Python程序读取多张图片大小的方法
要读取多张图片的大小,Python 提供了多种工具和库来处理图像数据。使用Pillow库、使用OpenCV库、批量处理图像文件、获取图像尺寸是读取图片大小的常用方法。本文将详细介绍如何使用这些工具和技术来实现这一任务。
一、使用Pillow库
Pillow 是一个广泛使用的Python图像处理库,基于PIL(Python Imaging Library)开发,提供了强大的图像处理能力。
安装Pillow
首先,你需要确保已经安装了Pillow库。如果没有安装,可以使用以下命令安装:
pip install pillow
读取单张图片的大小
使用Pillow读取单张图片的大小非常简单。下面是一个示例代码:
from PIL import Image
def get_image_size(image_path):
with Image.open(image_path) as img:
return img.size # 返回 (width, height)
示例
image_path = 'example.jpg'
print(get_image_size(image_path))
读取多张图片的大小
要读取多张图片的大小,可以使用循环来处理多个图像文件。下面是一个示例代码:
import os
from PIL import Image
def get_images_sizes(directory):
sizes = {}
for filename in os.listdir(directory):
if filename.endswith(('.png', '.jpg', '.jpeg', '.bmp', '.gif')):
file_path = os.path.join(directory, filename)
with Image.open(file_path) as img:
sizes[filename] = img.size
return sizes
示例
directory = 'path_to_images'
print(get_images_sizes(directory))
在上述代码中,get_images_sizes
函数遍历指定目录中的所有图像文件,并使用 Image.open
打开每个图像文件,然后获取其大小。
二、使用OpenCV库
OpenCV 是一个强大的计算机视觉库,提供了丰富的图像处理功能。使用OpenCV读取图像大小同样非常简单。
安装OpenCV
首先,你需要确保已经安装了OpenCV库。如果没有安装,可以使用以下命令安装:
pip install opencv-python
读取单张图片的大小
使用OpenCV读取单张图片的大小非常简单。下面是一个示例代码:
import cv2
def get_image_size(image_path):
img = cv2.imread(image_path)
return img.shape[1], img.shape[0] # 返回 (width, height)
示例
image_path = 'example.jpg'
print(get_image_size(image_path))
读取多张图片的大小
要读取多张图片的大小,可以使用循环来处理多个图像文件。下面是一个示例代码:
import os
import cv2
def get_images_sizes(directory):
sizes = {}
for filename in os.listdir(directory):
if filename.endswith(('.png', '.jpg', '.jpeg', '.bmp', '.gif')):
file_path = os.path.join(directory, filename)
img = cv2.imread(file_path)
sizes[filename] = (img.shape[1], img.shape[0])
return sizes
示例
directory = 'path_to_images'
print(get_images_sizes(directory))
在上述代码中,get_images_sizes
函数遍历指定目录中的所有图像文件,并使用 cv2.imread
打开每个图像文件,然后获取其大小。
三、批量处理图像文件
批量处理图像文件的关键在于如何高效地遍历目录并处理图像。除了使用循环遍历目录外,还可以使用一些高级技术来优化批量处理的效率。
使用多线程或多进程
对于大量图像文件,可以考虑使用多线程或多进程来提高处理速度。Python提供了threading
和multiprocessing
模块来实现多线程和多进程处理。
以下是使用多线程处理图像大小的示例代码:
import os
import threading
from PIL import Image
def get_image_size(image_path, sizes):
with Image.open(image_path) as img:
sizes[image_path] = img.size
def get_images_sizes(directory):
sizes = {}
threads = []
for filename in os.listdir(directory):
if filename.endswith(('.png', '.jpg', '.jpeg', '.bmp', '.gif')):
file_path = os.path.join(directory, filename)
thread = threading.Thread(target=get_image_size, args=(file_path, sizes))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
return sizes
示例
directory = 'path_to_images'
print(get_images_sizes(directory))
在上述代码中,get_image_size
函数在一个单独的线程中运行,get_images_sizes
函数创建并启动多个线程来并行处理图像文件。
使用异步IO
对于I/O密集型任务(如读取图像文件),异步IO可以显著提高效率。Python提供了asyncio
模块来实现异步IO。
以下是使用异步IO处理图像大小的示例代码:
import os
import asyncio
from PIL import Image
from concurrent.futures import ThreadPoolExecutor
async def get_image_size(image_path, sizes, loop):
