:
def __init__(self):
self.items = []
def add_item(self, name, price, quantity):
self.items.append({"name": name, "price": price, "quantity": quantity})
def calculate_total(self, discount_rate=0.0, tax_rate=0.0):
total_price = 0
# 计算总价
for item in self.items:
total_price += item["price"] * item["quantity"]
# 计算折扣
discount = total_price * discount_rate
# 计算税收
tax = (total_price - discount) * tax_rate
# 计算最终总价
final_total_price = total_price - discount + tax
return final_total_price
创建购物车对象
cart = ShoppingCart()
cart.add_item("apple", 10.99, 2)
cart.add_item("banana", 5.99, 1)
cart.add_item("orange", 3.49, 4)
cart.add_item("grape", 12.99, 3)
输出总价
final_price = cart.calculate_total(discount_rate=0.1, tax_rate=0.08)
print(f"Total Price after discount and tax: ${final_price:.2f}")
在这个示例中,我们定义了一个ShoppingCart
类,包含add_item
方法用于添加商品,以及calculate_total
方法用于计算总价。这样,我们可以轻松管理购物车中的商品,并在需要时调整折扣和税率。
五、处理浮点数精度问题
在实际应用中,处理货币计算时需要特别注意浮点数的精度问题。由于浮点数的有限精度,简单的加减乘除运算可能导致不准确的结果。在这种情况下,使用Python的decimal
模块可以帮助我们提高计算精度。
from decimal import Decimal, ROUND_HALF_UP
def calculate_total_with_precision(prices, quantities, discount_rate=0.1, tax_rate=0.08):
total_price = Decimal('0.00')
# 计算总价
for price, quantity in zip(prices, quantities):
total_price += Decimal(price) * Decimal(quantity)
# 计算折扣
discount = total_price * Decimal(discount_rate)
# 计算税收
tax = (total_price - discount) * Decimal(tax_rate)
# 计算最终总价
final_total_price = total_price - discount + tax
# 使用四舍五入保留两位小数
final_total_price = final_total_price.quantize(Decimal('0.00'), rounding=ROUND_HALF_UP)
return final_total_price
输出总价
final_price = calculate_total_with_precision(prices, quantities)
print(f"Total Price with precision: ${final_price}")
在这个示例中,我们使用decimal.Decimal
类来处理价格计算。通过将数字转换为Decimal
类型,我们能够更准确地进行加减乘除运算,并使用quantize
方法进行精确的四舍五入。
六、优化和扩展程序
当需求变得复杂时,我们需要对程序进行优化和扩展。例如,支持多种货币、处理不同类型的折扣(如按商品类别折扣)、或实时更新商品价格。
- 支持多种货币
在国际化应用中,处理多种货币是一个常见需求。我们可以通过引入汇率转换功能来实现这一点。
class ShoppingCart:
def __init__(self, currency='USD'):
self.items = []
self.currency = currency
def add_item(self, name, price, quantity):
self.items.append({"name": name, "price": price, "quantity": quantity})
def set_currency(self, currency):
self.currency = currency
def calculate_total(self, exchange_rates, discount_rate=0.0, tax_rate=0.0):
total_price = Decimal('0.00')
for item in self.items:
total_price += Decimal(item["price"]) * Decimal(item["quantity"])
discount = total_price * Decimal(discount_rate)
tax = (total_price - discount) * Decimal(tax_rate)
final_total_price = total_price - discount + tax
# 转换为目标货币
final_total_price *= Decimal(exchange_rates.get(self.currency, 1.0))
final_total_price = final_total_price.quantize(Decimal('0.00'), rounding=ROUND_HALF_UP)
return final_total_price
示例使用
exchange_rates = {'USD': 1.0, 'EUR': 0.85}
cart.set_currency('EUR')
final_price = cart.calculate_total(exchange_rates, discount_rate=0.1, tax_rate=0.08)
print(f"Total Price in EUR: €{final_price}")
在这个例子中,我们在ShoppingCart
类中添加了货币属性和汇率转换功能。通过exchange_rates
字典存储不同货币的汇率,程序可以根据设置的货币进行自动转换。
- 处理不同类型的折扣
在现实中,不同商品可能有不同的折扣政策。我们可以通过扩展程序来处理基于商品类别、时间等条件的折扣。
class ShoppingCart:
def __init__(self, currency='USD'):
self.items = []
self.currency = currency
self.discounts = {}
def add_item(self, name, price, quantity, category=None):
self.items.append({"name": name, "price": price, "quantity": quantity, "category": category})
def set_discount(self, category, discount_rate):
self.discounts[category] = discount_rate
