Python如何打开网页里的网址
使用Python打开网页里的网址,可以通过以下几种方式:使用requests库获取网页内容、使用BeautifulSoup库解析网页获取链接、使用webbrowser模块打开链接、结合selenium库模拟浏览器操作。其中,使用requests库获取网页内容是一个常见且方便的方法,接下来我将详细描述这一点。
使用requests库获取网页内容:首先,requests库是一个简单易用的HTTP库,可以通过它发送HTTP请求并获取响应。通过requests库,我们可以获取网页的HTML内容,然后再使用BeautifulSoup库解析这些内容,从中提取出我们需要的链接。具体步骤如下:
-
安装requests和BeautifulSoup库:
pip install requests
pip install beautifulsoup4
-
使用requests库发送HTTP请求获取网页内容:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
else:
print('Failed to retrieve the webpage')
-
使用BeautifulSoup解析网页内容并提取链接:
soup = BeautifulSoup(html_content, 'html.parser')
links = soup.find_all('a')
for link in links:
href = link.get('href')
if href:
print(href)
-
使用webbrowser模块打开链接:
import webbrowser
for link in links:
href = link.get('href')
if href:
webbrowser.open(href)
通过上述步骤,我们就可以使用Python获取网页内容,并从中提取出所有的链接,然后使用webbrowser模块打开这些链接。
一、安装和使用requests库
requests库是一个用于发送HTTP请求的第三方库,它非常简洁易用。首先,我们需要安装requests库,可以通过以下命令进行安装:
pip install requests
安装完成后,我们就可以使用requests库发送HTTP请求并获取网页内容。以下是一个简单的示例:
import requests
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print('Failed to retrieve the webpage')
在这个示例中,我们首先导入了requests库,然后使用requests.get(url)
方法发送了一个HTTP GET请求,并将响应存储在response
变量中。接着,我们检查了响应的状态码,如果状态码为200,表示请求成功,我们就可以获取网页的HTML内容并打印出来。
二、使用BeautifulSoup解析网页内容
获取到网页的HTML内容后,我们可以使用BeautifulSoup库来解析这些内容,并从中提取出我们需要的信息。BeautifulSoup是一个用于解析HTML和XML文档的第三方库,它可以方便地从网页中提取数据。
首先,我们需要安装BeautifulSoup库,可以通过以下命令进行安装:
pip install beautifulsoup4
安装完成后,我们就可以使用BeautifulSoup库解析网页内容并提取链接。以下是一个示例:
from bs4 import BeautifulSoup
html_content = '''<!DOCTYPE html>
<html>
<head>
<title>Example</title>
</head>
<body>
<a href="https://example.com/page1">Page 1</a>
<a href="https://example.com/page2">Page 2</a>
<a href="https://example.com/page3">Page 3</a>
</body>
</html>'''
soup = BeautifulSoup(html_content, 'html.parser')
links = soup.find_all('a')
for link in links:
href = link.get('href')
if href:
print(href)
在这个示例中,我们首先导入了BeautifulSoup库,然后定义了一个HTML字符串html_content
。接着,我们使用BeautifulSoup(html_content, 'html.parser')
方法创建了一个BeautifulSoup对象,并使用soup.find_all('a')
方法找到了所有的<a>
标签。最后,我们遍历了所有的<a>
标签,并打印了它们的href
属性。
三、使用webbrowser模块打开链接
在提取到网页中的链接后,我们可以使用webbrowser模块来打开这些链接。webbrowser模块是Python标准库中的一个模块,它可以在默认的浏览器中打开URL。
以下是一个示例:
import webbrowser
links = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
for link in links:
webbrowser.open(link)
在这个示例中,我们首先导入了webbrowser模块,然后定义了一个包含多个链接的列表links
。接着,我们遍历了所有的链接,并使用webbrowser.open(link)
方法在默认的浏览器中打开每一个链接。
四、结合使用requests、BeautifulSoup和webbrowser
通过结合使用requests、BeautifulSoup和webbrowser模块,我们可以实现从网页中提取链接并在浏览器中打开这些链接的完整流程。以下是一个完整的示例:
import requests
from bs4 import BeautifulSoup
import webbrowser
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
links = soup.find_all('a')
for link in links:
href = link.get('href')
if href:
webbrowser.open(href)
else:
print('Failed to retrieve the webpage')
在这个示例中,我们首先使用requests库获取了网页的HTML内容,然后使用BeautifulSoup解析了这些内容并提取出了所有的链接,最后使用webbrowser模块在浏览器中打开了这些链接。
五、处理相对链接
在实际应用中,我们可能会遇到相对链接。相对链接是指不包含完整URL的链接,它们通常以/
开头。为了处理相对链接,我们可以使用urllib.parse模块中的urljoin
方法将相对链接转换为绝对链接。
以下是一个示例:
import requests
from bs4 import BeautifulSoup
import webbrowser
from urllib.parse import urljoin
base_url = 'https://example.com'
response = requests.get(base_url)
if response.status_code == 200:
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
links = soup.find_all('a')
