Python跳一跳解决方案包括:图像识别、距离计算、模拟点击、调试与优化。其中图像识别是关键步骤,通过图像识别可以准确定位跳跃的起点和终点,这是整个解决方案的基础。
一、图像识别
图像识别是解决Python跳一跳的第一步,通过分析屏幕截图来确定跳跃的起点和终点。常用的图像识别方法包括模板匹配和边缘检测。
1、模板匹配
模板匹配是一种简单而有效的图像识别方法。通过预先定义的模板,匹配屏幕截图中的相似部分。具体实现方法如下:
- 获取屏幕截图:使用Python库如Pillow或OpenCV从设备屏幕获取截图。
- 定义模板:选择跳跃过程中需要识别的物体,如小人和方块的图像,作为模板。
- 匹配模板:使用OpenCV的
matchTemplate
函数在截图中搜索模板的位置。 - 确定位置:通过
minMaxLoc
函数确定匹配的位置。
import cv2
import numpy as np
Load the screenshot and template
screenshot = cv2.imread('screenshot.png')
template = cv2.imread('template.png', 0)
Convert screenshot to grayscale
gray_screenshot = cv2.cvtColor(screenshot, cv2.COLOR_BGR2GRAY)
Perform template matching
result = cv2.matchTemplate(gray_screenshot, template, cv2.TM_CCOEFF_NORMED)
Get the location of the best match
min_val, max_val, min_loc, max_loc = cv2.minMaxLoc(result)
Draw a rectangle around the matched region
top_left = max_loc
bottom_right = (top_left[0] + template.shape[1], top_left[1] + template.shape[0])
cv2.rectangle(screenshot, top_left, bottom_right, (0, 255, 0), 2)
Save the result
cv2.imwrite('result.png', screenshot)
2、边缘检测
边缘检测是一种有效的图像处理技术,通过检测图像中的边缘,可以识别出需要跳跃的物体。常用的边缘检测方法包括Canny边缘检测。
import cv2
Load the screenshot
screenshot = cv2.imread('screenshot.png')
Convert to grayscale
gray_screenshot = cv2.cvtColor(screenshot, cv2.COLOR_BGR2GRAY)
Perform Canny edge detection
edges = cv2.Canny(gray_screenshot, 50, 150)
Save the result
cv2.imwrite('edges.png', edges)
二、距离计算
通过图像识别确定起点和终点后,需要计算跳跃的距离。距离计算通常包括两部分:起点位置和终点位置。
1、起点位置
起点位置是小人的位置,通过图像识别确定。假设小人的位置为(x1, y1)
。
2、终点位置
终点位置是目标方块的位置,通过图像识别确定。假设目标方块的位置为(x2, y2)
。
3、计算距离
使用欧几里得距离公式计算起点和终点之间的距离:
[ \text{distance} = \sqrt{(x2 – x1)^2 + (y2 – y1)^2} ]
import math
Coordinates of the starting and ending points
x1, y1 = 100, 200
x2, y2 = 300, 400
Calculate the distance
distance = math.sqrt((x2 - x1)<strong>2 + (y2 - y1)</strong>2)
print('Distance:', distance)
三、模拟点击
模拟点击是通过编程方式在设备上模拟触摸屏幕,以实现自动跳跃。常用的模拟点击方法包括使用ADB命令和Python库pyautogui
。
1、ADB命令
通过ADB命令可以在Android设备上模拟点击。
adb shell input swipe x1 y1 x2 y2 time
2、Python库pyautogui
使用pyautogui
库可以在电脑上模拟点击。
import pyautogui
Coordinates and duration
x, y = 300, 400
duration = 0.5
