如何用Python模拟原神抽卡
使用Python模拟原神抽卡,可以通过编写一个程序来随机生成抽卡结果、模拟抽卡概率、设计抽卡保底机制、构建一个抽卡界面。 其中,模拟抽卡概率是最为关键的一步,可以通过设置不同稀有度的掉落率来实现。本文将详细介绍如何用Python编写一个完整的原神抽卡模拟程序,包括各个步骤的实现和代码示例。
一、创建抽卡池和概率
在原神中,抽卡池分为角色池和武器池,每个池子有不同的掉落率和稀有度。我们需要首先定义这些抽卡池的内容和概率。以下是一个简单的抽卡池定义:
import random
定义稀有度和掉落率
rarity = {
"5星": 0.006,
"4星": 0.051,
"3星": 0.943
}
定义卡池
character_pool = {
"5星": ["角色A", "角色B", "角色C"],
"4星": ["角色D", "角色E", "角色F"],
"3星": ["角色G", "角色H", "角色I"]
}
weapon_pool = {
"5星": ["武器A", "武器B", "武器C"],
"4星": ["武器D", "武器E", "武器F"],
"3星": ["武器G", "武器H", "武器I"]
}
二、实现抽卡功能
接下来,我们需要编写一个函数来实现抽卡功能,该函数将根据定义的掉落率随机生成一个结果。
def draw(pool):
prob = random.random()
cumulative_prob = 0.0
for rarity, rate in rarity.items():
cumulative_prob += rate
if prob < cumulative_prob:
return random.choice(pool[rarity])
return None
示例使用
result = draw(character_pool)
print(f"抽到的结果是: {result}")
三、设计保底机制
原神的抽卡机制中有一个保底机制,即在一定次数内没有抽到高稀有度的角色或武器时,系统会保证一定会抽到。我们可以通过增加计数器来实现这一功能。
class GachaSimulator:
def __init__(self, pool, pity=90, soft_pity=75):
self.pool = pool
self.pity = pity
self.soft_pity = soft_pity
self.counter = 0
def draw(self):
self.counter += 1
# 保底机制
if self.counter >= self.pity:
self.counter = 0
return random.choice(self.pool["5星"])
# 软保底机制
if self.counter >= self.soft_pity:
prob = random.random()
if prob < (self.counter - self.soft_pity + 1) * 0.06:
self.counter = 0
return random.choice(self.pool["5星"])
# 正常抽卡
return draw(self.pool)
示例使用
simulator = GachaSimulator(character_pool)
for _ in range(10):
result = simulator.draw()
print(f"抽到的结果是: {result}")
四、创建用户界面
为了使抽卡模拟更加直观,我们可以使用Python的GUI库,如Tkinter,来创建一个简单的用户界面。
import tkinter as tk
from tkinter import messagebox
class GachaApp:
def __init__(self, root):
self.simulator = GachaSimulator(character_pool)
self.root = root
self.root.title("原神抽卡模拟器")
self.label = tk.Label(root, text="点击按钮抽卡")
self.label.pack()
self.button = tk.Button(root, text="抽卡", command=self.draw)
self.button.pack()
def draw(self):
result = self.simulator.draw()
messagebox.showinfo("抽卡结果", f"抽到的结果是: {result}")
创建GUI应用
root = tk.Tk()
app = GachaApp(root)
root.mainloop()
五、优化和扩展
以上代码已经实现了一个基本的原神抽卡模拟程序,但我们可以进一步优化和扩展它。例如,可以增加更多的卡池、增加更多的稀有度、实现更多的保底机制、记录抽卡历史等。
- 增加更多的卡池
如果需要模拟不同的卡池,可以在程序中增加选择卡池的功能。
class GachaApp:
def __init__(self, root):
self.character_simulator = GachaSimulator(character_pool)
self.weapon_simulator = GachaSimulator(weapon_pool)
