使用Python输出文件到文件夹的方法有很多:使用内置的open()函数、使用os模块创建目录、使用路径拼接函数os.path.join()。其中,使用open()函数是最常用的方法。
在Python中,文件处理是一个非常常见的任务。我们通常需要将数据写入文件,并将这些文件存储在特定的文件夹中。为了实现这个目标,我们需要掌握几个关键步骤:使用open()函数打开文件、使用write()方法将数据写入文件、使用os模块创建目录、使用os.path.join()函数组合路径。下面我们将详细介绍这些步骤。
一、使用open()函数写入文件
在Python中,最基本的文件操作是使用内置的open()函数。这个函数用于打开一个文件,并返回一个文件对象。我们可以通过文件对象来读写文件。
with open('example.txt', 'w') as file:
file.write('Hello, world!')
在这个例子中,我们使用open()函数打开了一个名为example.txt的文件,并使用write()方法将字符串'Hello, world!'写入文件。注意,这里我们使用了with语句,这是一种上下文管理器,可以确保文件在操作完成后自动关闭。
二、使用os模块创建目录
有时候,我们需要将文件写入特定的目录。如果目录不存在,我们需要先创建目录。os模块提供了创建目录的函数。
import os
directory = 'output_folder'
if not os.path.exists(directory):
os.makedirs(directory)
在这个例子中,我们首先检查output_folder目录是否存在。如果目录不存在,我们使用os.makedirs()函数创建目录。
三、使用os.path.join()函数组合路径
当我们处理文件路径时,手动组合路径容易出错。os.path.join()函数可以帮助我们组合路径,并自动处理路径分隔符问题。
import os
directory = 'output_folder'
filename = 'example.txt'
filepath = os.path.join(directory, filename)
with open(filepath, 'w') as file:
file.write('Hello, world!')
在这个例子中,我们使用os.path.join()函数将目录和文件名组合成完整的文件路径,并使用open()函数打开文件进行写入操作。
四、示例:将数据写入文件并存储在指定目录中
结合以上步骤,我们可以编写一个完整的示例,将数据写入文件并存储在指定目录中。
import os
def save_to_file(directory, filename, data):
if not os.path.exists(directory):
os.makedirs(directory)
filepath = os.path.join(directory, filename)
with open(filepath, 'w') as file:
file.write(data)
使用示例
directory = 'output_folder'
filename = 'example.txt'
data = 'Hello, world!'
save_to_file(directory, filename, data)
在这个示例中,我们定义了一个名为save_to_file()的函数。这个函数接受目录、文件名和数据作为参数。如果目录不存在,函数会创建目录,并将数据写入指定文件。
五、处理不同类型的数据
在实际应用中,我们可能需要处理不同类型的数据,例如字符串、列表、字典等。我们可以扩展save_to_file()函数,处理这些不同类型的数据。
import os
import json
def save_to_file(directory, filename, data):
if not os.path.exists(directory):
os.makedirs(directory)
filepath = os.path.join(directory, filename)
with open(filepath, 'w') as file:
if isinstance(data, str):
file.write(data)
elif isinstance(data, (list, dict)):
json.dump(data, file)
else:
raise TypeError('Unsupported data type')
使用示例
directory = 'output_folder'
filename = 'example.json'
data = {'message': 'Hello, world!'}
save_to_file(directory, filename, data)
在这个示例中,我们扩展了save_to_file()函数,使用json模块处理列表和字典类型的数据。对于字符串类型的数据,函数仍然使用write()方法进行写入。
六、处理文件名冲突
在某些情况下,文件名可能会发生冲突。例如,如果我们尝试写入一个已经存在的文件,我们可能希望避免覆盖原文件。我们可以通过添加时间戳或序列号来避免文件名冲突。
import os
import time
def save_to_file(directory, filename, data):
if not os.path.exists(directory):
os.makedirs(directory)
filepath = os.path.join(directory, filename)
base, ext = os.path.splitext(filepath)
counter = 1
while os.path.exists(filepath):
filepath = f"{base}_{counter}{ext}"
counter += 1
with open(filepath, 'w') as file:
file.write(data)
使用示例
directory = 'output_folder'
filename = 'example.txt'
data = 'Hello, world!'
