在Python中使用百度地图API,需要完成以下几个步骤:获取API密钥、安装相关库、编写代码进行接口调用、处理返回数据。接下来,我们将详细介绍如何使用百度地图API在Python中进行地图和位置服务的开发。
一、获取API密钥
要使用百度地图API,首先需要在百度开发者平台申请一个API密钥。访问百度开发者平台(http://lbsyun.baidu.com/),注册并登录后,创建一个应用并获取API密钥。
二、安装相关库
为了方便与百度地图API进行交互,我们需要安装一些Python库,例如requests库,用于发送HTTP请求。可以使用pip安装:
pip install requests
三、编写代码进行接口调用
接下来,我们将使用百度地图API的一些功能,例如地理编码、逆地理编码、路线规划等。
地理编码(地址转坐标)
地理编码是将地址转换为地理坐标(经纬度)的过程。下面是一个示例代码:
import requests
def geocode_address(address, api_key):
base_url = "http://api.map.baidu.com/geocoding/v3/"
params = {
"address": address,
"output": "json",
"ak": api_key
}
response = requests.get(base_url, params=params)
if response.status_code == 200:
result = response.json()
if result['status'] == 0:
location = result['result']['location']
return location
else:
return result['msg']
else:
return "Error: Unable to fetch data"
api_key = "YOUR_API_KEY"
address = "北京市海淀区上地十街10号"
location = geocode_address(address, api_key)
print(location)
逆地理编码(坐标转地址)
逆地理编码是将地理坐标转换为地址的过程。下面是一个示例代码:
def reverse_geocode(lat, lng, api_key):
base_url = "http://api.map.baidu.com/reverse_geocoding/v3/"
params = {
"location": f"{lat},{lng}",
"output": "json",
"ak": api_key
}
response = requests.get(base_url, params=params)
if response.status_code == 200:
result = response.json()
if result['status'] == 0:
address = result['result']['formatted_address']
return address
else:
return result['msg']
else:
return "Error: Unable to fetch data"
lat, lng = 39.983424, 116.322987
address = reverse_geocode(lat, lng, api_key)
print(address)
路线规划
百度地图API还支持路线规划功能,可以根据起点和终点进行驾车、步行、骑行路线规划。下面是驾车路线规划的示例代码:
def driving_route(origin, destination, api_key):
base_url = "http://api.map.baidu.com/directionlite/v1/driving"
params = {
"origin": origin,
"destination": destination,
"ak": api_key
}
response = requests.get(base_url, params=params)
if response.status_code == 200:
result = response.json()
if result['status'] == 0:
routes = result['result']['routes']
return routes
else:
return result['msg']
else:
return "Error: Unable to fetch data"
origin = "39.915,116.404"
destination = "39.975,116.329"
routes = driving_route(origin, destination, api_key)
print(routes)
四、处理返回数据
API返回的数据通常是JSON格式,需要进行解析处理。上面的示例代码中已经展示了如何解析返回的JSON数据并提取所需的信息。
处理地理编码数据
地理编码返回的数据包括经纬度坐标,我们可以将其打印或者存储在数据库中:
location = geocode_address(address, api_key)
if isinstance(location, dict):
print(f"Latitude: {location['lat']}, Longitude: {location['lng']}")
else:
print(location)
处理逆地理编码数据
逆地理编码返回的数据包括格式化地址信息,我们可以将其打印或者使用在其他业务逻辑中:
address = reverse_geocode(lat, lng, api_key)
print(f"Address: {address}")
处理路线规划数据
路线规划返回的数据包括路线的详细信息,例如距离、时间、途径点等。我们可以解析这些信息并进行展示:
routes = driving_route(origin, destination, api_key)
if isinstance(routes, list):
for route in routes:
print(f"Distance: {route['distance']} meters, Duration: {route['duration']} seconds")
for step in route['steps']:
print(f"Step: {step['instruction']}")
else:
print(routes)
五、综合应用
通过上述步骤,我们已经掌握了如何在Python中使用百度地图API。我们可以将这些功能集成到一个综合应用中,例如一个简单的地图查询工具:
def main():
api_key = "YOUR_API_KEY"
while True:
print("1. Geocode Address")
print("2. Reverse Geocode")
print("3. Driving Route")
print("4. Exit")
choice = input("Enter your choice: ")
if choice == '1':
address = input("Enter address: ")
location = geocode_address(address, api_key)
if isinstance(location, dict):
print(f"Latitude: {location['lat']}, Longitude: {location['lng']}")
else:
print(location)
elif choice == '2':
lat = input("Enter latitude: ")
lng = input("Enter longitude: ")
address = reverse_geocode(lat, lng, api_key)
print(f"Address: {address}")
elif choice == '3':
origin = input("Enter origin (lat,lng): ")
destination = input("Enter destination (lat,lng): ")
routes = driving_route(origin, destination, api_key)
if isinstance(routes, list):
for route in routes:
print(f"Distance: {route['distance']} meters, Duration: {route['duration']} seconds")
for step in route['steps']:
print(f"Step: {step['instruction']}")
else:
print(routes)
elif choice == '4':
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
通过以上步骤,我们实现了一个简单的Python程序,能够使用百度地图API进行地理编码、逆地理编码和路线规划等操作。这只是百度地图API功能的一小部分,开发者可以根据具体需求探索更多的API功能,例如地点搜索、周边检索、行政区划查询等,来实现更加复杂和丰富的地图应用。
相关问答FAQs:
如何在Python中获取百度地图API的密钥?
要使用百度地图API,首先需要申请一个API密钥。您可以访问百度地图开放平台网站,注册账号后创建应用。在应用设置中,您将能够生成一个API密钥。请确保您选择的服务类型与您的需求相符,如地理编码、路径规划等。
如何在Python中发送请求并解析百度地图API的响应?
使用Python发送HTTP请求可以通过库如requests
来实现。您可以构建URL,包含API密钥和所需的参数,然后使用requests.get()
方法发送请求。收到的响应通常是JSON格式,您可以使用response.json()
方法将其解析为Python字典,以便提取所需的信息,例如经纬度或地址。
百度地图API支持哪些功能?
百度地图API提供多种功能,包括地理编码(地址转换为坐标)、逆地理编码(坐标转换为地址)、路径规划、地点搜索、周边检索等。每个功能都有对应的API接口,您可以根据具体需求选择合适的接口进行调用,帮助您实现地图相关的应用开发。
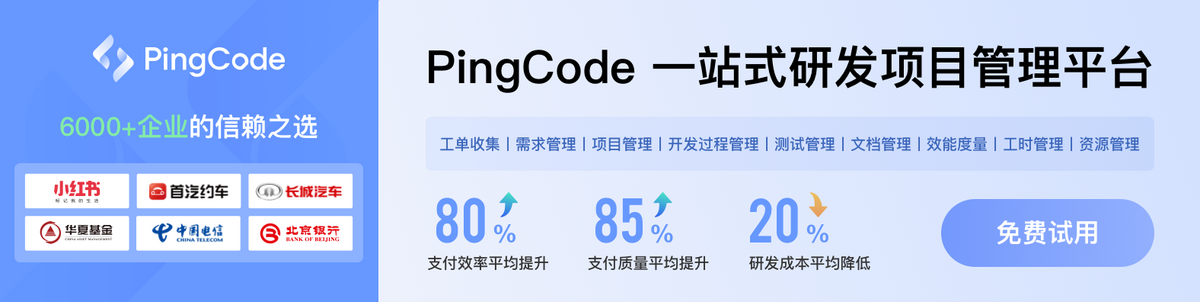