for link in links:
href = link.get('href')
if href:
absolute_url = urljoin(base_url, href)
webbrowser.open(absolute_url)
else:
print('Failed to retrieve the webpage')
在这个示例中,我们首先导入了urljoin
方法,然后在提取链接后使用urljoin(base_url, href)
方法将相对链接转换为了绝对链接。这样,我们就可以处理相对链接并在浏览器中打开它们。
六、处理重定向和错误
在实际应用中,我们可能会遇到重定向和错误。为了处理这些情况,我们可以使用requests库中的一些选项,例如allow_redirects
和timeout
。
以下是一个示例:
import requests
from bs4 import BeautifulSoup
import webbrowser
from urllib.parse import urljoin
base_url = 'https://example.com'
try:
response = requests.get(base_url, allow_redirects=True, timeout=10)
response.raise_for_status()
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
links = soup.find_all('a')
for link in links:
href = link.get('href')
if href:
absolute_url = urljoin(base_url, href)
webbrowser.open(absolute_url)
except requests.exceptions.RequestException as e:
print(f'Error: {e}')
在这个示例中,我们使用了allow_redirects=True
选项来允许重定向,使用了timeout=10
选项来设置超时时间为10秒,并使用了response.raise_for_status()
方法来检查响应的状态码。如果发生请求异常,我们会捕获异常并打印错误信息。
七、使用Selenium模拟浏览器操作
除了使用requests和BeautifulSoup库外,我们还可以使用Selenium库来模拟浏览器操作,从而打开网页并提取链接。Selenium是一个用于自动化浏览器操作的第三方库,它可以模拟用户在浏览器中的操作,例如点击链接、填写表单等。
首先,我们需要安装Selenium库和浏览器驱动程序,例如ChromeDriver。可以通过以下命令安装Selenium库:
pip install selenium
然后,我们需要下载ChromeDriver,并将其路径添加到系统环境变量中。以下是一个使用Selenium的示例:
from selenium import webdriver
url = 'https://example.com'
driver = webdriver.Chrome()
driver.get(url)
links = driver.find_elements_by_tag_name('a')
for link in links:
href = link.get_attribute('href')
if href:
print(href)
driver.quit()
在这个示例中,我们首先导入了Selenium库,然后创建了一个Chrome浏览器实例,并使用driver.get(url)
方法打开了网页。接着,我们使用driver.find_elements_by_tag_name('a')
方法找到了所有的<a>
标签,并使用link.get_attribute('href')
方法获取了它们的href
属性。最后,我们打印了所有的链接,并关闭了浏览器。
八、处理JavaScript生成的内容
在一些网页中,内容是通过JavaScript生成的,requests和BeautifulSoup库无法获取这些内容。这时,我们可以使用Selenium库来处理JavaScript生成的内容。
以下是一个示例:
from selenium import webdriver
url = 'https://example.com'
driver = webdriver.Chrome()
driver.get(url)
等待页面加载完成
driver.implicitly_wait(10)
提取链接
links = driver.find_elements_by_tag_name('a')
for link in links:
href = link.get_attribute('href')
if href:
print(href)
driver.quit()
在这个示例中,我们使用了driver.implicitly_wait(10)
方法来等待页面加载完成。这样,我们就可以处理JavaScript生成的内容,并提取出网页中的链接。
九、总结
通过上述内容,我们详细介绍了如何使用Python打开网页里的网址,包括使用requests库获取网页内容、使用BeautifulSoup库解析网页获取链接、使用webbrowser模块打开链接、结合selenium库模拟浏览器操作等方法。同时,我们还介绍了如何处理相对链接、重定向和错误,以及如何处理JavaScript生成的内容。
这些方法在实际应用中各有优势,可以根据具体需求选择合适的方法来实现从网页中提取链接并打开这些链接的功能。无论是简单的网页内容提取还是复杂的浏览器自动化操作,Python都提供了强大的工具和库来帮助我们完成任务。
相关问答FAQs:
如何使用Python打开一个特定的网页?
您可以使用Python的webbrowser
模块轻松打开特定的网页。只需导入该模块并调用open()
函数,传入您想访问的URL即可。例如:
import webbrowser
webbrowser.open('https://www.example.com')
这段代码会在默认浏览器中打开指定的网页。
在Python中如何提取网页中的所有链接?
可以使用requests
和BeautifulSoup
库来抓取网页并提取其中的所有链接。首先,使用requests
库获取网页内容,然后通过BeautifulSoup
解析HTML并提取所有的<a>
标签中的href
属性。例如:
import requests
from bs4 import BeautifulSoup
response = requests.get('https://www.example.com')
soup = BeautifulSoup(response.text, 'html.parser')
links = [a['href'] for a in soup.find_all('a', href=True)]
这段代码将返回网页中所有链接的列表。
使用Python如何自动化打开多个网页?
您可以通过循环来实现自动化打开多个网页。将所有网址存储在一个列表中,然后遍历该列表,使用webbrowser.open()
函数打开每一个网址。例如:
import webbrowser
urls = ['https://www.example1.com', 'https://www.example2.com', 'https://www.example3.com']
for url in urls:
webbrowser.open(url)
这样,您可以一次性打开多个网页,提升工作效率。
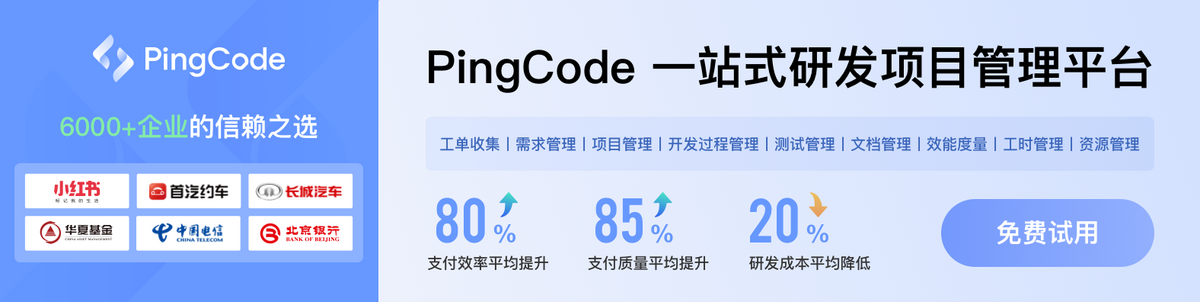