Python伪装MAC地址的步骤:使用subprocess
库、修改网络接口、重启网络接口
伪装MAC地址是通过修改网络适配器的物理地址来实现的。Python可以通过调用操作系统命令来实现这一操作。以下是详细步骤和示例代码。
一、使用subprocess
库
Python中的subprocess
库可以用来执行系统命令,这对于伪装MAC地址非常有用。通过subprocess
,我们可以调用系统命令来修改网络接口的MAC地址。
import subprocess
def change_mac(interface, new_mac):
subprocess.call(["sudo", "ifconfig", interface, "down"])
subprocess.call(["sudo", "ifconfig", interface, "hw", "ether", new_mac])
subprocess.call(["sudo", "ifconfig", interface, "up"])
在这个函数中,我们使用subprocess.call
来调用ifconfig
命令。首先,我们将网络接口关闭,然后将其MAC地址更改为新的地址,最后重新启用网络接口。
二、修改网络接口
要伪装MAC地址,首先需要确定哪个网络接口需要修改。常见的网络接口包括eth0
(以太网)和wlan0
(无线网络)。可以通过以下命令查看当前系统中的网络接口及其MAC地址:
ifconfig
运行上述命令后,您将看到类似以下输出:
eth0 Link encap:Ethernet HWaddr 00:1a:2b:3c:4d:5e
inet addr:192.168.0.101 Bcast:192.168.0.255 Mask:255.255.255.0
...
wlan0 Link encap:Ethernet HWaddr 00:1a:2b:3c:4d:5f
inet addr:192.168.0.102 Bcast:192.168.0.255 Mask:255.255.255.0
...
假设我们想要修改eth0
的MAC地址,可以使用以下代码:
interface = "eth0"
new_mac = "02:42:ac:11:00:02"
change_mac(interface, new_mac)
三、重启网络接口
在修改MAC地址之后,重启网络接口是为了确保更改生效。这也是通过subprocess
库调用ifconfig
命令来实现的。在上面的示例函数中,我们已经包含了这一步骤。
示例代码
以下是一个完整的示例代码,展示了如何使用Python来伪装MAC地址:
import subprocess
def get_current_mac(interface):
result = subprocess.check_output(["ifconfig", interface])
result = result.decode('utf-8')
for line in result.split('\n'):
if 'ether' in line:
return line.strip().split(' ')[1]
def change_mac(interface, new_mac):
print(f"[+] Changing MAC address for {interface} to {new_mac}")
subprocess.call(["sudo", "ifconfig", interface, "down"])
subprocess.call(["sudo", "ifconfig", interface, "hw", "ether", new_mac])
subprocess.call(["sudo", "ifconfig", interface, "up"])
interface = "eth0"
new_mac = "02:42:ac:11:00:02"
current_mac = get_current_mac(interface)
print(f"Current MAC: {current_mac}")
change_mac(interface, new_mac)
current_mac = get_current_mac(interface)
print(f"New MAC: {current_mac}")
注意事项
- 权限问题:由于更改网络接口的MAC地址需要管理员权限,因此需要以root用户身份运行Python脚本,或者在命令前面加上
sudo
。 - 兼容性:上面的示例代码适用于类Unix系统(如Linux和macOS)。如果是在Windows系统上,需要使用不同的方法来修改MAC地址。
- 合法性:在某些国家或地区,伪装MAC地址可能违反法律法规。在进行此操作之前,请确保了解并遵守相关的法律法规。
总结
通过使用Python的subprocess
库,我们可以轻松地调用系统命令来修改网络接口的MAC地址。关键步骤包括关闭网络接口、修改MAC地址以及重启网络接口。注意在执行这些操作时需要管理员权限,并确保遵守相关法律法规。
相关问答FAQs:
如何使用Python更改我的MAC地址?
在Python中,可以使用subprocess
模块来调用系统命令,更改MAC地址。首先需要使用ifconfig
(Linux)或netsh
(Windows)命令。通过Python脚本,可以临时更改网络接口的MAC地址。确保在执行之前具有管理员权限,并了解网络配置的影响。
伪装MAC地址是否会影响我的网络连接?
伪装MAC地址可能会影响网络连接,因为某些网络设备或服务可能会根据MAC地址进行身份验证。如果您在公共Wi-Fi或公司网络中使用伪装的MAC地址,可能会导致连接问题或被网络管理员检测到。因此,在进行此操作之前,请考虑潜在的后果和风险。
使用Python伪装MAC地址是否合法?
在许多国家和地区,伪装MAC地址本身并不违法,但它可能违反某些网络服务的使用条款。在进行此操作之前,请务必了解您所在地区的法律法规以及您连接的网络的政策。使用伪装的MAC地址可能会导致账户封禁或其他后果,因此建议谨慎行事。
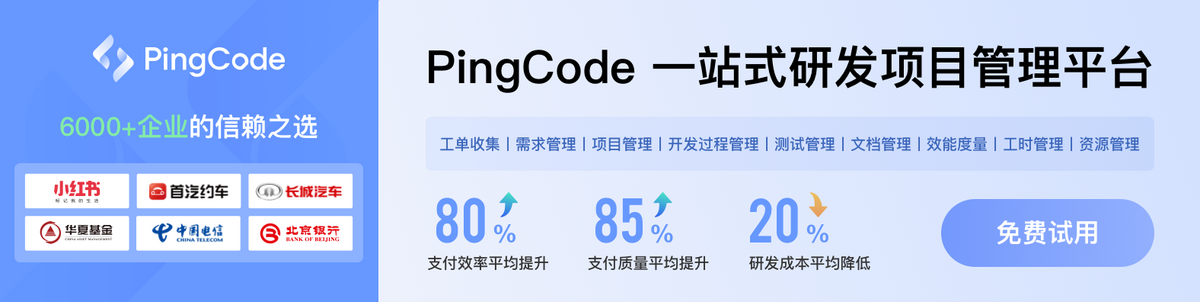