Python开发写配置文件的方式有多种,可以使用JSON、YAML、ini文件等,最常见的方法包括使用内置的configparser模块、json模块和第三方的PyYAML库。其中,使用configparser模块是最常见和方便的方式,因为其语法简单易懂,适合初学者和大多数应用场景。
以下是详细描述如何使用configparser模块进行配置文件的编写和读取:
一、使用ConfigParser进行配置文件的编写和读取
1、编写配置文件
首先,创建一个配置文件,例如 config.ini
,格式如下:
[DEFAULT]
ServerAliveInterval = 45
Compression = yes
CompressionLevel = 9
ForwardX11 = yes
[bitbucket.org]
User = hg
[topsecret.server.com]
Port = 50022
ForwardX11 = no
2、读取配置文件
接下来,使用ConfigParser模块读取这个配置文件:
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
读取默认配置
server_alive_interval = config['DEFAULT']['ServerAliveInterval']
compression = config['DEFAULT']['Compression']
compression_level = config['DEFAULT'].getint('CompressionLevel')
读取特定部分的配置
user = config['bitbucket.org']['User']
port = config['topsecret.server.com'].getint('Port')
forward_x11 = config['topsecret.server.com'].getboolean('ForwardX11')
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"CompressionLevel: {compression_level}")
print(f"User: {user}")
print(f"Port: {port}")
print(f"ForwardX11: {forward_x11}")
3、写入配置文件
如果需要动态生成或修改配置文件,也可以使用ConfigParser模块来写入配置:
import configparser
config = configparser.ConfigParser()
config['DEFAULT'] = {
'ServerAliveInterval': '45',
'Compression': 'yes',
'CompressionLevel': '9',
'ForwardX11': 'yes'
}
config['bitbucket.org'] = {'User': 'hg'}
config['topsecret.server.com'] = {
'Port': '50022',
'ForwardX11': 'no'
}
with open('config.ini', 'w') as configfile:
config.write(configfile)
二、使用JSON进行配置文件的编写和读取
1、编写配置文件
首先,创建一个配置文件,例如 config.json
,格式如下:
{
"ServerAliveInterval": 45,
"Compression": true,
"CompressionLevel": 9,
"ForwardX11": true,
"bitbucket.org": {
"User": "hg"
},
"topsecret.server.com": {
"Port": 50022,
"ForwardX11": false
}
}
2、读取配置文件
接下来,使用json模块读取这个配置文件:
import json
with open('config.json', 'r') as configfile:
config = json.load(configfile)
server_alive_interval = config['ServerAliveInterval']
compression = config['Compression']
compression_level = config['CompressionLevel']
user = config['bitbucket.org']['User']
port = config['topsecret.server.com']['Port']
forward_x11 = config['topsecret.server.com']['ForwardX11']
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"CompressionLevel: {compression_level}")
print(f"User: {user}")
print(f"Port: {port}")
print(f"ForwardX11: {forward_x11}")
3、写入配置文件
如果需要动态生成或修改配置文件,也可以使用json模块来写入配置:
import json
config = {
