在Python中,可以通过多种方式将文件传入到类中,比如使用文件路径、文件对象或文件内容。最常见的方法包括:使用open
函数打开文件并将文件对象传递给类构造函数、直接将文件内容读取到一个字符串并传递给类构造函数、或使用文件路径并在类中处理文件读取。最推荐的是使用文件路径并在类中处理文件读取,因为这样可以更好地管理文件的打开和关闭。
详细描述: 使用文件路径并在类中处理文件读取是最灵活和安全的方法。这种方法允许类内部控制文件的打开和关闭操作,确保资源得到正确管理,并且可以根据需要处理文件内容。下面将通过具体示例详细展示这种方法的实现。
一、使用文件路径
使用文件路径将文件传入类中是最常见的方法。通过在类的构造函数中传递文件路径,类可以灵活地处理文件的打开、读取和关闭操作。
class FileHandler:
def __init__(self, file_path):
self.file_path = file_path
self.content = self.read_file()
def read_file(self):
with open(self.file_path, 'r') as file:
content = file.read()
return content
def display_content(self):
print(self.content)
示例用法
file_handler = FileHandler('example.txt')
file_handler.display_content()
在上面的示例中,FileHandler
类接收一个文件路径,并在__init__
方法中调用read_file
方法读取文件内容。read_file
方法使用with open
语句打开文件,并将文件内容读取到self.content
中。然后,通过display_content
方法可以展示文件内容。
二、使用文件对象
除了文件路径,还可以直接传入一个已打开的文件对象。这种方法适用于在类外部已经打开文件,并希望在类内部进一步处理文件内容的情况。
class FileHandler:
def __init__(self, file_obj):
self.file_obj = file_obj
self.content = self.read_file()
def read_file(self):
content = self.file_obj.read()
self.file_obj.close()
return content
def display_content(self):
print(self.content)
示例用法
with open('example.txt', 'r') as file:
file_handler = FileHandler(file)
file_handler.display_content()
在上面的示例中,文件在类外部使用open
函数打开,并传入FileHandler
类。FileHandler
类的构造函数接收文件对象并读取内容。
三、使用文件内容
有时候,我们可能已经读取了文件内容,并希望将其传入类中进行处理。此时,可以直接将文件内容作为字符串传递给类。
class FileHandler:
def __init__(self, content):
self.content = content
def display_content(self):
print(self.content)
示例用法
with open('example.txt', 'r') as file:
content = file.read()
file_handler = FileHandler(content)
file_handler.display_content()
在这个示例中,文件内容在类外部读取并传入FileHandler
类。类的构造函数接收文件内容,并通过display_content
方法展示内容。
四、在类中处理不同类型的输入
有时候,我们可能希望我们的类能够处理多种类型的输入,比如文件路径、文件对象或文件内容。我们可以通过在构造函数中检查输入类型来实现这种灵活性。
class FileHandler:
def __init__(self, input_data):
if isinstance(input_data, str):
self.file_path = input_data
self.content = self.read_file_from_path()
elif hasattr(input_data, 'read'):
self.file_obj = input_data
self.content = self.read_file_from_obj()
else:
self.content = input_data
def read_file_from_path(self):
with open(self.file_path, 'r') as file:
content = file.read()
return content
def read_file_from_obj(self):
content = self.file_obj.read()
self.file_obj.close()
return content
def display_content(self):
print(self.content)
示例用法
file_handler = FileHandler('example.txt')
file_handler.display_content()
with open('example.txt', 'r') as file:
file_handler = FileHandler(file)
file_handler.display_content()
content = "This is the file content."
file_handler = FileHandler(content)
file_handler.display_content()
在这个示例中,FileHandler
类的构造函数检查输入数据的类型,如果是字符串,则假定为文件路径并调用read_file_from_path
方法;如果具有read
方法,则假定为文件对象并调用read_file_from_obj
方法;否则,假定输入数据已经是文件内容。
五、处理文件读取错误
在实际应用中,文件读取可能会遇到各种错误,比如文件不存在、权限不足等。为了提高代码的鲁棒性,我们可以在文件读取过程中添加错误处理机制。
class FileHandler:
def __init__(self, file_path):
self.file_path = file_path
self.content = self.read_file()
def read_file(self):
try:
with open(self.file_path, 'r') as file:
content = file.read()
return content
except FileNotFoundError:
print(f"Error: The file {self.file_path} does not exist.")
return None
except IOError:
print(f"Error: An error occurred while reading the file {self.file_path}.")
return None
def display_content(self):
if self.content:
print(self.content)
else:
print("No content to display.")
