Python如何做搜索引擎
在使用Python创建搜索引擎时,选择合适的算法、使用高效的数据结构、实现爬虫功能、进行文本处理和分析、建立索引、提供查询接口、评估和优化性能是关键步骤。本文将详细介绍这些步骤,并提供一些实用的建议和技巧。
一、选择合适的算法
搜索引擎的核心在于选择合适的算法,这直接影响搜索结果的准确性和性能。常见的算法包括布尔搜索、向量空间模型和PageRank算法等。
布尔搜索
布尔搜索使用布尔逻辑(AND, OR, NOT)来组合查询词,从而实现简单的搜索功能。它的优点是实现简单,适用于小规模的数据集。以下是一个简单的布尔搜索实现例子:
def boolean_search(documents, query):
query_words = query.lower().split()
results = []
for doc in documents:
if all(word in doc.lower() for word in query_words):
results.append(doc)
return results
documents = ["Python is great", "Python is a snake", "I love programming in Python"]
query = "Python is"
print(boolean_search(documents, query))
向量空间模型
向量空间模型(Vector Space Model, VSM)将文档和查询表示为向量,通过计算向量之间的相似度(如余弦相似度)来判断文档的相关性。实现向量空间模型时,需要进行文本预处理(如分词、去停用词、词干提取)和TF-IDF计算。
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
def vector_space_search(documents, query):
vectorizer = TfidfVectorizer()
tfidf_matrix = vectorizer.fit_transform(documents + [query])
cosine_similarities = cosine_similarity(tfidf_matrix[-1], tfidf_matrix[:-1])
return cosine_similarities.argsort()[0][::-1]
documents = ["Python is great", "Python is a snake", "I love programming in Python"]
query = "Python programming"
print(vector_space_search(documents, query))
二、使用高效的数据结构
选择合适的数据结构可以提高搜索引擎的性能。常见的数据结构包括倒排索引、B树和哈希表等。
倒排索引
倒排索引是一种常用的数据结构,用于快速查找包含某个词的文档。它由一个词典和一个文档列表组成,词典存储词和对应的文档列表。
from collections import defaultdict
def build_inverted_index(documents):
inverted_index = defaultdict(list)
for doc_id, doc in enumerate(documents):
for word in doc.lower().split():
inverted_index[word].append(doc_id)
return inverted_index
documents = ["Python is great", "Python is a snake", "I love programming in Python"]
inverted_index = build_inverted_index(documents)
print(inverted_index)
三、实现爬虫功能
爬虫(Crawler)是搜索引擎的重要组成部分,负责从互联网上收集数据。Python的requests
和BeautifulSoup
库可以方便地实现爬虫功能。
import requests
from bs4 import BeautifulSoup
def crawl(url):
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
return soup.get_text()
return ""
url = "https://www.example.com"
print(crawl(url))
四、进行文本处理和分析
文本处理和分析是搜索引擎的基础,包括分词、去停用词、词干提取等。
分词
分词是将文本分解为单词或短语的过程。Python的nltk
库提供了强大的分词工具。
import nltk
nltk.download('punkt')
def tokenize(text):
return nltk.word_tokenize(text)
text = "I love programming in Python"
print(tokenize(text))
去停用词
停用词是指对文本分析无关紧要的高频词,如“the”、“is”等。去停用词可以提高搜索引擎的性能。
from nltk.corpus import stopwords
nltk.download('stopwords')
def remove_stopwords(words):
stop_words = set(stopwords.words('english'))