"ServerAliveInterval": 45,
"Compression": True,
"CompressionLevel": 9,
"ForwardX11": True,
"bitbucket.org": {
"User": "hg"
},
"topsecret.server.com": {
"Port": 50022,
"ForwardX11": False
}
}
with open('config.json', 'w') as configfile:
json.dump(config, configfile, indent=4)
三、使用YAML进行配置文件的编写和读取
1、编写配置文件
首先,创建一个配置文件,例如 config.yaml
,格式如下:
ServerAliveInterval: 45
Compression: true
CompressionLevel: 9
ForwardX11: true
bitbucket.org:
User: hg
topsecret.server.com:
Port: 50022
ForwardX11: false
2、读取配置文件
接下来,使用PyYAML库读取这个配置文件:
import yaml
with open('config.yaml', 'r') as configfile:
config = yaml.safe_load(configfile)
server_alive_interval = config['ServerAliveInterval']
compression = config['Compression']
compression_level = config['CompressionLevel']
user = config['bitbucket.org']['User']
port = config['topsecret.server.com']['Port']
forward_x11 = config['topsecret.server.com']['ForwardX11']
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"CompressionLevel: {compression_level}")
print(f"User: {user}")
print(f"Port: {port}")
print(f"ForwardX11: {forward_x11}")
3、写入配置文件
如果需要动态生成或修改配置文件,也可以使用PyYAML库来写入配置:
import yaml
config = {
'ServerAliveInterval': 45,
'Compression': True,
'CompressionLevel': 9,
'ForwardX11': True,
'bitbucket.org': {
'User': 'hg'
},
'topsecret.server.com': {
'Port': 50022,
'ForwardX11': False
}
}
with open('config.yaml', 'w') as configfile:
yaml.dump(config, configfile, default_flow_style=False)
四、总结
通过使用ConfigParser、json模块和PyYAML库,Python开发者可以轻松地编写和读取配置文件。ConfigParser适用于简单的配置需求,json模块适用于结构化数据,而PyYAML库则适用于更复杂的配置需求。选择合适的工具和格式,可以提高代码的可维护性和可读性。
相关问答FAQs:
如何在Python中使用配置文件来管理应用程序设置?
在Python中,使用配置文件可以有效地管理应用程序的设置和参数,减少硬编码的需要。最常见的配置文件格式包括INI、JSON和YAML。Python标准库提供了configparser
模块来处理INI格式的配置文件,使用json
模块可以处理JSON文件,而PyYAML
库则用于读取和写入YAML格式的文件。用户可以选择适合自己项目需求的格式,并通过相应的库来加载和解析配置内容。
Python配置文件的最佳实践有哪些?
在编写Python配置文件时,遵循一些最佳实践可以提高代码的可维护性和可读性。首先,确保配置文件与代码分离,这样可以方便地进行修改而不需要更改代码。其次,使用明确的命名方式来标识配置项,避免使用模糊的名称。此外,提供适当的注释,帮助其他开发者理解各个配置项的含义和用途。最后,考虑使用默认值和环境变量来增强灵活性,使得配置可以适应不同的运行环境。
如何在Python中读取和写入不同格式的配置文件?
读取和写入配置文件的方法取决于文件的格式。对于INI格式,可以使用configparser
模块的ConfigParser
类来读取和写入。例如,可以使用config.read('config.ini')
读取文件,使用config.write(open('config.ini', 'w'))
保存修改。对于JSON格式,使用json.load()
和json.dump()
方法分别读取和保存数据。而对于YAML格式,则可以使用yaml.safe_load()
和yaml.dump()
方法。确保在使用前安装相应的库,并根据项目需求选择合适的格式。
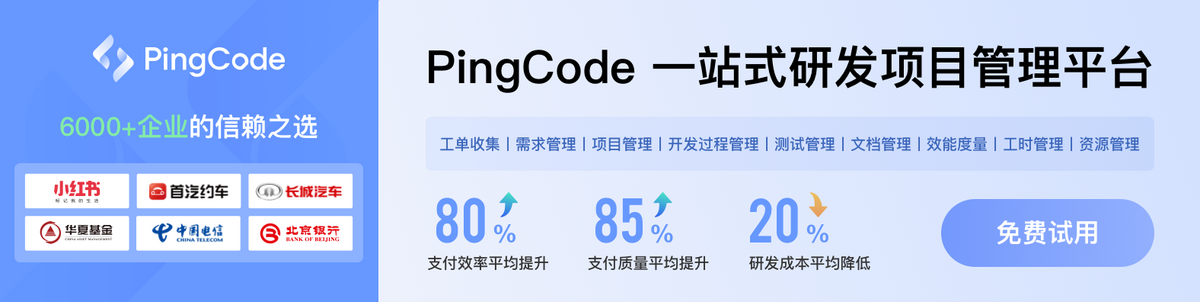