Python批量复制多个文件夹的方法:使用os模块、shutil模块、glob模块
在Python中,批量复制多个文件夹的方法有很多种,通常使用到的模块包括os模块、shutil模块、glob模块。你可以通过这些模块的组合使用,轻松实现批量复制文件夹的功能。以下将详细介绍这些方法,并提供相应的代码示例。
一、os模块
os
模块提供了与操作系统进行交互的功能,它可以用来遍历目录、创建目录等。利用os
模块,我们可以遍历目录并找到需要复制的文件夹。
示例代码:
import os
import shutil
def copy_folders(src, dest):
# 创建目标目录
if not os.path.exists(dest):
os.makedirs(dest)
for item in os.listdir(src):
s = os.path.join(src, item)
d = os.path.join(dest, item)
if os.path.isdir(s):
shutil.copytree(s, d, False, None)
else:
shutil.copy2(s, d)
source_directory = 'path_to_source_directory'
destination_directory = 'path_to_destination_directory'
copy_folders(source_directory, destination_directory)
在这个示例中,copy_folders
函数接受源目录和目标目录作为参数,遍历源目录中的所有文件和文件夹,并将它们复制到目标目录中。
二、shutil模块
shutil
模块提供了高级的文件和目录操作功能,比如复制、移动、重命名等。shutil.copytree
函数可以递归地复制整个目录树,非常适合用于批量复制文件夹。
示例代码:
import shutil
def copy_folders(src, dest):
shutil.copytree(src, dest)
source_directory = 'path_to_source_directory'
destination_directory = 'path_to_destination_directory'
copy_folders(source_directory, destination_directory)
在这个示例中,shutil.copytree
函数直接复制整个源目录到目标目录,非常简洁和高效。
三、glob模块
glob
模块提供了文件模式匹配功能,可以用来查找符合特定模式的文件和文件夹。结合os
和shutil
模块,可以实现批量复制特定模式的文件夹。
示例代码:
import glob
import shutil
import os
def copy_folders(pattern, dest):
# 创建目标目录
if not os.path.exists(dest):
os.makedirs(dest)
for folder in glob.glob(pattern):
if os.path.isdir(folder):
folder_name = os.path.basename(folder)
shutil.copytree(folder, os.path.join(dest, folder_name))
source_pattern = 'path_to_source_directory/*'
destination_directory = 'path_to_destination_directory'
copy_folders(source_pattern, destination_directory)
在这个示例中,glob.glob
函数根据指定的模式查找所有匹配的文件夹,并使用shutil.copytree
函数将它们复制到目标目录中。
四、综合应用
为了更好地理解如何批量复制多个文件夹,我们可以结合os
、shutil
和glob
模块,编写一个更通用的脚本,实现更灵活的文件夹复制功能。
示例代码:
import os
import shutil
import glob
def copy_folders(src, dest, pattern='*'):
# 创建目标目录
if not os.path.exists(dest):
os.makedirs(dest)
for folder in glob.glob(os.path.join(src, pattern)):
if os.path.isdir(folder):
folder_name = os.path.basename(folder)
shutil.copytree(folder, os.path.join(dest, folder_name))
source_directory = 'path_to_source_directory'
destination_directory = 'path_to_destination_directory'
copy_folders(source_directory, destination_directory)
在这个示例中,copy_folders
函数接受源目录、目标目录和文件夹模式作为参数,使用glob
模块查找所有匹配的文件夹,并使用shutil.copytree
函数将它们复制到目标目录中。
五、处理文件夹冲突
在批量复制文件夹的过程中,可能会遇到目标目录中已经存在同名文件夹的情况。为了解决这个问题,可以在复制之前检查目标目录中是否存在同名文件夹,如果存在,可以选择跳过、覆盖或重命名文件夹。
示例代码:
import os
import shutil
import glob
def copy_folders(src, dest, pattern='*', conflict_resolution='skip'):
# 创建目标目录
if not os.path.exists(dest):
os.makedirs(dest)
for folder in glob.glob(os.path.join(src, pattern)):
if os.path.isdir(folder):
folder_name = os.path.basename(folder)
dest_folder = os.path.join(dest, folder_name)
if os.path.exists(dest_folder):
if conflict_resolution == 'skip':
continue
elif conflict_resolution == 'overwrite':
shutil.rmtree(dest_folder)
elif conflict_resolution == 'rename':
counter = 1
