在手机上查看Python动图的方法包括使用Jupyter Notebook、使用Python库生成动图并保存为GIF或视频文件、利用在线平台、使用移动应用程序。这些方法使得在移动设备上查看和操作Python生成的动图变得简单方便。下面我将详细展开其中的一个方法:使用Jupyter Notebook。
使用Jupyter Notebook:
Jupyter Notebook 是一个非常流行的工具,广泛用于数据科学和机器学习。它允许用户在一个交互式环境中编写和运行代码,生成图表和动图,并即时查看结果。通过在手机上访问Jupyter Notebook,可以方便地查看Python生成的动图。
一、使用Jupyter Notebook
-
安装和设置 Jupyter Notebook:
首先,在你的电脑上安装Jupyter Notebook。你可以使用Anaconda进行安装,这是一款包含了许多数据科学工具的开源Python发行版。安装完成后,启动Jupyter Notebook。
conda install jupyter
jupyter notebook
-
编写生成动图的Python代码:
在Jupyter Notebook中编写生成动图的Python代码。例如,使用Matplotlib库来生成简单的动图:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
x = np.arange(0, 2*np.pi, 0.01)
line, = ax.plot(x, np.sin(x))
def update(num, x, line):
line.set_ydata(np.sin(x + num / 10.0))
return line,
ani = animation.FuncAnimation(fig, update, frames=100, fargs=[x, line], interval=50)
plt.show()
-
保存动图为GIF文件:
将生成的动图保存为GIF文件,以便在手机上查看。你可以使用ImageMagick或Pillow库来保存动图:
ani.save('sine_wave.gif', writer='imagemagick', fps=30)
-
将文件传输到手机:
使用USB连接或通过云存储(如Google Drive、Dropbox)将生成的GIF文件传输到手机。
-
在手机上查看动图:
在手机上打开GIF文件,你就可以查看Python生成的动图了。
二、使用Python库生成动图并保存为GIF或视频文件
-
使用Matplotlib库生成动图:
Matplotlib是一个强大的绘图库,可以生成静态、动画和交互式图表。下面是一个简单的例子,使用Matplotlib生成动图并保存为GIF文件:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
x = np.arange(0, 2*np.pi, 0.01)
line, = ax.plot(x, np.sin(x))
def update(num, x, line):
line.set_ydata(np.sin(x + num / 10.0))
return line,
ani = animation.FuncAnimation(fig, update, frames=100, fargs=[x, line], interval=50)
ani.save('sine_wave.gif', writer='imagemagick', fps=30)
-
使用Pillow库保存动图:
Pillow是Python Imaging Library(PIL)的一个分支,支持许多图像文件格式。你可以使用Pillow库来保存动图:
from PIL import Image
images = []
for i in range(100):
fig, ax = plt.subplots()
x = np.arange(0, 2*np.pi, 0.01)
y = np.sin(x + i / 10.0)
plt.plot(x, y)
plt.savefig(f'frame_{i}.png')
images.append(Image.open(f'frame_{i}.png'))
images[0].save('sine_wave.gif', save_all=True, append_images=images[1:], duration=50, loop=0)
-
使用FFmpeg库生成视频文件:
FFmpeg是一个多媒体处理工具,可以处理视频、音频和其他多媒体文件。你可以使用FFmpeg库将生成的动图保存为视频文件:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
x = np.arange(0, 2*np.pi, 0.01)
line, = ax.plot(x, np.sin(x))
def update(num, x, line):
line.set_ydata(np.sin(x + num / 10.0))
