如何用Python画一辆校车
用Python画一辆校车可以通过使用图形库来实现,其中最常用的是turtle
库。Python的turtle
库非常适合做简单的图形和绘制工作,具有简单易用的接口,适合初学者。下面是一段详细的代码示例及步骤,教你如何用Python画一辆校车。
import turtle
def draw_rectangle(color, x, y, width, height):
turtle.penup()
turtle.goto(x, y)
turtle.pendown()
turtle.color(color)
turtle.begin_fill()
for _ in range(2):
turtle.forward(width)
turtle.right(90)
turtle.forward(height)
turtle.right(90)
turtle.end_fill()
def draw_wheel(x, y, radius):
turtle.penup()
turtle.goto(x, y)
turtle.pendown()
turtle.color("black")
turtle.begin_fill()
turtle.circle(radius)
turtle.end_fill()
def draw_window(x, y, width, height):
draw_rectangle("white", x, y, width, height)
turtle.color("black")
turtle.penup()
turtle.goto(x, y)
turtle.pendown()
for _ in range(2):
turtle.forward(width)
turtle.right(90)
turtle.forward(height)
turtle.right(90)
def draw_school_bus():
# Draw the body of the bus
draw_rectangle("yellow", -150, 0, 300, 100)
# Draw the windows of the bus
draw_window(-130, -20, 40, 40)
draw_window(-70, -20, 40, 40)
draw_window(-10, -20, 40, 40)
draw_window(50, -20, 40, 40)
# Draw the wheels of the bus
draw_wheel(-100, -100, 20)
draw_wheel(50, -100, 20)
# Draw the door of the bus
draw_rectangle("blue", 80, -20, 30, 60)
# Draw the stop sign
turtle.penup()
turtle.goto(150, -10)
turtle.pendown()
turtle.color("red")
turtle.write("STOP", font=("Arial", 16, "normal"))
Set up the turtle environment
turtle.speed(3)
turtle.bgcolor("white")
Draw the school bus
draw_school_bus()
Hide turtle and display the result
turtle.hideturtle()
turtle.done()
一、准备工作
首先,需要安装Python并确保安装了turtle
库。大多数Python安装包中会自带turtle
库,如果没有,可以通过命令pip install PythonTurtle
来安装。
二、绘制校车主体
校车的主体是一个长方形,可以通过turtle
库的begin_fill
和end_fill
方法来填充颜色。我们使用draw_rectangle
函数来绘制长方形,并在函数中定义颜色、坐标、宽度和高度。
三、绘制车窗
校车的车窗也是长方形,使用draw_window
函数来绘制多个车窗。需要注意的是,车窗的颜色通常是白色,并且在绘制完之后,需要用黑色的线条来描边。
四、绘制车轮
车轮是圆形,通过turtle
库的circle
方法来绘制。我们使用draw_wheel
函数来定义车轮的位置和大小,并用黑色填充颜色。
五、绘制车门和停靠标志
车门是一个蓝色的长方形,使用draw_rectangle
函数来绘制。停靠标志是一个红色的文字,使用turtle.write
方法来绘制"STOP"字样。
六、设置turtle环境
在绘制之前,需要设置turtle
的速度和背景颜色。绘制完成后,通过hideturtle
方法隐藏turtle
,并通过done
方法显示绘制结果。
结论
通过以上步骤,我们成功地绘制了一辆校车。使用turtle
库可以方便地进行图形绘制,并且代码简洁明了,适合初学者。希望这篇文章能帮助你理解如何用Python绘制简单的图形。
相关问答FAQs:
如何用Python绘制简单的校车图形?
绘制校车可以使用Python的图形库,如turtle或matplotlib。选择一个库后,您可以开始绘制矩形作为车身,并添加细节,如窗户、轮子和车灯,以使其看起来更像校车。可以通过设置不同的颜色和形状来增强视觉效果。
我需要安装哪些库才能使用Python绘制图形?
如果您选择使用turtle库,只需确保Python环境中已安装该库,因为它通常是Python的标准库之一。对于matplotlib,您可以使用pip命令进行安装:pip install matplotlib
。安装完成后,您就可以开始绘制校车。
除了校车,还有哪些图形可以用Python绘制?
Python的图形库非常强大,您可以绘制多种图形,如房屋、树木、动物和各种交通工具。通过组合不同的形状和颜色,您可以创造出复杂的图像,甚至可以尝试创建简单的动画效果。探索不同的绘图功能可以提高您的编程和艺术技巧。
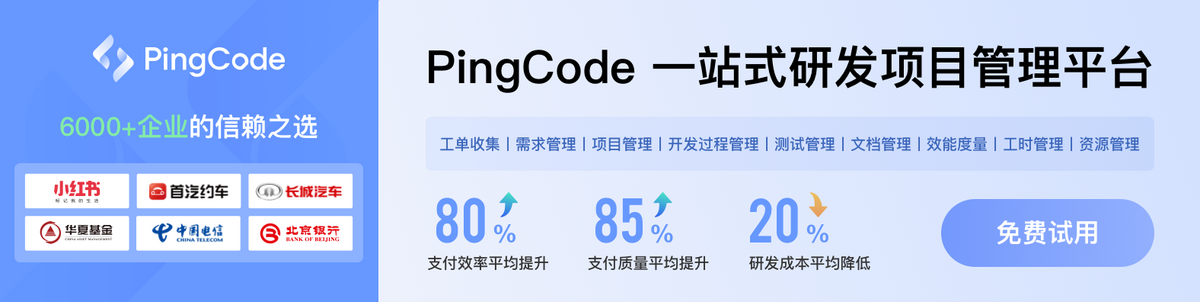