在Python CGI编程中处理图片,可以通过以下几种方法:使用cgi
模块处理上传、使用Pillow
库处理图片、将图片保存到服务器、设置正确的MIME类型。这些方法可以有效地处理图片上传和操作。在详细描述其中一点,即使用cgi
模块处理上传时,必须确保正确解析和存储上传的图片文件。
一、使用CGI模块处理上传
在Python CGI编程中,处理图片上传的第一步是解析上传的文件数据。这里我们主要使用cgi
模块来处理。cgi
模块可以帮助我们处理来自HTML表单的文件上传。
在处理上传文件时,需要注意以下几点:
- 使用
cgi.FieldStorage()
来获取表单数据。 - 检查上传的文件是否存在。
- 读取并保存上传的文件。
import cgi
import os
def save_uploaded_file():
form = cgi.FieldStorage()
fileitem = form['file']
if fileitem.filename:
# 设置文件保存路径
filepath = os.path.join('/path/to/upload/directory', os.path.basename(fileitem.filename))
# 写入文件
with open(filepath, 'wb') as f:
f.write(fileitem.file.read())
print("Content-type:text/html\r\n\r\n")
print(f"<html><body><h2>File '{fileitem.filename}' uploaded successfully!</h2></body></html>")
else:
print("Content-type:text/html\r\n\r\n")
print("<html><body><h2>No file was uploaded</h2></body></html>")
save_uploaded_file()
二、使用Pillow库处理图片
Pillow库是Python中一个强大的图像处理库,可以对图片进行各种操作,如裁剪、旋转、调整大小等。在处理上传的图片后,您可以使用Pillow库对图片进行进一步处理。
- 安装Pillow库:
pip install pillow
- 使用Pillow库处理图片:
from PIL import Image
def process_image(filepath):
with Image.open(filepath) as img:
# 示例操作:将图片转换为灰度图像
grayscale_img = img.convert("L")
grayscale_img.save('/path/to/upload/directory/grayscale_image.png')
print("Image processed and saved as grayscale_image.png")
三、将图片保存到服务器
保存上传的图片文件到服务器是处理上传文件的一个重要步骤。在保存文件时,需要确保文件路径的安全性,并适当地处理文件名重复的问题。
- 确保文件路径的安全性:
import os
def secure_filename(filename):
return os.path.basename(filename)
- 处理文件名重复的问题:
import os
def save_file_with_unique_name(directory, filename):
base, ext = os.path.splitext(filename)
counter = 1
new_filename = filename
while os.path.exists(os.path.join(directory, new_filename)):
new_filename = f"{base}_{counter}{ext}"
counter += 1
return new_filename
filepath = os.path.join('/path/to/upload/directory', save_file_with_unique_name('/path/to/upload/directory', fileitem.filename))
四、设置正确的MIME类型
在处理图片上传和下载时,设置正确的MIME类型是非常重要的。这可以确保浏览器能够正确地处理图片文件。
- 设置MIME类型:
import mimetypes
def get_mime_type(filepath):
mime_type, _ = mimetypes.guess_type(filepath)
return mime_type
def serve_image(filepath):
mime_type = get_mime_type(filepath)
if mime_type:
print(f"Content-type: {mime_type}\r\n\r\n")