示例用法
file_handler = FileHandler('nonexistent.txt')
file_handler.display_content()
在这个示例中,read_file
方法使用try...except
语句捕获文件读取过程中可能发生的FileNotFoundError
和IOError
异常,并在发生错误时输出相应的错误信息。
总结
将文件传入类中可以通过多种方法实现,主要包括使用文件路径、文件对象或文件内容。最推荐的方法是使用文件路径并在类中处理文件读取,因为这种方法灵活、安全且易于管理。 在实际应用中,可以根据需要选择合适的方法,并考虑添加错误处理机制以提高代码的鲁棒性。通过这些方法,可以有效地将文件内容传入类中进行处理,从而实现更多的功能和应用。
相关问答FAQs:
如何在Python中将文件路径传递给类的实例?
在Python中,可以通过在类的构造函数中添加一个参数来传递文件路径。以下是一个示例代码:
class FileHandler:
def __init__(self, file_path):
self.file_path = file_path
self.content = self.read_file()
def read_file(self):
with open(self.file_path, 'r') as file:
return file.read()
# 使用示例
file_handler = FileHandler('example.txt')
print(file_handler.content)
这样,您就可以在创建类的实例时将文件路径传入。
如何在类中处理文件的读取和写入操作?
在类中处理文件的读取和写入操作,可以定义多个方法来分别处理这些功能。以下是一个示例:
class FileHandler:
def __init__(self, file_path):
self.file_path = file_path
def read_file(self):
with open(self.file_path, 'r') as file:
return file.read()
def write_file(self, content):
with open(self.file_path, 'w') as file:
file.write(content)
# 使用示例
file_handler = FileHandler('example.txt')
file_handler.write_file('Hello, World!')
print(file_handler.read_file())
此示例演示了如何在类中实现文件的读取和写入。
如何在Python类中处理不同类型的文件?
为了处理不同类型的文件,可以在类中定义不同的方法来处理各种文件格式,例如文本文件、CSV文件或JSON文件。以下是处理JSON文件的示例:
import json
class FileHandler:
def __init__(self, file_path):
self.file_path = file_path
def read_json(self):
with open(self.file_path, 'r') as file:
return json.load(file)
def write_json(self, data):
with open(self.file_path, 'w') as file:
json.dump(data, file)
# 使用示例
file_handler = FileHandler('data.json')
file_handler.write_json({'name': 'Alice', 'age': 30})
print(file_handler.read_json())
通过这种方式,您可以灵活处理不同类型的文件。
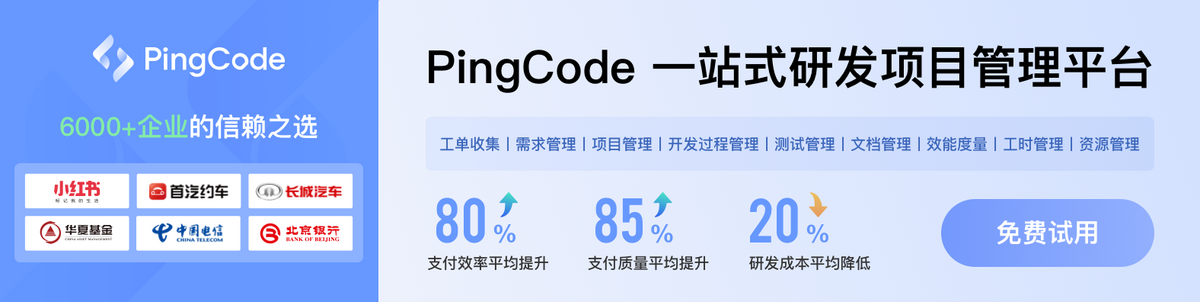