在Python中,只执行一次代码的方法包括使用全局变量、函数装饰器、单例模式等。其中使用函数装饰器是比较推荐的方法,下面将详细介绍函数装饰器的方法。
在Python编程中,有时候我们需要确保某段代码只被执行一次,无论该代码块被调用多少次。以下是一些常见的方法和实现方式:
一、全局变量
通过全局变量可以控制代码的执行次数。全局变量的作用是记录代码是否已经执行过,如果已经执行过则不会再执行。
executed = False
def execute_once():
global executed
if not executed:
print("This code runs only once.")
executed = True
Test
execute_once()
execute_once()
在这个例子中,executed
变量在第一次调用execute_once
函数时被设置为True
,因此后续的调用将不会再执行代码块内的内容。
二、函数装饰器
函数装饰器是一种优雅的解决方案,可以封装函数的行为,使其只执行一次。
def run_once(f):
def wrapper(*args, kwargs):
if not wrapper.has_run:
result = f(*args, kwargs)
wrapper.has_run = True
return result
wrapper.has_run = False
return wrapper
@run_once
def my_function():
print("This code runs only once.")
Test
my_function()
my_function()
在这个例子中,装饰器run_once
确保my_function
函数只执行一次。第一次调用时,wrapper.has_run
被设置为True
,因此后续调用将不会再执行。
三、单例模式
单例模式确保一个类只有一个实例,并提供一个全局访问点。通过这种方式,可以确保某些代码只执行一次。
class Singleton:
_instance = None
def __new__(cls, *args, kwargs):
if not cls._instance:
cls._instance = super().__new__(cls, *args, kwargs)
return cls._instance
def run_once(self):
if not hasattr(self, '_has_run'):
print("This code runs only once.")
self._has_run = True
Test
singleton = Singleton()
singleton.run_once()
singleton.run_once()
在这个例子中,Singleton
类通过__new__
方法确保只有一个实例存在,run_once
方法确保代码只执行一次。
四、使用缓存(Memoization)
缓存是一种优化技术,可以存储函数的计算结果,避免重复计算。通过缓存机制可以确保函数只执行一次。
from functools import lru_cache
@lru_cache(maxsize=1)
def my_function():
print("This code runs only once.")
return "result"
Test
my_function()
my_function()
在这个例子中,lru_cache
装饰器确保my_function
只执行一次,后续调用将直接返回缓存的结果。
五、上下文管理器
上下文管理器可以用于管理资源的使用,通过上下文管理器可以确保某些代码只执行一次。
class RunOnceContext:
def __init__(self):
self.has_run = False
def __enter__(self):
if not self.has_run:
self.has_run = True
return True
return False
def __exit__(self, exc_type, exc_val, exc_tb):
pass
Test
with RunOnceContext() as run_once:
if run_once:
print("This code runs only once.")
with RunOnceContext() as run_once:
if run_once:
print("This code will not run.")
在这个例子中,RunOnceContext
类通过上下文管理器机制确保代码块只执行一次。
六、线程安全的单例模式
在多线程环境中,确保代码只执行一次需要考虑线程安全问题。可以使用线程锁来确保线程安全。
import threading
class ThreadSafeSingleton:
_instance = None
_lock = threading.Lock()
def __new__(cls, *args, kwargs):
with cls._lock:
if not cls._instance:
cls._instance = super().__new__(cls, *args, kwargs)
return cls._instance
def run_once(self):
if not hasattr(self, '_has_run'):
print("This code runs only once.")
self._has_run = True
Test
singleton = ThreadSafeSingleton()
singleton.run_once()
singleton.run_once()
在这个例子中,ThreadSafeSingleton
类通过线程锁确保在多线程环境下只有一个实例存在,并确保代码只执行一次。
总结:
通过以上几种方法可以确保Python代码只执行一次。使用全局变量、函数装饰器、单例模式、缓存、上下文管理器以及线程安全的单例模式都可以实现这一目标。具体选择哪种方法可以根据实际需求和应用场景来决定。无论选择哪种方法,都要注意代码的可读性和维护性,确保代码简洁、清晰、易于理解。
相关问答FAQs:
如何在Python中确保代码只执行一次?
在Python中,可以使用模块级别的代码或者函数装饰器来确保某段代码只执行一次。常见的做法是将代码放置在一个函数中,并使用全局变量或单例模式来控制执行。还可以利用threading
模块中的Lock
类来确保多线程环境下的单次执行。
在Python中使用类的静态方法来执行一次代码有什么好处?
通过类的静态方法,您可以将执行逻辑与类的其他功能解耦,提供更清晰的代码结构。这种方式不仅能实现代码只执行一次的需求,还能方便后续的维护和扩展。使用类的静态方法时,可以通过类属性来跟踪是否已执行过代码。
如何在多线程环境中确保某段代码只执行一次?
在多线程环境中,可以使用threading.Lock
类来确保某段代码的线程安全性。通过在代码块前后加锁和解锁,可以防止多个线程同时执行该代码,从而确保其只执行一次。使用上下文管理器可以使锁的管理更加简洁和安全。
有没有简单的方法在脚本执行时只运行一次特定代码?
可以通过将特定代码放入一个函数中,并在脚本开始时调用该函数。结合使用全局变量来标识该函数是否已被调用,可以有效地控制代码的执行。此方法简单易行,适合小型脚本和简单的应用场景。
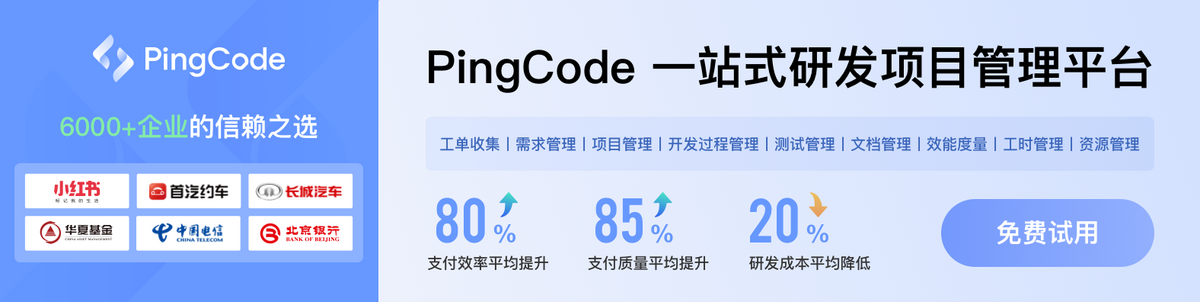