在Python中提取第一行的方法有多种,包括使用文件操作、Pandas库和其他方法。以下是一些常见的方法:使用文件操作、使用Pandas库、使用Numpy库。下面我们将详细探讨其中的一种方法。
使用文件操作:在Python中,可以使用内置的open()
函数来读取文件,并通过调用readline()
方法来获取文件的第一行。这种方法适用于处理较小的文件,因为它一次性将整个文件读取到内存中。如果文件非常大,建议使用其他更高效的方法。以下是一个简单的示例代码:
# 使用文件操作来读取文件的第一行
with open('example.txt', 'r') as file:
first_line = file.readline().strip()
print(first_line)
在上面的代码中,我们使用with open('example.txt', 'r')
来打开文件,其中'r'
表示以只读模式打开文件。file.readline()
方法读取文件的第一行,并使用strip()
方法去除行尾的换行符和空白字符。最后,通过print()
函数输出第一行内容。
一、使用文件操作
1.1 使用open()函数和readline()方法
Python内置的open()
函数和readline()
方法可以非常方便地读取文件的第一行。以下是具体的操作步骤:
def read_first_line(file_path):
try:
with open(file_path, 'r') as file:
first_line = file.readline().strip()
return first_line
except FileNotFoundError:
return "File not found."
except Exception as e:
return str(e)
示例
file_path = 'example.txt'
print(read_first_line(file_path))
在这个示例中,file_path
是文件的路径。open(file_path, 'r')
以只读模式打开文件,readline().strip()
方法读取第一行并去除多余的空白字符。错误处理部分使用了try-except
块,以防止文件不存在或其他异常情况。
1.2 使用readlines()方法
readlines()
方法可以一次性读取文件中的所有行,并返回一个列表。通过访问列表的第一个元素,可以获得文件的第一行。以下是一个示例:
def read_first_line(file_path):
try:
with open(file_path, 'r') as file:
lines = file.readlines()
if lines:
first_line = lines[0].strip()
return first_line
else:
return "File is empty."
except FileNotFoundError:
return "File not found."
except Exception as e:
return str(e)
示例
file_path = 'example.txt'
print(read_first_line(file_path))
此方法适用于需要对文件进行多行操作的情况,但对于大型文件,由于一次性读取所有行,可能会占用大量内存。
二、使用Pandas库
2.1 读取CSV文件的第一行
Pandas是一个强大的数据分析库,特别适合处理结构化数据,如CSV文件。以下是使用Pandas读取CSV文件第一行的示例:
import pandas as pd
def read_first_line_csv(file_path):
try:
df = pd.read_csv(file_path, nrows=1)
first_line = df.iloc[0].to_string(index=False)
return first_line
except FileNotFoundError:
return "File not found."
except Exception as e:
return str(e)
示例
file_path = 'example.csv'
print(read_first_line_csv(file_path))
在这个示例中,pd.read_csv(file_path, nrows=1)
读取CSV文件的第一行,并将其存储在DataFrame对象中。df.iloc[0].to_string(index=False)
方法将第一行转换为字符串格式,并去除索引。
2.2 读取Excel文件的第一行
Pandas还支持读取Excel文件。以下是读取Excel文件第一行的示例:
import pandas as pd
def read_first_line_excel(file_path):
try:
df = pd.read_excel(file_path, nrows=1)
first_line = df.iloc[0].to_string(index=False)
return first_line
except FileNotFoundError:
return "File not found."
except Exception as e:
return str(e)
示例
file_path = 'example.xlsx'
print(read_first_line_excel(file_path))
与读取CSV文件类似,我们使用pd.read_excel(file_path, nrows=1)
读取Excel文件的第一行,并将其转换为字符串格式。
三、使用Numpy库
Numpy是一个用于科学计算的库,尽管它主要用于处理数值数据,但也可以用于读取文件的第一行。以下是一个示例:
import numpy as np
def read_first_line_numpy(file_path):
try:
data = np.genfromtxt(file_path, delimiter=',', dtype=str, max_rows=1)
if data.size > 0:
first_line = ','.join(data)
return first_line
else:
return "File is empty."
except FileNotFoundError:
return "File not found."
except Exception as e:
return str(e)
示例
file_path = 'example.csv'
print(read_first_line_numpy(file_path))
在这个示例中,np.genfromtxt(file_path, delimiter=',', dtype=str, max_rows=1)
函数从文件中读取第一行,并将其存储为Numpy数组。','.join(data)
方法将数组转换为逗号分隔的字符串。
四、使用Pathlib库
Python的pathlib
库提供了一种面向对象的方式来处理文件和目录路径。以下是使用pathlib
库读取文件第一行的示例:
from pathlib import Path
def read_first_line_pathlib(file_path):
try:
path = Path(file_path)
if path.is_file():
first_line = path.read_text().splitlines()[0]
return first_line
else:
return "File not found."
except IndexError:
return "File is empty."
except Exception as e:
return str(e)
示例
file_path = 'example.txt'
print(read_first_line_pathlib(file_path))
在这个示例中,我们使用Path(file_path)
创建一个Path对象,path.read_text().splitlines()[0]
方法读取文件内容并获取第一行。
五、使用csv模块
Python内置的csv
模块也可以用于读取CSV文件的第一行。以下是一个示例:
import csv
def read_first_line_csv_module(file_path):
try:
with open(file_path, 'r') as file:
reader = csv.reader(file)
first_line = next(reader)
return ','.join(first_line)
except FileNotFoundError:
return "File not found."
except StopIteration:
return "File is empty."
