使用Python3来替换Shell的主要方法包括:使用os模块、subprocess模块、提供更好的错误处理和调试支持。Python3 提供了丰富的模块和库,可以帮助我们替换 Shell 脚本,以实现更加复杂和高效的系统管理任务。下面将详细描述这三种方法的实现。
一、使用os模块
os模块是Python的标准库之一,它提供了与操作系统进行交互的功能。通过os模块,我们可以执行文件、获取环境变量、操作文件系统等。
1、执行系统命令
使用os模块中的os.system()函数可以执行系统命令。这个函数会调用系统的shell来执行命令。
import os
执行ls命令
os.system('ls -la')
这种方法简单直接,但它有几个缺点:无法获取命令的输出,无法捕获错误信息,无法对命令参数进行更复杂的处理。
2、获取环境变量
我们可以使用os.environ来获取和设置环境变量。
import os
获取环境变量
home_dir = os.environ.get('HOME')
print(f'Home directory: {home_dir}')
设置环境变量
os.environ['MY_VAR'] = 'my_value'
print(f'MY_VAR: {os.environ.get("MY_VAR")}')
3、操作文件系统
os模块还提供了许多与文件系统交互的函数,如os.path、os.mkdir、os.rename等。
import os
创建目录
os.mkdir('my_directory')
重命名文件
os.rename('old_name.txt', 'new_name.txt')
删除文件
os.remove('new_name.txt')
二、使用subprocess模块
subprocess模块是Python 3中推荐用于执行系统命令的模块。它提供了更强大的功能,如捕获命令输出、处理错误信息等。
1、执行系统命令并捕获输出
使用subprocess.run()函数可以执行系统命令并捕获输出。我们可以通过传递参数来控制命令的执行方式。
import subprocess
执行ls命令并捕获输出
result = subprocess.run(['ls', '-la'], capture_output=True, text=True)
print(result.stdout)
2、处理错误信息
subprocess.run()函数可以通过check参数来捕获命令的错误信息。
import subprocess
try:
# 执行一个不存在的命令
result = subprocess.run(['nonexistent_command'], check=True, capture_output=True, text=True)
except subprocess.CalledProcessError as e:
print(f'Error: {e}')
3、管道和重定向
subprocess模块还支持管道和重定向操作。我们可以使用stdin、stdout和stderr参数来实现这些功能。
import subprocess
执行命令并将输出重定向到文件
with open('output.txt', 'w') as f:
subprocess.run(['ls', '-la'], stdout=f)
使用管道将一个命令的输出作为另一个命令的输入
p1 = subprocess.Popen(['ls', '-la'], stdout=subprocess.PIPE)
p2 = subprocess.Popen(['grep', 'my_file'], stdin=p1.stdout, stdout=subprocess.PIPE)
p1.stdout.close()
output = p2.communicate()[0]
print(output.decode('utf-8'))
三、提供更好的错误处理和调试支持
Python 提供了丰富的异常处理和调试工具,使我们能够更好地处理错误和调试程序。
1、异常处理
我们可以使用try-except块来捕获和处理异常,确保程序在出现错误时不会崩溃。
try:
# 打开一个不存在的文件
with open('nonexistent_file.txt', 'r') as f:
content = f.read()
except FileNotFoundError as e:
print(f'Error: {e}')
2、日志记录
Python 的logging模块允许我们记录程序的运行信息,以便调试和分析。
import logging
配置日志记录
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
记录信息
logging.info('This is an info message')
logging.warning('This is a warning message')
logging.error('This is an error message')
3、调试工具
Python 提供了多种调试工具,如pdb、ipdb等,可以帮助我们逐步调试程序。
import pdb
def my_function():
a = 1
b = 2
pdb.set_trace() # 设置断点
c = a + b
print(c)
my_function()
四、综合示例
下面是一个综合示例,展示了如何使用Python3替换Shell脚本,实现一个简单的系统管理任务。
import os
import subprocess
import logging
配置日志记录
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def main():
try:
# 获取环境变量
home_dir = os.environ.get('HOME')
logging.info(f'Home directory: {home_dir}')
# 创建目录
new_dir = os.path.join(home_dir, 'my_directory')
os.mkdir(new_dir)
logging.info(f'Created directory: {new_dir}')
# 执行系统命令并捕获输出
result = subprocess.run(['ls', '-la', new_dir], capture_output=True, text=True)
logging.info(f'Directory contents:\n{result.stdout}')
# 重命名文件
old_file = os.path.join(new_dir, 'old_name.txt')
new_file = os.path.join(new_dir, 'new_name.txt')
with open(old_file, 'w') as f:
f.write('Hello, world!')