return [word for word in words if word not in stop_words]
words = tokenize("I love programming in Python")
print(remove_stopwords(words))
五、建立索引
建立索引是搜索引擎的重要步骤,倒排索引是常用的索引结构。通过倒排索引,可以快速查找包含查询词的文档。
def build_index(documents):
index = defaultdict(list)
for doc_id, doc in enumerate(documents):
words = remove_stopwords(tokenize(doc))
for word in words:
index[word].append(doc_id)
return index
documents = ["Python is great", "Python is a snake", "I love programming in Python"]
index = build_index(documents)
print(index)
六、提供查询接口
提供查询接口是搜索引擎的最终目标,通过查询接口,用户可以方便地搜索相关文档。
def search(index, query):
query_words = remove_stopwords(tokenize(query))
results = set(index[query_words[0]])
for word in query_words[1:]:
results &= set(index[word])
return results
query = "Python programming"
print(search(index, query))
七、评估和优化性能
评估和优化性能是搜索引擎开发的重要环节。常见的评估指标包括精准率、召回率和F1值。优化性能的方法包括使用缓存、并行计算和分布式计算等。
精准率和召回率
精准率是指正确检索到的文档数占检索到的文档总数的比例,召回率是指正确检索到的文档数占相关文档总数的比例。
def precision_recall(true_positive, false_positive, false_negative):
precision = true_positive / (true_positive + false_positive)
recall = true_positive / (true_positive + false_negative)
return precision, recall
true_positive = 5
false_positive = 2
false_negative = 3
print(precision_recall(true_positive, false_positive, false_negative))
使用缓存
缓存可以减少重复计算,提高搜索引擎的性能。Python的functools.lru_cache
装饰器可以方便地实现缓存功能。
from functools import lru_cache
@lru_cache(maxsize=100)
def expensive_function(x):
return x * x
print(expensive_function(10))
并行计算
并行计算可以提高搜索引擎的性能,Python的multiprocessing
库提供了并行计算的功能。
from multiprocessing import Pool
def square(x):
return x * x
with Pool(4) as p:
print(p.map(square, [1, 2, 3, 4]))
分布式计算
分布式计算可以处理大规模数据,Hadoop和Spark是常用的分布式计算框架。Python的pyspark
库提供了与Spark的接口。
from pyspark import SparkContext
sc = SparkContext("local", "Simple App")
data = [1, 2, 3, 4, 5]
dist_data = sc.parallelize(data)
print(dist_data.map(lambda x: x * x).collect())
结论
通过以上步骤,我们可以使用Python创建一个简单但功能强大的搜索引擎。选择合适的算法、使用高效的数据结构、实现爬虫功能、进行文本处理和分析、建立索引、提供查询接口、评估和优化性能是关键步骤。希望本文对您有所帮助,祝您在搜索引擎开发中取得成功。
相关问答FAQs:
Python可以用于构建搜索引擎吗?
是的,Python非常适合构建搜索引擎。它拥有强大的库和框架,如Scrapy用于数据抓取,Whoosh和Elasticsearch用于索引和搜索功能。Python的简单语法和丰富的社区支持使得开发自定义搜索引擎变得相对容易,尤其是在处理文本数据和实现自然语言处理时。
使用Python构建搜索引擎需要哪些基本组件?
构建搜索引擎通常需要几个关键组件:数据抓取器、数据存储、索引器和查询处理器。数据抓取器用于从互联网上提取信息,数据存储可以使用数据库或文件系统,索引器用于建立可快速搜索的数据结构,而查询处理器则负责处理用户的搜索请求并返回结果。
如何提升Python搜索引擎的搜索性能?
提升搜索性能可以通过多种方式实现。优化索引结构和算法是关键,可以考虑使用倒排索引来加速搜索。此外,使用缓存机制来存储常见查询的结果也能显著提高响应速度。并且,合理设计数据模型和使用多线程或异步处理可以有效提升整体性能。
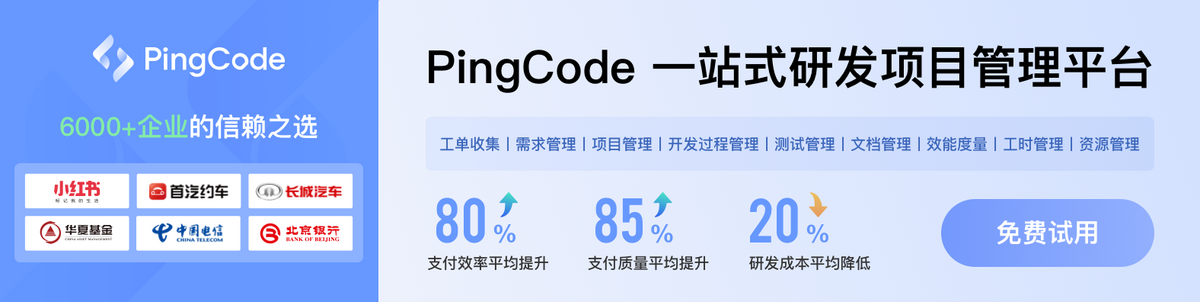