如何判断程序是否还在运行python
判断程序是否还在运行可以通过轮询状态检查、使用进程管理工具、查看日志输出、通过网络连接监控等方式来实现。使用进程管理工具是其中一种常见且有效的方法。通过操作系统提供的进程管理工具,如ps
命令或tasklist
命令,用户可以查询指定的进程是否还在运行。
使用进程管理工具
通过进程管理工具检测程序是否在运行是一种高效的方法。这种工具可以列出系统中所有的进程,并且可以根据进程名称或ID过滤出特定的进程。以下是使用Python脚本结合操作系统命令来判断程序是否在运行的详细步骤。
import subprocess
def is_program_running(program_name):
try:
# 使用 ps 命令检查进程
output = subprocess.check_output(['ps', 'aux']).decode()
return program_name in output
except Exception as e:
print(f"An error occurred: {e}")
return False
示例使用
if is_program_running('python'):
print("Python 程序正在运行")
else:
print("Python 程序未在运行")
一、轮询状态检查
轮询状态检查是一种简单的方法,通过定期检查某些状态指标来判断程序是否在运行。它适用于较小规模的程序和进程,但对于大型系统可能会带来性能开销。
1.1 使用文件锁
文件锁是一种常见的轮询状态检查方法。程序在运行时创建一个锁文件,当程序退出时删除该文件。通过检查锁文件是否存在,可以判断程序是否在运行。
import os
lock_file = '/tmp/my_program.lock'
def is_running():
return os.path.exists(lock_file)
创建锁文件表示程序正在运行
with open(lock_file, 'w') as f:
f.write('')
检查程序是否在运行
if is_running():
print("程序正在运行")
else:
print("程序未在运行")
程序退出时删除锁文件
os.remove(lock_file)
1.2 使用进程ID文件
进程ID文件(PID文件)是一种更精确的轮询状态检查方法。程序在启动时将其进程ID写入PID文件,退出时删除该文件。通过读取PID文件中的进程ID并检查该进程是否存在,可以更准确地判断程序是否在运行。
import os
import signal
pid_file = '/tmp/my_program.pid'
def is_running():
if os.path.exists(pid_file):
with open(pid_file, 'r') as f:
pid = int(f.read())
try:
os.kill(pid, 0)
return True
except ProcessLookupError:
return False
return False
创建PID文件表示程序正在运行
with open(pid_file, 'w') as f:
f.write(str(os.getpid()))
检查程序是否在运行
if is_running():
print("程序正在运行")
else:
print("程序未在运行")
程序退出时删除PID文件
os.remove(pid_file)
二、使用进程管理工具
进程管理工具是操作系统提供的用于管理和监控进程的工具。这些工具通常可以列出系统中所有的进程,并提供详细的进程信息。通过使用这些工具,可以很方便地判断某个程序是否在运行。
2.1 使用ps命令
ps
命令是类Unix系统中常用的进程管理工具。它可以列出当前系统中的所有进程,并提供详细的进程信息。通过结合使用grep
命令,可以很方便地判断某个程序是否在运行。
import subprocess
def is_program_running(program_name):
try:
output = subprocess.check_output(['ps', 'aux']).decode()
return program_name in output
except Exception as e:
print(f"An error occurred: {e}")
return False
示例使用
if is_program_running('python'):
print("Python 程序正在运行")
else:
print("Python 程序未在运行")
2.2 使用tasklist命令
tasklist
命令是Windows系统中的进程管理工具。它可以列出当前系统中的所有进程,并提供详细的进程信息。通过结合使用findstr
命令,可以很方便地判断某个程序是否在运行。
import subprocess
def is_program_running(program_name):
try:
output = subprocess.check_output(['tasklist']).decode()
return program_name in output
except Exception as e:
print(f"An error occurred: {e}")
return False
示例使用
if is_program_running('python.exe'):
