在Python中引入math库可以使用import语句。导入math库后,可以使用库中的各种数学函数和常量,例如:sqrt、sin、cos、pi等。具体方法如下:
import math
在使用math库时,通过调用库中的函数和常量,可以方便地进行各种数学计算和操作。以下是一些常见的math库函数和用法:
- 平方根计算:使用math.sqrt()函数
import math
number = 16
sqrt_value = math.sqrt(number)
print("The square root of", number, "is", sqrt_value)
- 三角函数:使用math.sin()、math.cos()、math.tan()函数
import math
angle = math.pi / 4 # 45 degrees in radians
sin_value = math.sin(angle)
cos_value = math.cos(angle)
tan_value = math.tan(angle)
print("Sine of 45 degrees is", sin_value)
print("Cosine of 45 degrees is", cos_value)
print("Tangent of 45 degrees is", tan_value)
- 常量:使用math.pi和math.e
import math
pi_value = math.pi
e_value = math.e
print("The value of pi is", pi_value)
print("The value of e is", e_value)
- 对数函数:使用math.log()和math.log10()函数
import math
number = 100
log_value = math.log(number)
log10_value = math.log10(number)
print("The natural logarithm of", number, "is", log_value)
print("The base-10 logarithm of", number, "is", log10_value)
- 指数函数:使用math.exp()函数
import math
number = 2
exp_value = math.exp(number)
print("The value of e raised to the power of", number, "is", exp_value)
- 角度转换:使用math.degrees()和math.radians()函数
import math
radian = math.pi
degree = math.degrees(radian)
print("The value of pi radians in degrees is", degree)
degree = 180
radian = math.radians(degree)
print("The value of 180 degrees in radians is", radian)
通过引入math库,可以大大简化数学计算的代码,使其更加简洁和高效。在实际应用中,math库的功能非常强大,可以满足大多数数学计算的需求。
相关问答FAQs:
如何在Python中导入math库?
在Python中,导入math库非常简单。你只需在代码的开始部分使用import math
语句。这样,你就可以使用math库中的各种数学函数和常量,比如math.sqrt()
计算平方根,或者使用math.pi
获取圆周率的值。
使用math库时,有哪些常见的数学函数?
math库提供了多种实用的数学函数,包括但不限于:math.sqrt()
(平方根),math.pow()
(幂运算),math.sin()
(正弦),math.cos()
(余弦),以及math.factorial()
(阶乘)等。这些函数可以大大简化复杂的数学计算。
math库中是否有常量可以使用?
是的,math库中包含多个常量,例如math.pi
和math.e
。math.pi
代表圆周率(π)的值,约为3.14159,而math.e
则是自然对数的底数,约为2.71828。这些常量在进行科学计算时非常有用。
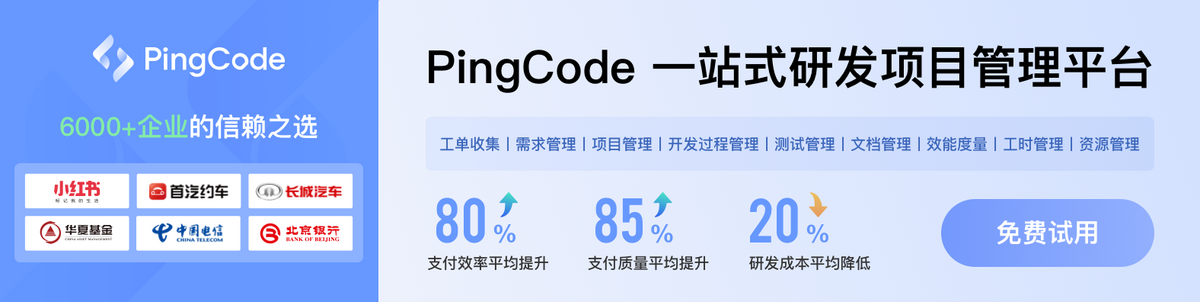