在Python中,输入时另起一行的主要方法有使用多行字符串、使用换行符(\n)、使用循环读取输入。通过这些方法,可以方便地处理多行输入,满足不同情境的需求。下面是对其中一种方法的详细描述:
使用多行字符串:Python支持使用三引号(''' 或 """)来表示多行字符串,用户可以在输入时直接输入多行内容,直到结束输入的标记。这种方式简单直观,非常适合处理多行文本输入。
# Example of using triple quotes for multiline input
user_input = '''First line of input
Second line of input
Third line of input'''
print(user_input)
这种方法直接在代码中定义了多行字符串,非常适合用于静态多行输入的情境。
一、使用换行符(\n)
在Python中,可以使用换行符“\n”来另起一行输入。这个方法常用于字符串操作,可以将多个输入连接成一个包含换行符的字符串,从而实现多行输入。
# Example of using newline character for multiline input
user_input = "First line of input\nSecond line of input\nThird line of input"
print(user_input)
换行符方法非常灵活,可以通过字符串拼接、格式化等方式动态生成多行输入。
二、使用循环读取输入
对于需要动态获取用户输入的情境,可以使用循环来逐行读取用户输入,直到满足某个条件(如输入空行或特定的结束标记)为止。
# Example of using loop to read multiple lines of input
print("Enter your input (type 'END' to finish):")
lines = []
while True:
line = input()
if line == "END":
break
lines.append(line)
user_input = "\n".join(lines)
print(user_input)
这种方法非常适合处理用户交互式输入,可以根据实际需求灵活控制输入结束条件。
三、使用多行字符串输入
Python支持使用三引号(''' 或 """)来表示多行字符串,用户可以在输入时直接输入多行内容,直到结束输入的标记。这种方式简单直观,非常适合处理多行文本输入。
# Example of using triple quotes for multiline input
user_input = '''First line of input
Second line of input
Third line of input'''
print(user_input)
这种方法直接在代码中定义了多行字符串,非常适合用于静态多行输入的情境。
四、使用文本文件输入
有时,输入的内容可能比较长,或者需要从文件中读取多行输入。这时可以将多行内容存储在一个文本文件中,然后通过读取文件内容来实现多行输入。
# Example of reading multiline input from a file
with open("input.txt", "r") as file:
user_input = file.read()
print(user_input)
这种方法适用于需要从文件中读取大量输入的场景,非常实用。
五、使用多行输入控件(GUI)
在图形用户界面(GUI)编程中,可以使用多行输入控件(如Tkinter的Text控件)来实现多行输入。在这种情况下,用户可以在图形界面中输入多行内容,程序可以获取并处理这些输入。
# Example of using Tkinter Text widget for multiline input
import tkinter as tk
def get_input():
user_input = text_widget.get("1.0", tk.END)
print(user_input)
root = tk.Tk()
text_widget = tk.Text(root, height=10, width=40)
text_widget.pack()
submit_button = tk.Button(root, text="Submit", command=get_input)
submit_button.pack()
root.mainloop()
这种方法适用于开发图形用户界面的应用程序,可以提供更友好的用户输入体验。
六、使用正则表达式处理多行输入
在某些高级应用场景中,可能需要使用正则表达式来处理和分析多行输入。Python的re
模块支持多行模式,可以方便地对多行输入进行匹配和提取。
# Example of using regular expressions to process multiline input
import re
user_input = """First line of input
Second line of input
Third line of input"""
pattern = re.compile(r"(?m)^(.*input)$")
matches = pattern.findall(user_input)
for match in matches:
print(match)
这种方法适用于需要对多行输入进行复杂模式匹配和处理的情况。
七、使用JSON格式处理多行输入
在处理结构化多行输入时,可以使用JSON格式来方便地解析和处理输入内容。Python的json
模块可以将JSON字符串转换为Python对象,便于进一步处理。
# Example of using JSON format for multiline input
import json
user_input = """{
"lines": [
"First line of input",
"Second line of input",
"Third line of input"
]
}"""
data = json.loads(user_input)
for line in data["lines"]:
print(line)
这种方法适用于需要处理结构化数据的多行输入场景,特别是在与API接口交互时非常有用。
八、使用命令行参数处理多行输入
在某些命令行工具中,可以通过命令行参数传递多行输入。Python的argparse
模块提供了丰富的命令行参数解析功能,可以方便地处理多行输入。
# Example of using argparse for multiline input
import argparse
parser = argparse.ArgumentParser(description="Process multiline input.")
parser.add_argument("input", nargs="+", help="Lines of input")
args = parser.parse_args()
user_input = "\n".join(args.input)
print(user_input)
这种方法适用于开发命令行工具,可以通过命令行参数传递多行输入,方便在脚本和工具中使用。
综上所述,Python提供了多种方式来处理多行输入,适用于不同的应用场景。根据具体需求选择合适的方法,可以有效提高代码的灵活性和可维护性。
相关问答FAQs:
如何在Python中实现多行输入?
在Python中,可以使用循环结构来实现多行输入。用户可以通过输入特定的结束标识符来终止输入。例如,可以让用户输入数据直到输入一个空行为止。示例代码如下:
lines = []
while True:
line = input("请输入内容(输入空行结束):")
if line == "":
break
lines.append(line)
print("您输入的内容是:")
print("\n".join(lines))
如何在Python中格式化输出以显示多行内容?
在Python中,可以使用print()
函数的特殊参数来格式化输出。可以通过添加换行符\n
来实现多行显示。例如:
text = "第一行\n第二行\n第三行"
print(text)
这样可以在控制台中以多行的形式输出内容。
在Python中如何处理用户输入的换行符?
如果用户输入的字符串中包含换行符,Python会将其视为字符串的一部分。可以使用str.splitlines()
方法来将字符串按行分割为列表。例如:
user_input = "第一行\n第二行\n第三行"
lines = user_input.splitlines()
for line in lines:
print(line)
这种方式可以有效处理用户输入中的多行内容。
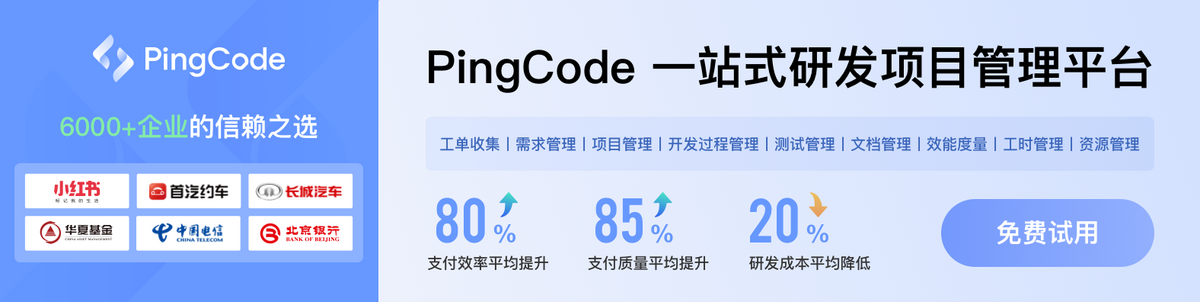