while os.path.exists(dest_folder):
dest_folder = os.path.join(dest, f"{folder_name}_{counter}")
counter += 1
shutil.copytree(folder, dest_folder)
source_directory = 'path_to_source_directory'
destination_directory = 'path_to_destination_directory'
copy_folders(source_directory, destination_directory, conflict_resolution='rename')
在这个示例中,copy_folders
函数增加了一个conflict_resolution
参数,用于指定文件夹冲突时的处理方式。可以选择跳过(skip)、覆盖(overwrite)或重命名(rename)文件夹。
六、日志记录和错误处理
在批量复制文件夹的过程中,记录日志和处理错误是非常重要的。可以使用logging
模块记录操作日志,并在出现错误时进行适当的处理。
示例代码:
import os
import shutil
import glob
import logging
def setup_logging():
logging.basicConfig(filename='copy_folders.log', level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s')
def copy_folders(src, dest, pattern='*', conflict_resolution='skip'):
setup_logging()
logging.info(f"Starting to copy folders from {src} to {dest}")
# 创建目标目录
if not os.path.exists(dest):
os.makedirs(dest)
for folder in glob.glob(os.path.join(src, pattern)):
if os.path.isdir(folder):
folder_name = os.path.basename(folder)
dest_folder = os.path.join(dest, folder_name)
if os.path.exists(dest_folder):
if conflict_resolution == 'skip':
logging.warning(f"Skipping folder {folder_name} because it already exists")
continue
elif conflict_resolution == 'overwrite':
shutil.rmtree(dest_folder)
logging.info(f"Overwriting folder {folder_name}")
elif conflict_resolution == 'rename':
counter = 1
while os.path.exists(dest_folder):
dest_folder = os.path.join(dest, f"{folder_name}_{counter}")
counter += 1
logging.info(f"Renaming folder {folder_name} to {folder_name}_{counter-1}")
try:
shutil.copytree(folder, dest_folder)
logging.info(f"Copied folder {folder_name} to {dest_folder}")
except Exception as e:
logging.error(f"Failed to copy folder {folder_name}: {e}")
source_directory = 'path_to_source_directory'
destination_directory = 'path_to_destination_directory'
copy_folders(source_directory, destination_directory, conflict_resolution='rename')
在这个示例中,使用logging
模块记录每个文件夹复制操作的详细信息,包括开始复制、跳过文件夹、覆盖文件夹、重命名文件夹和复制失败等情况。
七、总结
通过以上几种方法和代码示例,我们详细介绍了如何使用Python批量复制多个文件夹。主要使用了os
、shutil
和glob
模块,并结合实际需求进行了优化,比如处理文件夹冲突、记录日志和处理错误等。希望这些内容能帮助你更好地理解和掌握Python批量复制文件夹的方法。
相关问答FAQs:
如何在Python中实现批量复制文件夹的功能?
在Python中,您可以使用shutil
模块轻松实现批量复制文件夹。shutil.copytree()
函数能够复制整个文件夹及其内容。只需提供源文件夹路径和目标文件夹路径即可。同时,您可以结合os
模块遍历多个文件夹并逐一复制。以下是一个简单的示例代码:
import os
import shutil
source_dirs = ['folder1', 'folder2', 'folder3'] # 源文件夹列表
destination = 'backup/' # 目标路径
for folder in source_dirs:
shutil.copytree(folder, os.path.join(destination, folder))
在复制文件夹时,如何处理文件夹内的文件冲突?
当您复制的文件夹中存在同名文件时,shutil.copytree()
会抛出错误。为了解决这个问题,可以在复制之前检查目标文件夹是否已存在,或者使用dirs_exist_ok=True
参数来允许覆盖现有文件夹。示例如下:
shutil.copytree(source_folder, destination_folder, dirs_exist_ok=True)
这样,当目标文件夹已存在时,文件夹内容将被合并,而不是产生错误。
有没有推荐的第三方库来进行文件夹的批量复制?
除了Python内置的shutil
模块,您还可以考虑使用第三方库如pathlib
和filecmp
,它们提供了更为灵活的文件操作功能。pathlib
可以方便地处理路径对象,而filecmp
则有助于比较文件夹内容,确保复制后的文件夹与源文件夹一致。这些库可以为您的文件管理提供更多的便利性和功能。
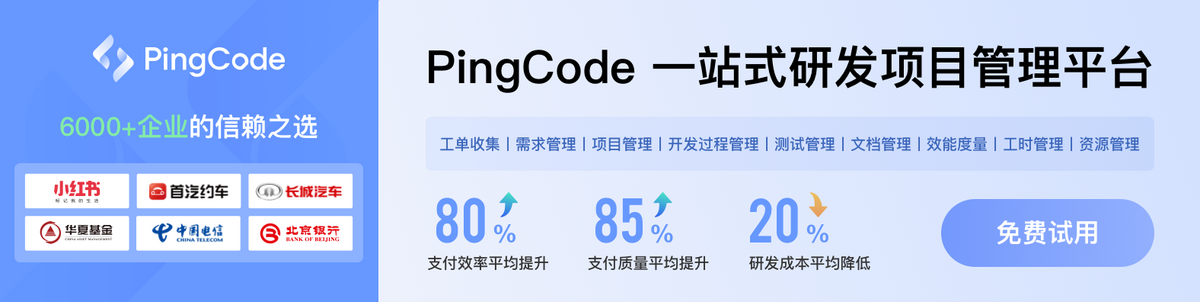