os.rename(old_file, new_file)
logging.info(f'Renamed file: {old_file} to {new_file}')
# 使用管道将一个命令的输出作为另一个命令的输入
p1 = subprocess.Popen(['ls', '-la', new_file], stdout=subprocess.PIPE)
p2 = subprocess.Popen(['grep', 'new_name'], stdin=p1.stdout, stdout=subprocess.PIPE)
p1.stdout.close()
output = p2.communicate()[0]
logging.info(f'Grep output:\n{output.decode("utf-8")}')
except Exception as e:
logging.error(f'Error: {e}')
if __name__ == '__main__':
main()
这个示例展示了如何使用Python3替换Shell脚本,实现一个简单的系统管理任务,包括获取环境变量、创建目录、执行系统命令、重命名文件和使用管道等操作。通过这种方式,我们可以充分利用Python的强大功能,实现更加复杂和高效的系统管理任务。
相关问答FAQs:
如何用Python3实现与Shell相似的功能?
Python3可以通过内置的os
和subprocess
模块实现许多Shell的功能,如文件操作、命令执行和进程管理。使用subprocess
模块,你可以轻松地执行系统命令并获取输出,类似于在Shell中运行命令。例如,subprocess.run()
可以执行命令并返回结果,同时支持输入输出重定向。
在Python3中如何执行Shell命令并处理输出?
使用subprocess
模块的run()
函数,可以直接在Python中执行Shell命令。通过设置参数,您可以捕获标准输出和错误信息。例如,result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
将执行ls -l
命令,并将输出存储在result.stdout
中,方便后续处理。
Python3是否能替代Shell脚本用于自动化任务?
是的,Python3非常适合用于自动化任务。与Shell脚本相比,Python的语法更为清晰,功能更为强大。您可以利用Python的丰富库进行文件处理、网络请求、数据库操作等多种任务,使得自动化脚本更加灵活和易于维护。同时,Python的异常处理机制也使得错误处理更加简洁。
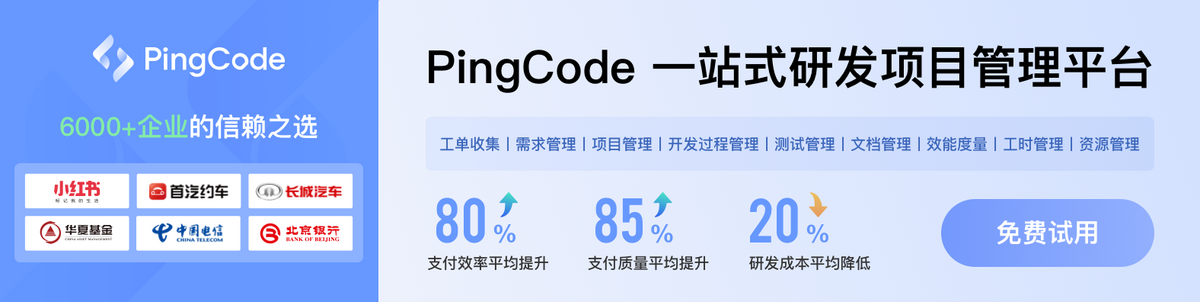