在Python中实现文件下发通知模板,您可以使用以下方法:利用模板引擎(如Jinja2)、自动生成邮件内容、发送邮件功能。 其中,模板引擎Jinja2是一个功能强大且易于使用的模板引擎,能够让你将变量和逻辑嵌入到模板中。通过这种方式,你可以轻松地生成个性化的通知邮件。下面我将详细描述如何使用Jinja2创建文件下发通知模板,并通过Python自动发送通知邮件。
一、模板引擎Jinja2
Jinja2是一个现代的、设计用于Python的模板引擎。它的设计灵活,易于扩展,可以与Python的其他库无缝集成。以下是使用Jinja2创建文件下发通知模板的步骤。
安装Jinja2
首先,你需要安装Jinja2库。你可以使用pip来安装:
pip install Jinja2
创建模板文件
创建一个HTML模板文件(如notification_template.html
),在这个模板文件中定义你的邮件内容,并使用占位符来插入动态数据。例如:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>File Dispatch Notification</title>
</head>
<body>
<p>Dear {{ recipient_name }},</p>
<p>This is to inform you that the file <strong>{{ file_name }}</strong> has been successfully dispatched to your designated location.</p>
<p>Details:</p>
<ul>
<li>File Name: {{ file_name }}</li>
<li>Dispatch Date: {{ dispatch_date }}</li>
<li>Location: {{ location }}</li>
</ul>
<p>Thank you,</p>
<p>Your Company</p>
</body>
</html>
渲染模板
使用Jinja2渲染模板,插入动态数据。以下是示例代码:
from jinja2 import Environment, FileSystemLoader
import datetime
Create a Jinja2 environment and load the template file
env = Environment(loader=FileSystemLoader('.'))
template = env.get_template('notification_template.html')
Data to insert into the template
data = {
'recipient_name': 'John Doe',
'file_name': 'report.pdf',
'dispatch_date': datetime.datetime.now().strftime('%Y-%m-%d'),
'location': 'Dropbox/Reports'
}
Render the template with the data
email_content = template.render(data)
print(email_content)
二、自动生成邮件内容
一旦你有了模板文件,并知道如何使用Jinja2渲染模板,你可以编写一个函数来自动生成邮件内容。这个函数可以接受必要的参数,并返回渲染后的模板内容。
def generate_email_content(recipient_name, file_name, dispatch_date, location):
from jinja2 import Environment, FileSystemLoader
env = Environment(loader=FileSystemLoader('.'))
template = env.get_template('notification_template.html')
data = {
'recipient_name': recipient_name,
'file_name': file_name,
'dispatch_date': dispatch_date,
'location': location
}
return template.render(data)
Example usage
email_content = generate_email_content(
'Jane Smith',
'financial_report.xlsx',
datetime.datetime.now().strftime('%Y-%m-%d'),
'Google Drive/Financial Reports'
)
print(email_content)
三、发送邮件功能
有了生成邮件内容的函数后,你还需要编写一个函数来发送邮件。你可以使用Python内置的smtplib
库来发送邮件。
配置邮件服务器
首先,你需要配置邮件服务器的连接信息,例如SMTP服务器地址、端口号、发件人邮箱和密码等。
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
SMTP_SERVER = 'smtp.example.com'
SMTP_PORT = 587
SMTP_USERNAME = 'your_email@example.com'
SMTP_PASSWORD = 'your_password'
发送邮件函数
接下来,编写一个函数来发送邮件。这个函数可以接受邮件内容、收件人地址等参数,并使用smtplib
发送邮件。
def send_email(subject, recipient, content):
msg = MIMEMultipart()
msg['From'] = SMTP_USERNAME
msg['To'] = recipient
msg['Subject'] = subject
msg.attach(MIMEText(content, 'html'))
with smtplib.SMTP(SMTP_SERVER, SMTP_PORT) as server:
server.starttls()
server.login(SMTP_USERNAME, SMTP_PASSWORD)
server.sendmail(SMTP_USERNAME, recipient, msg.as_string())
Example usage
subject = 'File Dispatch Notification'
recipient = 'recipient@example.com'
send_email(subject, recipient, email_content)
四、整合代码
将上述步骤整合到一个完整的脚本中,以便实现文件下发通知模板的自动生成和发送。
from jinja2 import Environment, FileSystemLoader
import datetime
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
SMTP configuration
SMTP_SERVER = 'smtp.example.com'
SMTP_PORT = 587
SMTP_USERNAME = 'your_email@example.com'
SMTP_PASSWORD = 'your_password'
def generate_email_content(recipient_name, file_name, dispatch_date, location):
env = Environment(loader=FileSystemLoader('.'))