self.root = root
self.root.title("原神抽卡模拟器")
self.label = tk.Label(root, text="选择卡池并点击按钮抽卡")
self.label.pack()
self.character_button = tk.Button(root, text="角色池", command=self.draw_character)
self.character_button.pack()
self.weapon_button = tk.Button(root, text="武器池", command=self.draw_weapon)
self.weapon_button.pack()
def draw_character(self):
result = self.character_simulator.draw()
messagebox.showinfo("抽卡结果", f"抽到的结果是: {result}")
def draw_weapon(self):
result = self.weapon_simulator.draw()
messagebox.showinfo("抽卡结果", f"抽到的结果是: {result}")
创建GUI应用
root = tk.Tk()
app = GachaApp(root)
root.mainloop()
- 增加更多的稀有度
如果需要增加更多的稀有度,可以在定义卡池和概率时添加新的稀有度级别。
rarity = {
"6星": 0.001,
"5星": 0.005,
"4星": 0.05,
"3星": 0.944
}
character_pool = {
"6星": ["角色S"],
"5星": ["角色A", "角色B", "角色C"],
"4星": ["角色D", "角色E", "角色F"],
"3星": ["角色G", "角色H", "角色I"]
}
- 记录抽卡历史
如果需要记录抽卡历史,可以在抽卡类中添加一个列表来存储每次抽卡的结果。
class GachaSimulator:
def __init__(self, pool, pity=90, soft_pity=75):
self.pool = pool
self.pity = pity
self.soft_pity = soft_pity
self.counter = 0
self.history = []
def draw(self):
self.counter += 1
# 保底机制
if self.counter >= self.pity:
self.counter = 0
result = random.choice(self.pool["5星"])
self.history.append(result)
return result
# 软保底机制
if self.counter >= self.soft_pity:
prob = random.random()
if prob < (self.counter - self.soft_pity + 1) * 0.06:
self.counter = 0
result = random.choice(self.pool["5星"])
self.history.append(result)
return result
# 正常抽卡
result = draw(self.pool)
self.history.append(result)
return result
示例使用
simulator = GachaSimulator(character_pool)
for _ in range(10):
result = simulator.draw()
print(f"抽到的结果是: {result}")
print("抽卡历史:", simulator.history)
通过以上步骤,我们已经详细介绍了如何用Python模拟原神抽卡。希望通过这些代码示例和详细说明,能够帮助你更好地理解和实现抽卡模拟程序。
相关问答FAQs:
如何在Python中实现抽卡的随机性?
在原神的抽卡系统中,随机性是关键。可以使用Python的random
模块来模拟这一过程。具体方法是设定一个权重分布,代表不同稀有度的角色和武器的获得概率。通过生成随机数并与权重进行比较,可以决定抽到的物品。例如,对于一个5星角色的抽取,可以设定1%概率,4星角色则为10%。通过多次抽卡,可以观察到不同角色的获得情况,从而优化模拟过程。
如何设置原神抽卡的权重和概率?
在模拟原神抽卡时,首先需要定义各个角色和武器的稀有度及其对应的概率。一般来说,5星角色的获得概率较低,通常为0.6%-1.0%;4星角色的概率在5%-10%之间,而3星角色的概率则更高。可以创建一个字典,将每种角色或武器的名称与其获得概率关联,然后在抽卡时利用这些概率生成相应的随机数,决定抽取的结果。
如何记录抽卡结果并进行统计分析?
在模拟抽卡后,为了更好地理解抽卡的结果,可以将每次抽卡的结果记录下来。可以使用一个列表来存储每次抽到的角色或武器名称,并在抽卡结束后,使用collections.Counter
来统计各个角色或武器的出现次数。这不仅能够显示抽卡的运气,还能帮助分析不同角色的获得频率,从而为后续的抽卡策略提供数据支持。
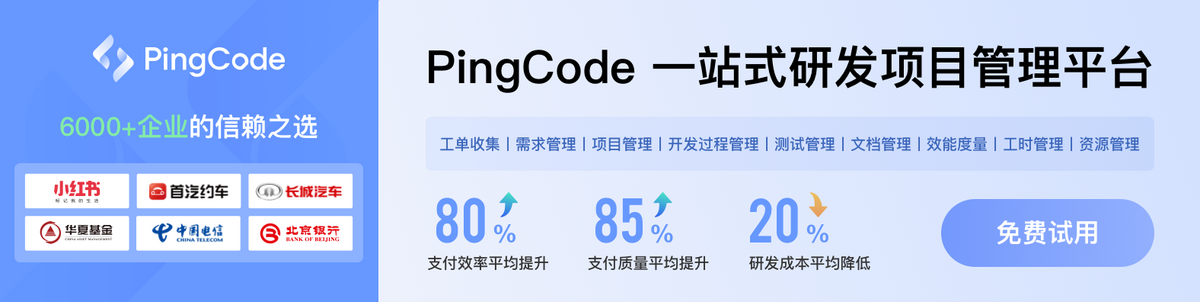