def calculate_total(self, exchange_rates, tax_rate=0.0):
total_price = Decimal('0.00')
for item in self.items:
category_discount = self.discounts.get(item["category"], 0.0)
item_price = Decimal(item["price"]) * Decimal(item["quantity"])
item_discount = item_price * Decimal(category_discount)
total_price += item_price - item_discount
tax = total_price * Decimal(tax_rate)
final_total_price = total_price + tax
final_total_price *= Decimal(exchange_rates.get(self.currency, 1.0))
final_total_price = final_total_price.quantize(Decimal('0.00'), rounding=ROUND_HALF_UP)
return final_total_price
示例使用
cart.add_item("apple", 10.99, 2, category="fruit")
cart.add_item("banana", 5.99, 1, category="fruit")
cart.set_discount("fruit", 0.1)
final_price = cart.calculate_total(exchange_rates, tax_rate=0.08)
print(f"Total Price with category discount in EUR: €{final_price}")
在这个示例中,我们在ShoppingCart
类中添加了一个discounts
字典,用于存储不同商品类别的折扣率。计算总价时,根据商品类别应用相应的折扣。
七、处理实时更新商品价格
在某些情况下,商品价格可能会实时更新。为了应对这一需求,我们需要设计一个机制来动态获取和更新商品价格。
import requests
class ShoppingCart:
def __init__(self, currency='USD'):
self.items = []
self.currency = currency
self.price_api = "https://api.example.com/get_price"
def add_item(self, name, quantity, category=None):
price = self.get_price_from_api(name)
self.items.append({"name": name, "price": price, "quantity": quantity, "category": category})
def get_price_from_api(self, product_name):
response = requests.get(f"{self.price_api}?product={product_name}")
return response.json().get('price', 0.0)
示例使用
cart = ShoppingCart()
cart.add_item("apple", 2, category="fruit")
cart.add_item("banana", 1, category="fruit")
final_price = cart.calculate_total(exchange_rates, tax_rate=0.08)
print(f"Total Price with real-time prices in EUR: €{final_price}")
在这个示例中,我们假设有一个价格API可以提供实时的商品价格。通过调用get_price_from_api
方法,我们可以在添加商品时动态获取其当前价格。
八、总结
通过以上几个部分,我们探讨了使用Python计算总价的多种方法。从简单的列表和循环,到字典和面向对象编程,再到处理复杂的折扣、税收、多种货币、以及实时价格更新,每种方法都有其适用的场景和优缺点。根据实际需求选择合适的方案,能有效提高程序的性能和可维护性。在编写涉及价格计算的程序时,务必注意浮点数精度问题,并考虑现实应用中的各种因素,如折扣、税率和汇率等。
相关问答FAQs:
如何在Python程序中计算商品的总价?
在Python中,可以通过定义一个函数来计算商品的总价。首先,您需要定义每个商品的单价和数量,然后将它们相乘并累加。示例代码如下:
def calculate_total_price(prices, quantities):
total = 0
for price, quantity in zip(prices, quantities):
total += price * quantity
return total
prices = [10.99, 5.49, 3.75]
quantities = [2, 1, 5]
total_price = calculate_total_price(prices, quantities)
print("总价为:", total_price)
这个程序将输出所有商品的总价。
在Python中如何处理不同货币的总价计算?
如果您需要计算不同货币的总价,可以使用汇率进行转换。可以定义一个汇率字典,然后在计算总价时将金额转换为目标货币。示例代码如下:
exchange_rates = {'USD': 1, 'EUR': 0.85, 'CNY': 6.45}
def calculate_total_price_with_currency(prices, quantities, currency):
total = 0
for price, quantity in zip(prices, quantities):
total += price * quantity
return total * exchange_rates[currency]
prices = [10.99, 5.49, 3.75]
quantities = [2, 1, 5]
total_price_usd = calculate_total_price_with_currency(prices, quantities, 'USD')
print("总价为:", total_price_usd, "USD")
此代码将以指定货币输出总价。
如何在Python程序中添加税费计算?
在计算总价时,税费通常是一个重要因素。可以通过在总价的基础上增加相应的税率来实现。示例代码如下:
def calculate_total_price_with_tax(prices, quantities, tax_rate):
total = 0
for price, quantity in zip(prices, quantities):
total += price * quantity
total_with_tax = total * (1 + tax_rate)
return total_with_tax
prices = [10.99, 5.49, 3.75]
quantities = [2, 1, 5]
tax_rate = 0.07 # 7%的税率
total_price_with_tax = calculate_total_price_with_tax(prices, quantities, tax_rate)
print("总价(含税)为:", total_price_with_tax)
此代码计算并输出包含税费的总价。
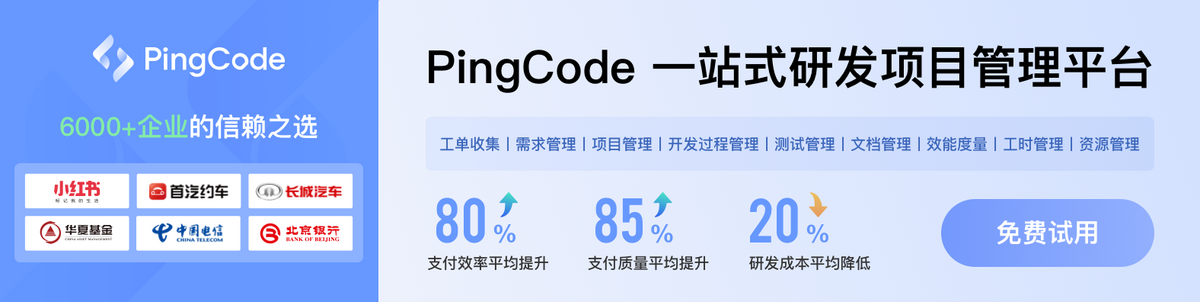