except Exception as e:
return str(e)
示例
file_path = 'example.csv'
print(read_first_line_csv_module(file_path))
在这个示例中,我们使用csv.reader(file)
创建一个CSV读取器对象,next(reader)
方法读取CSV文件的第一行。
六、使用gzip模块处理压缩文件
如果文件是gzip压缩格式,我们可以使用Python内置的gzip
模块来读取文件的第一行。以下是一个示例:
import gzip
def read_first_line_gzip(file_path):
try:
with gzip.open(file_path, 'rt') as file:
first_line = file.readline().strip()
return first_line
except FileNotFoundError:
return "File not found."
except Exception as e:
return str(e)
示例
file_path = 'example.txt.gz'
print(read_first_line_gzip(file_path))
在这个示例中,我们使用gzip.open(file_path, 'rt')
以文本模式打开压缩文件,并使用readline().strip()
方法读取第一行。
七、使用itertools模块
itertools
模块提供了高效的迭代工具,可以用于读取文件的第一行。以下是一个示例:
import itertools
def read_first_line_itertools(file_path):
try:
with open(file_path, 'r') as file:
first_line = next(itertools.islice(file, 1)).strip()
return first_line
except FileNotFoundError:
return "File not found."
except StopIteration:
return "File is empty."
except Exception as e:
return str(e)
示例
file_path = 'example.txt'
print(read_first_line_itertools(file_path))
在这个示例中,我们使用itertools.islice(file, 1)
迭代文件,并使用next()
方法读取第一行。
八、比较不同方法的优缺点
8.1 文件操作
优点:
- 简单易用,适用于各种文件类型。
- 内置函数,无需额外安装库。
缺点:
- 对于大文件,可能会占用大量内存。
- 需要手动处理异常情况。
8.2 Pandas库
优点:
- 适用于结构化数据,如CSV和Excel文件。
- 功能强大,支持各种数据操作。
缺点:
- 需要安装额外的库。
- 对于简单的文件读取操作,可能显得过于复杂。
8.3 Numpy库
优点:
- 适用于数值数据处理。
- 性能优越,适合大数据处理。
缺点:
- 需要安装额外的库。
- 对于非数值数据,使用不够便捷。
8.4 Pathlib库
优点:
- 面向对象的路径处理方式,代码更加简洁。
- 内置函数,无需额外安装库。
缺点:
- 对于复杂的数据处理,功能有限。
8.5 csv模块
优点:
- 内置模块,适用于CSV文件。
- 简单易用,性能较好。
缺点:
- 仅适用于CSV文件,功能有限。
8.6 gzip模块
优点:
- 适用于处理gzip压缩文件。
- 内置模块,无需额外安装库。
缺点:
- 仅适用于gzip格式,功能有限。
8.7 itertools模块
优点:
- 高效迭代工具,性能优越。
- 内置模块,无需额外安装库。
缺点:
- 代码相对复杂,不适合初学者。
总结以上方法,每种方法都有其优缺点,选择合适的方法取决于具体的需求和文件格式。对于简单的文件读取操作,文件操作和pathlib
库是不错的选择;对于结构化数据,Pandas库更为适合;对于大文件和数值数据处理,Numpy库表现优越;对于特殊格式的文件,如gzip压缩文件,可以使用gzip
模块。希望这些方法能够帮助你在Python中轻松提取文件的第一行。
相关问答FAQs:
如何在Python中读取文件的第一行?
在Python中,可以使用内置的文件操作功能来读取文件的第一行。打开文件后,使用readline()
方法可以直接提取第一行。例如:
with open('文件名.txt', 'r') as file:
first_line = file.readline()
print(first_line)
此方法简单有效,适合大多数文本文件。
提取列表或字符串的第一行应该使用什么方法?
对于字符串或列表,如果希望提取第一项,可以直接通过索引实现。对于字符串,可以使用splitlines()
方法将其分割为多行,然后选择第一行。例如:
text = """这是第一行
这是第二行"""
first_line = text.splitlines()[0]
print(first_line)
对于列表,只需使用list[0]
来获得第一项。
在读取CSV文件时,如何提取第一行的数据?
处理CSV文件时,可以使用csv
模块来提取第一行。以下代码示例展示了如何实现:
import csv
with open('文件名.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
first_row = next(reader) # 读取第一行
print(first_row)
此方法不仅能够提取第一行,还可轻松处理CSV格式的数据。
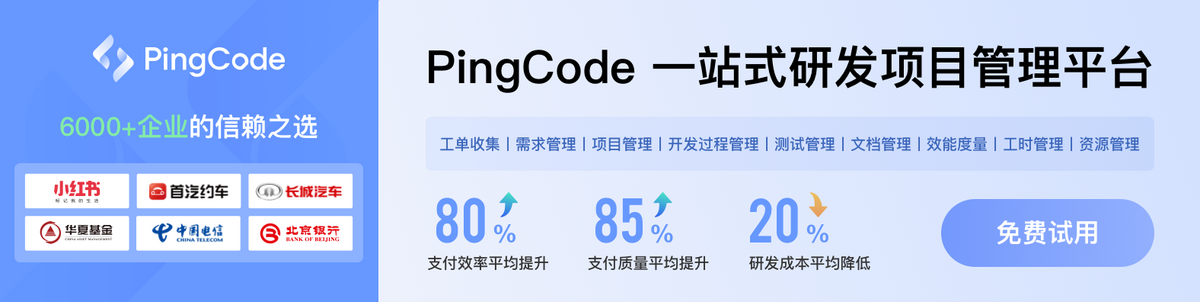