Perform the click
pyautogui.click(x, y, duration=duration)
四、调试与优化
在实现Python跳一跳解决方案后,需要进行调试与优化,以提高准确性和成功率。
1、调试
通过打印中间结果和绘制图像进行调试,确保图像识别和距离计算的准确性。
2、优化
通过调整图像识别参数、距离计算公式和点击时间进行优化,提高跳跃的成功率。
import cv2
import numpy as np
import pyautogui
import math
Load the screenshot and templates
screenshot = cv2.imread('screenshot.png')
template_start = cv2.imread('template_start.png', 0)
template_end = cv2.imread('template_end.png', 0)
Convert screenshot to grayscale
gray_screenshot = cv2.cvtColor(screenshot, cv2.COLOR_BGR2GRAY)
Perform template matching for start and end templates
result_start = cv2.matchTemplate(gray_screenshot, template_start, cv2.TM_CCOEFF_NORMED)
result_end = cv2.matchTemplate(gray_screenshot, template_end, cv2.TM_CCOEFF_NORMED)
Get the location of the best matches
_, _, _, max_loc_start = cv2.minMaxLoc(result_start)
_, _, _, max_loc_end = cv2.minMaxLoc(result_end)
Coordinates of the starting and ending points
x1, y1 = max_loc_start
x2, y2 = max_loc_end
Calculate the distance
distance = math.sqrt((x2 - x1)<strong>2 + (y2 - y1)</strong>2)
Calculate the duration based on distance (example coefficient)
duration = distance * 0.002
Perform the click
pyautogui.click(x1, y1, duration=duration)
Draw rectangles around matched regions for debugging
cv2.rectangle(screenshot, max_loc_start, (max_loc_start[0] + template_start.shape[1], max_loc_start[1] + template_start.shape[0]), (0, 255, 0), 2)
cv2.rectangle(screenshot, max_loc_end, (max_loc_end[0] + template_end.shape[1], max_loc_end[1] + template_end.shape[0]), (0, 255, 0), 2)
Save the result for debugging
cv2.imwrite('result.png', screenshot)
通过上述步骤,可以实现Python跳一跳的自动化解决方案。这个过程包括图像识别、距离计算、模拟点击以及调试与优化。每个步骤都需要仔细调试和优化,以确保最终方案的准确性和成功率。
相关问答FAQs:
如何使用Python实现“跳一跳”游戏的自动化?
要实现“跳一跳”游戏的自动化,您需要使用图像识别和模拟点击功能。可以使用OpenCV库来识别游戏界面中的元素,并使用pyautogui库模拟点击操作。通过不断调整跳跃距离和时间,可以实现自适应的游戏玩法。
在Python中如何优化“跳一跳”的跳跃策略?
优化“跳一跳”的跳跃策略涉及到对游戏机制的深入理解。您可以通过收集跳跃数据来分析最佳跳跃距离,并使用机器学习算法来预测最优的跳跃时间。这种方法能有效提高游戏的胜率。
是否有现成的Python库可以帮助开发“跳一跳”游戏的模拟器?
有一些Python库可以帮助您创建“跳一跳”游戏的模拟器,比如Pygame和PyOpenGL。Pygame非常适合快速开发2D游戏,而PyOpenGL则更适合需要3D效果的游戏。利用这些库,您可以快速构建和测试自己的游戏逻辑。
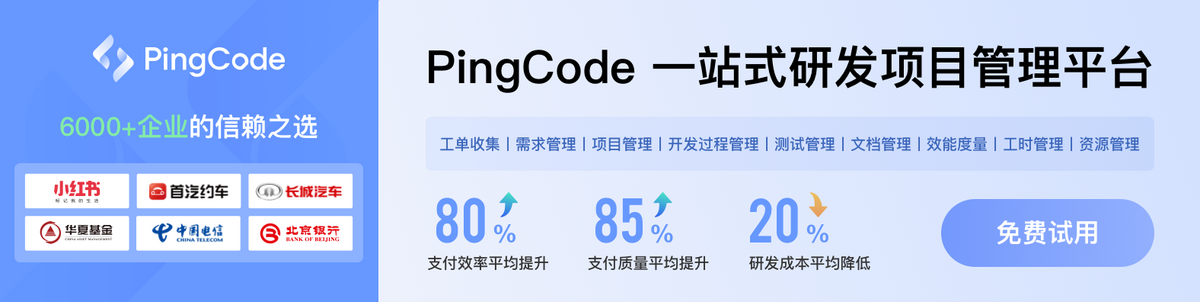