with open(filepath, 'rb') as f:
print(f.read())
else:
print("Content-type: text/html\r\n\r\n")
print("<html><body><h2>Could not determine MIME type</h2></body></html>")
serve_image('/path/to/upload/directory/grayscale_image.png')
五、使用HTML表单上传图片
为了让用户能够上传图片,需要在HTML表单中设置正确的属性。特别是需要设置enctype="multipart/form-data"
,这样表单才能正确地上传文件。
<!DOCTYPE html>
<html>
<body>
<h2>Upload Image</h2>
<form action="/cgi-bin/upload.py" method="post" enctype="multipart/form-data">
Select image to upload:
<input type="file" name="file">
<input type="submit" value="Upload Image" name="submit">
</form>
</body>
</html>
六、处理错误和安全问题
在处理图片上传时,错误处理和安全性是非常重要的。需要确保程序能够正确处理各种异常情况,并防止恶意文件上传。
- 错误处理:
def save_uploaded_file():
try:
form = cgi.FieldStorage()
fileitem = form['file']
if fileitem.filename:
filepath = os.path.join('/path/to/upload/directory', secure_filename(fileitem.filename))
with open(filepath, 'wb') as f:
f.write(fileitem.file.read())
print("Content-type:text/html\r\n\r\n")
print(f"<html><body><h2>File '{fileitem.filename}' uploaded successfully!</h2></body></html>")
else:
raise ValueError("No file was uploaded")
except Exception as e:
print("Content-type:text/html\r\n\r\n")
print(f"<html><body><h2>Error: {e}</h2></body></html>")
save_uploaded_file()
- 防止恶意文件上传:
def is_safe_file(filename):
allowed_extensions = {'png', 'jpg', 'jpeg', 'gif'}
return '.' in filename and filename.rsplit('.', 1)[1].lower() in allowed_extensions
if not is_safe_file(fileitem.filename):
raise ValueError("Invalid file type")
通过以上步骤,您可以在Python CGI编程中有效地处理图片上传和操作。确保正确解析和存储上传的文件,使用Pillow库对图片进行处理,设置正确的MIME类型,处理错误和安全问题,可以帮助您构建一个健壮的图片处理系统。
相关问答FAQs:
如何在Python CGI中处理上传的图片?
在Python CGI编程中处理上传的图片通常涉及几个步骤。首先,确保你的HTML表单设置为enctype="multipart/form-data"
。当用户上传图片后,可以使用cgi.FieldStorage()
获取上传的文件。接下来,使用文件对象的file
属性来保存图片到服务器的指定目录,确保处理文件的命名和安全性,以防止文件覆盖或路径遍历攻击。
如何将处理后的图片显示在网页上?
在处理完图片后,可以通过生成HTML代码来显示图片。可以使用<img>
标签,并将图片的URL路径指向你保存图片的地址。在CGI脚本中,可以构建这样的HTML响应,确保浏览器能够正确找到并显示图片。
Python CGI编程中如何处理图片格式?
在处理图片时,了解支持的图片格式至关重要。Python的PIL
库(Pillow)可以帮助你处理和转换图片格式。通过使用Pillow,你可以打开图片文件,进行格式转换、调整大小、裁剪等操作。务必在处理前检查文件的MIME类型,以确保用户上传的是正确的图片格式,从而提高应用的安全性和稳定性。
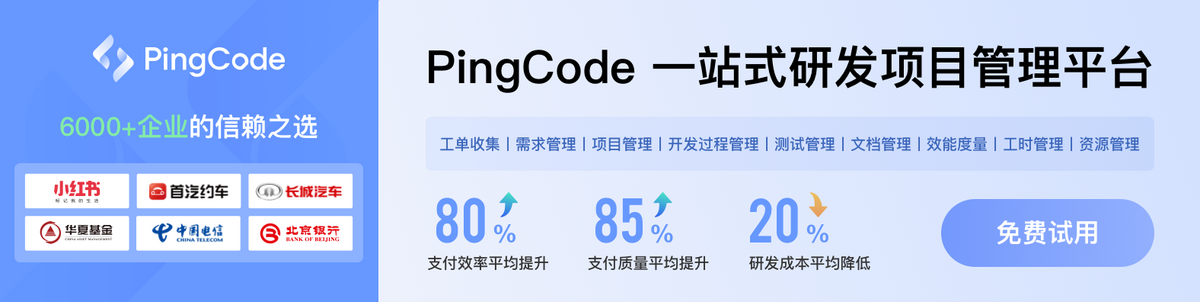