save_to_file(directory, filename, data)
在这个示例中,我们使用os.path.splitext()函数拆分文件路径,并在文件名冲突时添加序列号,以避免覆盖原文件。
七、扩展示例:保存日志文件
我们可以将以上方法应用于实际项目中,例如保存日志文件。下面是一个简单的日志记录示例,将日志消息写入文件,并存储在指定目录中。
import os
import time
class Logger:
def __init__(self, directory):
self.directory = directory
if not os.path.exists(directory):
os.makedirs(directory)
def log(self, message):
timestamp = time.strftime('%Y-%m-%d_%H-%M-%S')
filename = f"log_{timestamp}.txt"
filepath = os.path.join(self.directory, filename)
with open(filepath, 'w') as file:
file.write(message)
使用示例
logger = Logger('logs')
logger.log('This is a log message.')
在这个示例中,我们定义了一个Logger类。这个类在初始化时创建日志目录,并提供一个log()方法,将日志消息写入带有时间戳的文件。
八、总结
通过学习上述内容,我们掌握了如何使用Python将文件输出到指定文件夹。具体步骤包括使用open()函数写入文件、使用os模块创建目录、使用os.path.join()函数组合路径。此外,我们还学习了如何处理不同类型的数据、解决文件名冲突,以及应用于实际项目中,例如保存日志文件。
希望这些内容对你有所帮助,能够在你的Python编程中更加自如地处理文件操作任务。
相关问答FAQs:
如何在Python中指定输出文件的路径?
在Python中,可以使用文件路径来指定输出文件的位置。通过在打开文件时提供完整路径,比如 open('/path/to/your/folder/output.txt', 'w')
,你可以将输出直接写入指定的文件夹。确保该路径存在,否则会引发错误。
Python支持哪些文件格式进行输出?
Python支持多种文件格式进行输出,包括文本文件(.txt)、CSV文件(.csv)、JSON文件(.json)、Excel文件(.xlsx)等。你可以根据需要选择适合的库和方法进行不同格式的输出,比如使用csv
模块输出CSV文件,或使用json
模块输出JSON格式的数据。
如何处理文件夹不存在的情况?
在进行文件输出前,可以使用os
模块检查目标文件夹是否存在。如果不存在,可以使用os.makedirs()
创建文件夹。例如,先检查文件夹路径,如果不存在就创建它,这样可以避免在写入文件时出现错误。
import os
folder_path = '/path/to/your/folder'
if not os.path.exists(folder_path):
os.makedirs(folder_path)
with open(os.path.join(folder_path, 'output.txt'), 'w') as f:
f.write('Hello, World!')
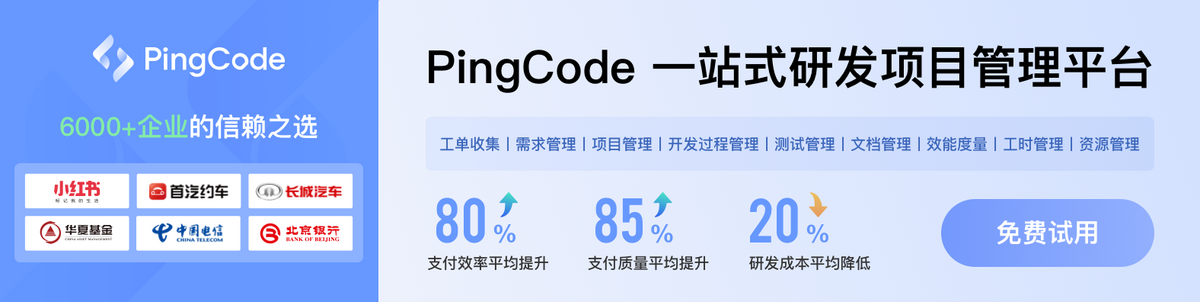