with Image.open(image_path) as img:
sizes[image_path] = img.size
async def get_images_sizes(directory):
sizes = {}
loop = asyncio.get_event_loop()
with ThreadPoolExecutor() as executor:
tasks = []
for filename in os.listdir(directory):
if filename.endswith(('.png', '.jpg', '.jpeg', '.bmp', '.gif')):
file_path = os.path.join(directory, filename)
tasks.append(loop.run_in_executor(executor, get_image_size, file_path, sizes, loop))
await asyncio.gather(*tasks)
return sizes
示例
directory = 'path_to_images'
loop = asyncio.get_event_loop()
sizes = loop.run_until_complete(get_images_sizes(directory))
print(sizes)
在上述代码中,get_image_size
函数在一个线程池中运行,get_images_sizes
函数使用asyncio.gather
来并行处理图像文件。
四、获取图像尺寸的其他方法
除了使用Pillow和OpenCV库外,还有其他一些方法可以获取图像尺寸。例如,可以使用matplotlib
库来读取图像并获取其大小。
使用matplotlib库
matplotlib
是一个流行的Python绘图库,提供了丰富的图像处理功能。
首先,你需要确保已经安装了matplotlib库。如果没有安装,可以使用以下命令安装:
pip install matplotlib
以下是使用matplotlib
读取图像大小的示例代码:
import os
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
def get_image_size(image_path):
img = mpimg.imread(image_path)
return img.shape[1], img.shape[0] # 返回 (width, height)
def get_images_sizes(directory):
sizes = {}
for filename in os.listdir(directory):
if filename.endswith(('.png', '.jpg', '.jpeg', '.bmp', '.gif')):
file_path = os.path.join(directory, filename)
sizes[filename] = get_image_size(file_path)
return sizes
示例
directory = 'path_to_images'
print(get_images_sizes(directory))
在上述代码中,get_image_size
函数使用matplotlib.image.imread
来读取图像文件,然后获取其大小。
总结
本文介绍了在Python中读取多张图片大小的几种方法,包括使用Pillow库、使用OpenCV库、批量处理图像文件、获取图像尺寸等。每种方法都有其优点和适用场景,选择适合自己的方法可以提高图像处理的效率。
无论是处理单张图片还是批量处理多张图片,Python都提供了丰富的工具和库来实现这一任务。希望本文对你有所帮助,能够在实际项目中灵活应用这些技术来高效地处理图像数据。
相关问答FAQs:
如何使用Python获取多张图片的尺寸?
要获取多张图片的尺寸,您可以使用Python中的PIL库(Pillow)。首先,确保安装了Pillow库。可以通过命令pip install Pillow
来安装。接下来,使用以下代码读取图片并获取其尺寸:
from PIL import Image
import os
def get_image_sizes(image_folder):
sizes = {}
for image_file in os.listdir(image_folder):
if image_file.endswith(('jpg', 'jpeg', 'png', 'gif')): # 支持的图片格式
img_path = os.path.join(image_folder, image_file)
with Image.open(img_path) as img:
sizes[image_file] = img.size # 返回 (宽度, 高度)
return sizes
image_folder = 'path/to/your/images'
print(get_image_sizes(image_folder))
如何处理不同格式的图片以获取其尺寸?
不同格式的图片(如JPEG、PNG、GIF等)在读取时可能需要不同的处理方式。Pillow库支持多种格式,因此在使用Image.open()
时,您无需担心文件格式问题。只需确保在获取尺寸前检查文件扩展名,确保它们是受支持的格式即可。
可以使用哪些其他库读取图片尺寸?
除了Pillow,您还可以使用OpenCV、imageio等库来读取图片并获取尺寸。例如,使用OpenCV时,可以使用cv2.imread()
读取图片,然后通过shape
属性获取尺寸。以下是使用OpenCV的示例代码:
import cv2
import os
def get_image_sizes_with_opencv(image_folder):
sizes = {}
for image_file in os.listdir(image_folder):
if image_file.endswith(('jpg', 'jpeg', 'png', 'gif')):
img_path = os.path.join(image_folder, image_file)
img = cv2.imread(img_path)
sizes[image_file] = img.shape[1], img.shape[0] # (宽度, 高度)
return sizes
image_folder = 'path/to/your/images'
print(get_image_sizes_with_opencv(image_folder))
这些方法都可以有效地帮助您读取和处理多张图片的尺寸。
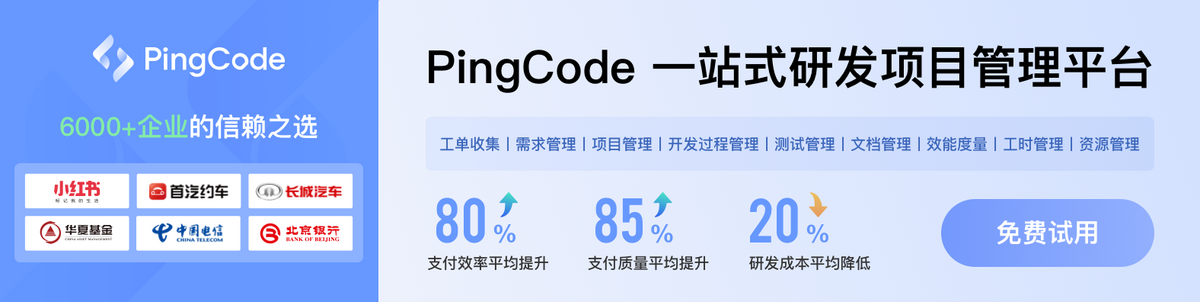