print("Python 程序正在运行")
else:
print("Python 程序未在运行")
三、查看日志输出
查看日志输出是一种间接的方法,通过分析程序的日志文件来判断程序是否在运行。这种方法适用于有日志记录功能的程序。
3.1 实时日志分析
通过实时分析程序的日志文件,可以判断程序是否在运行。例如,可以使用tail
命令实时查看日志文件的最新内容,如果日志文件中有持续的输出,则说明程序正在运行。
import subprocess
log_file = '/var/log/my_program.log'
def is_program_running():
try:
output = subprocess.check_output(['tail', '-n', '1', log_file]).decode()
return bool(output.strip())
except Exception as e:
print(f"An error occurred: {e}")
return False
示例使用
if is_program_running():
print("程序正在运行")
else:
print("程序未在运行")
3.2 日志文件更新检查
通过检查日志文件的更新时间,可以判断程序是否在运行。如果日志文件在一段时间内没有更新,则可能说明程序已经停止运行。
import os
import time
log_file = '/var/log/my_program.log'
def is_program_running():
try:
last_modified_time = os.path.getmtime(log_file)
current_time = time.time()
return (current_time - last_modified_time) < 60 # 检查日志文件是否在1分钟内更新
except Exception as e:
print(f"An error occurred: {e}")
return False
示例使用
if is_program_running():
print("程序正在运行")
else:
print("程序未在运行")
四、通过网络连接监控
对于网络服务程序,可以通过监控其网络连接来判断程序是否在运行。这种方法适用于运行在特定端口上的网络服务程序。
4.1 使用netstat命令
netstat
命令是网络状态查看工具。通过使用netstat
命令,可以查看系统中所有的网络连接,并判断特定端口是否在监听。
import subprocess
def is_port_listening(port):
try:
output = subprocess.check_output(['netstat', '-an']).decode()
return f":{port} " in output
except Exception as e:
print(f"An error occurred: {e}")
return False
示例使用
if is_port_listening(8080):
print("端口 8080 正在监听")
else:
print("端口 8080 未在监听")
4.2 使用socket库
socket
库是Python标准库中的网络通信模块。通过尝试连接特定端口,可以判断该端口是否在监听,从而判断程序是否在运行。
import socket
def is_port_listening(host, port):
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.settimeout(1)
try:
s.connect((host, port))
s.close()
return True
except Exception as e:
return False
示例使用
if is_port_listening('127.0.0.1', 8080):
print("端口 8080 正在监听")
else:
print("端口 8080 未在监听")
总结
判断程序是否还在运行有多种方法可供选择,每种方法都有其适用的场景和优缺点。使用进程管理工具是其中一种常见且有效的方法,适用于大多数操作系统和程序。此外,还可以通过轮询状态检查、查看日志输出以及通过网络连接监控等方法来判断程序是否在运行。根据实际需求和环境,选择最合适的方法来实现程序运行状态的监控。
相关问答FAQs:
如何检测Python程序是否在后台运行?
可以使用操作系统的任务管理器或命令行工具来检查Python程序是否在运行。在Windows上,可以打开任务管理器,查看“进程”标签,寻找“python.exe”或相关名称。在Linux或macOS上,可以使用ps aux | grep python
命令来筛选出正在运行的Python进程。
在Python中如何监控程序的运行状态?
可以利用Python内置的os
和signal
模块来检查程序的运行状态。例如,可以通过获取当前进程的PID并使用os.kill(pid, 0)
来判断该进程是否存在。如果该进程仍在运行,则不会抛出异常。
如何在Python脚本中实现自我监测?
可以在程序中设置一个定时器,定期检查某些条件,比如CPU占用率或内存使用情况,以确定程序是否正常运行。使用psutil
库可以方便地获取系统和进程信息,从而实现对程序状态的监控和记录。
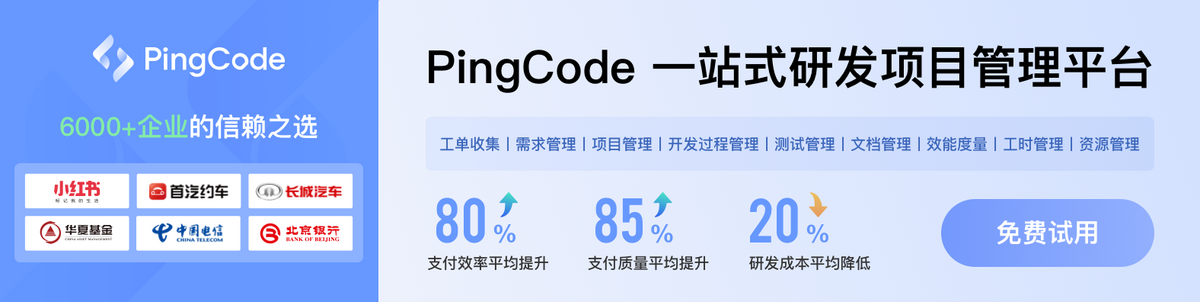