在Python程序源码中实现回退功能可以通过多种方法来完成,使用栈数据结构、保存状态快照、利用撤销重做模式。其中,使用栈数据结构是最常用的方式之一,因为它可以方便地存储和恢复状态。栈的后进先出(LIFO)特性非常适合实现回退功能。下面将详细描述如何使用栈数据结构来实现回退功能。
一、使用栈数据结构实现回退功能
栈是一种后进先出(LIFO)的数据结构,可以用来存储状态的历史记录。每次执行操作时,将当前状态压入栈中;当需要回退时,从栈中弹出上一个状态并恢复。
1.1、定义栈数据结构
在Python中,可以使用列表来实现栈。定义一个类来封装栈的操作:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
return None
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
1.2、保存和恢复状态
定义一个类来表示程序的状态,并在执行操作时保存状态到栈中:
class ProgramState:
def __init__(self, data):
self.data = data
def __str__(self):
return str(self.data)
class Program:
def __init__(self):
self.state_stack = Stack()
self.current_state = ProgramState(data={})
def save_state(self):
self.state_stack.push(self.current_state)
def restore_state(self):
previous_state = self.state_stack.pop()
if previous_state:
self.current_state = previous_state
def execute_operation(self, operation):
self.save_state()
# Simulate an operation that changes the state
self.current_state.data = operation(self.current_state.data)
def display_state(self):
print(self.current_state)
1.3、示例操作
示例操作函数可以是任何修改状态的操作:
def add_key_value(data):
data['key'] = 'value'
return data
def remove_key(data):
if 'key' in data:
del data['key']
return data
def main():
program = Program()
program.execute_operation(add_key_value)
program.display_state() # Output: {'key': 'value'}
program.execute_operation(remove_key)
program.display_state() # Output: {}
program.restore_state()
program.display_state() # Output: {'key': 'value'}
program.restore_state()
program.display_state() # Output: {}
program.restore_state()
program.display_state() # Output: {}
if __name__ == "__main__":
main()
二、保存状态快照
另一种实现回退功能的方法是保存程序的状态快照。每次执行操作时,保存当前状态的副本,当需要回退时,恢复保存的状态。
2.1、定义状态快照类
import copy
class StateSnapshot:
def __init__(self, state):
self.state = copy.deepcopy(state)
2.2、修改程序类以保存和恢复快照
class Program:
def __init__(self):
self.state_stack = Stack()
self.current_state = ProgramState(data={})
def save_snapshot(self):
snapshot = StateSnapshot(self.current_state)
self.state_stack.push(snapshot)
def restore_snapshot(self):
snapshot = self.state_stack.pop()
if snapshot:
self.current_state = snapshot.state
def execute_operation(self, operation):
self.save_snapshot()
self.current_state.data = operation(self.current_state.data)
def display_state(self):
print(self.current_state)
三、利用撤销重做模式
撤销重做模式是一种常见的设计模式,用于实现撤销和重做功能。
3.1、定义命令类
class Command:
def execute(self):
pass
def undo(self):
pass
class AddKeyValueCommand(Command):
def __init__(self, program, key, value):
self.program = program
self.key = key
self.value = value
def execute(self):
self.program.current_state.data[self.key] = self.value
def undo(self):
del self.program.current_state.data[self.key]
3.2、修改程序类以支持命令模式
class Program:
def __init__(self):
self.state_stack = Stack()
self.command_stack = Stack()
self.current_state = ProgramState(data={})
def execute_command(self, command):
self.command_stack.push(command)
command.execute()
def undo_command(self):
command = self.command_stack.pop()
if command:
command.undo()
def display_state(self):
print(self.current_state)
3.3、示例操作
def main():
program = Program()
add_command = AddKeyValueCommand(program, 'key', 'value')
program.execute_command(add_command)
program.display_state() # Output: {'key': 'value'}
program.undo_command()
program.display_state() # Output: {}
program.undo_command() # No effect
program.display_state() # Output: {}
if __name__ == "__main__":
main()
总结
在Python程序源码中实现回退功能可以通过多种方法实现,使用栈数据结构、保存状态快照、利用撤销重做模式。这些方法各有优缺点,选择适合自己程序需求的方法非常重要。栈数据结构适用于简单的回退功能,状态快照适用于复杂的状态保存,而撤销重做模式适用于需要复杂操作管理的程序。通过合理使用这些技术,可以有效地实现程序的回退功能,提高程序的易用性和可靠性。
相关问答FAQs:
如何在Python程序中实现撤销操作?
在Python程序中实现撤销操作通常涉及到使用数据结构来存储历史状态。常见的方法是使用栈(stack)来记录每一步的状态变化。当需要撤销时,可以从栈中弹出最近的状态,并将其应用到当前状态。这种方法适用于文本编辑器、绘图程序等需要记录用户操作的场景。
有什么常用的库可以帮助实现回退功能?
在Python中,有一些库可以帮助实现回退功能。例如,pickle
库可以用来序列化和反序列化对象,从而保存对象的状态。此外,command pattern
设计模式也可以被应用于实现撤销和重做的功能,通过将每个操作封装成对象来处理状态的保存和恢复。
如何处理复杂数据结构的回退功能?
对于复杂数据结构,如树或图,回退功能的实现可能需要更复杂的状态管理。可以选择在每次操作后保存完整的结构快照,或者使用变更日志来记录每个操作的细节。使用这些信息,可以在需要时恢复到之前的状态,确保程序的灵活性和可靠性。
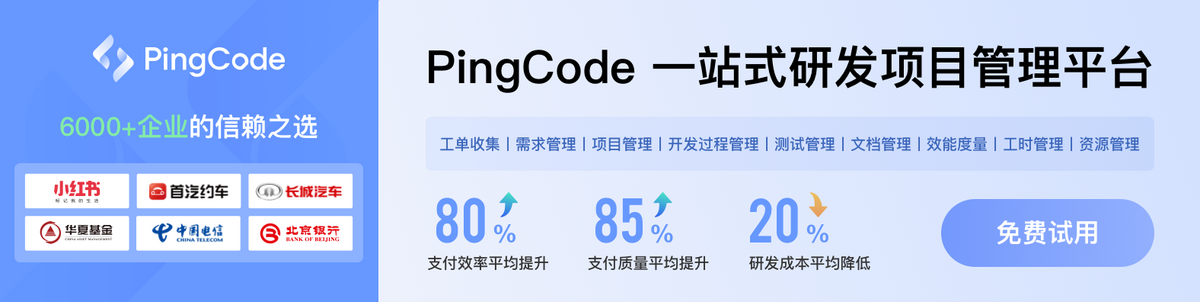