template = env.get_template('notification_template.html')
data = {
'recipient_name': recipient_name,
'file_name': file_name,
'dispatch_date': dispatch_date,
'location': location
}
return template.render(data)
def send_email(subject, recipient, content):
msg = MIMEMultipart()
msg['From'] = SMTP_USERNAME
msg['To'] = recipient
msg['Subject'] = subject
msg.attach(MIMEText(content, 'html'))
with smtplib.SMTP(SMTP_SERVER, SMTP_PORT) as server:
server.starttls()
server.login(SMTP_USERNAME, SMTP_PASSWORD)
server.sendmail(SMTP_USERNAME, recipient, msg.as_string())
Generate email content
email_content = generate_email_content(
'Jane Smith',
'financial_report.xlsx',
datetime.datetime.now().strftime('%Y-%m-%d'),
'Google Drive/Financial Reports'
)
Send email
subject = 'File Dispatch Notification'
recipient = 'recipient@example.com'
send_email(subject, recipient, email_content)
通过以上步骤,你可以使用Python实现文件下发通知模板,并自动生成和发送通知邮件。模板引擎Jinja2提供了强大的模板处理能力,使得邮件内容的生成变得简单和高效。结合smtplib
库,你可以实现自动化的邮件发送功能,从而提高工作效率。
相关问答FAQs:
如何在Python中创建文件下发通知模板?
在Python中,可以使用字符串格式化和模板引擎(如Jinja2)来创建文件下发通知模板。首先,设计通知的基本结构,然后利用Python的文件操作功能,读取模板内容并替换占位符。这样可以确保通知内容灵活且易于维护。
使用Python发送文件下发通知的最佳实践是什么?
在发送文件下发通知时,建议使用邮件或消息队列系统(如RabbitMQ、Kafka等)。同时,确保文件大小适中并提供清晰的下载链接。如果使用邮件,设置合适的标题和内容格式,使接收者能够快速理解通知的重点。
如何处理文件下发通知中的错误或异常?
在实现文件下发通知时,务必添加错误处理机制。可以使用try-except块捕获文件读取、发送邮件等过程中的异常,并提供适当的反馈给用户,例如重试机制或错误日志记录,确保通知过程的顺利进行。
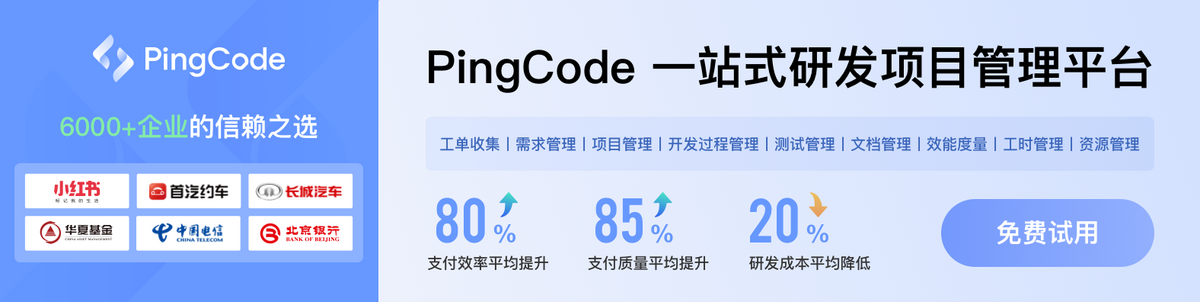