return line,
ani = animation.FuncAnimation(fig, update, frames=100, fargs=[x, line], interval=50)
ani.save('sine_wave.mp4', writer='ffmpeg', fps=30)
三、利用在线平台
-
使用Google Colab:
Google Colab是一个基于云的Jupyter Notebook环境,允许用户在浏览器中编写和运行Python代码。你可以在Google Colab中编写生成动图的Python代码,并直接在浏览器中查看动图。
from google.colab import output
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
x = np.arange(0, 2*np.pi, 0.01)
line, = ax.plot(x, np.sin(x))
def update(num, x, line):
line.set_ydata(np.sin(x + num / 10.0))
return line,
ani = animation.FuncAnimation(fig, update, frames=100, fargs=[x, line], interval=50)
ani.save('sine_wave.gif', writer='imagemagick', fps=30)
output.eval_js('new Audio("sine_wave.gif").play()')
-
使用Kaggle Kernels:
Kaggle Kernels是Kaggle提供的一个在线代码执行平台,允许用户在浏览器中编写和运行Python代码。你可以在Kaggle Kernels中编写生成动图的Python代码,并直接在浏览器中查看动图。
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
x = np.arange(0, 2*np.pi, 0.01)
line, = ax.plot(x, np.sin(x))
def update(num, x, line):
line.set_ydata(np.sin(x + num / 10.0))
return line,
ani = animation.FuncAnimation(fig, update, frames=100, fargs=[x, line], interval=50)
ani.save('sine_wave.gif', writer='imagemagick', fps=30)
四、使用移动应用程序
-
Juno:
Juno是一款iOS应用程序,允许用户在iPhone和iPad上运行Jupyter Notebook。通过Juno,你可以在移动设备上编写、运行Python代码,并查看生成的动图。
-
Pydroid 3:
Pydroid 3是一款Android应用程序,提供了一个完整的Python 3环境。通过Pydroid 3,你可以在Android设备上编写、运行Python代码,并查看生成的动图。
五、其他注意事项
-
优化动图的性能:
在生成动图时,注意优化性能。例如,减少动图的分辨率和帧数,可以显著降低文件大小和加载时间,提高查看体验。
-
保持代码简洁:
在编写生成动图的Python代码时,保持代码简洁、易读。这样可以方便调试和维护,提高代码质量。
-
使用合适的工具:
根据需求选择合适的工具和库。例如,Matplotlib适用于生成简单的动图,而Pillow和FFmpeg适用于保存和处理图像和视频文件。
通过以上方法和注意事项,你可以轻松地在手机上查看Python生成的动图。这些方法不仅提高了工作效率,还为移动设备上的数据可视化和分析提供了便利。希望本文能对你有所帮助,并激发你在移动设备上探索更多的Python应用。
相关问答FAQs:
如何在手机上查看Python生成的动图?
要在手机上查看Python生成的动图,您可以使用一些常见的应用程序和方法。首先,确保您在Python中将动图保存为兼容的格式,如GIF。然后,您可以通过电子邮件将动图发送到您的手机,或使用云存储服务(如Google Drive或Dropbox)上传文件。最后,使用手机上的图像查看器或专门的GIF播放器应用程序来查看动图。
Python动图的最佳格式是什么?
对于动图,GIF格式是最常用和广泛支持的。它能够保留动画效果并且在大多数设备和平台上都能顺利播放。为了确保最佳的图像质量和兼容性,您可以使用Python库如Matplotlib或Pillow来生成和保存GIF动图。
手机查看Python动图时遇到的问题该如何解决?
如果在手机上查看Python生成的动图时遇到问题,可以尝试以下几种解决方案。首先,检查动图是否已正确下载并且没有损坏。其次,确保您使用的图像查看应用程序支持GIF格式。如果动图仍然无法播放,尝试使用其他应用程序或重新生成动图以确保文件的完整性。
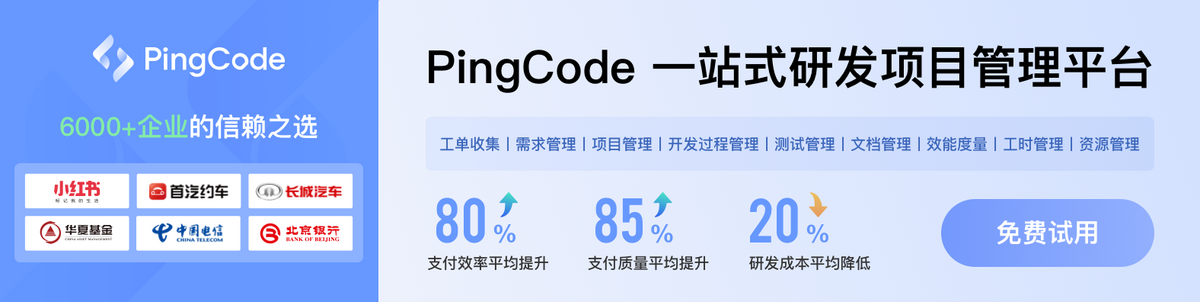