要补充猜数Python代码形成函数,可以将核心猜数逻辑封装成一个函数、增加输入参数、返回值等,并提供示例调用方法。 在本篇文章中,我们将详细讲解如何将一个简单的猜数游戏代码转变为一个可复用的函数,并逐步介绍代码的各个部分及其功能。
一、什么是猜数游戏
猜数游戏是一种经典的编程练习,通常用于帮助初学者理解基本的编程概念,如循环、条件判断、用户输入和随机数生成。在这个游戏中,计算机会随机生成一个数字,然后用户需要根据提示猜测这个数字。
二、补充猜数代码形成函数的步骤
- 定义函数:将核心逻辑封装成函数。
- 添加参数:使函数更通用。
- 增加返回值:提供函数执行结果。
- 示例调用:展示如何使用该函数。
1、定义函数
首先,我们需要将猜数游戏的核心逻辑封装成一个函数。这样做的好处是可以提高代码的可重用性和可维护性。以下是一个简单的猜数游戏代码:
import random
def guess_number():
number_to_guess = random.randint(1, 100)
guess = None
while guess != number_to_guess:
guess = int(input("Guess the number between 1 and 100: "))
if guess < number_to_guess:
print("Too low!")
elif guess > number_to_guess:
print("Too high!")
else:
print("Congratulations! You've guessed the number!")
2、添加参数
为了使函数更加通用,我们可以添加一些参数,例如猜测范围和最大尝试次数。
import random
def guess_number(min_value=1, max_value=100, max_attempts=10):
number_to_guess = random.randint(min_value, max_value)
attempts = 0
guess = None
while guess != number_to_guess and attempts < max_attempts:
guess = int(input(f"Guess the number between {min_value} and {max_value}: "))
attempts += 1
if guess < number_to_guess:
print("Too low!")
elif guess > number_to_guess:
print("Too high!")
else:
print("Congratulations! You've guessed the number!")
if guess != number_to_guess:
print(f"Sorry, you've used all {max_attempts} attempts. The number was {number_to_guess}.")
3、增加返回值
为了让函数不仅仅是打印结果,我们可以增加返回值,例如返回用户是否猜对了数字以及猜测次数。
import random
def guess_number(min_value=1, max_value=100, max_attempts=10):
number_to_guess = random.randint(min_value, max_value)
attempts = 0
guess = None
while guess != number_to_guess and attempts < max_attempts:
guess = int(input(f"Guess the number between {min_value} and {max_value}: "))
attempts += 1
if guess < number_to_guess:
print("Too low!")
elif guess > number_to_guess:
print("Too high!")
else:
print("Congratulations! You've guessed the number!")
if guess != number_to_guess:
print(f"Sorry, you've used all {max_attempts} attempts. The number was {number_to_guess}.")
return False, attempts
return True, attempts
4、示例调用
最后,我们展示如何调用这个函数,并处理其返回值。
if __name__ == "__main__":
success, attempts = guess_number(min_value=1, max_value=100, max_attempts=5)
if success:
print(f"You've guessed the number in {attempts} attempts!")
else:
print(f"Better luck next time! You used {attempts} attempts.")
三、进一步优化
1、添加用户输入验证
当前函数中,用户输入并没有进行验证,若用户输入非整数,程序会抛出异常。我们可以添加输入验证来提高程序的鲁棒性。
import random
def guess_number(min_value=1, max_value=100, max_attempts=10):
number_to_guess = random.randint(min_value, max_value)
attempts = 0
guess = None
while guess != number_to_guess and attempts < max_attempts:
try:
guess = int(input(f"Guess the number between {min_value} and {max_value}: "))
except ValueError:
print("Invalid input! Please enter a valid integer.")
continue
attempts += 1
if guess < number_to_guess:
print("Too low!")
elif guess > number_to_guess:
print("Too high!")
else:
print("Congratulations! You've guessed the number!")
if guess != number_to_guess:
print(f"Sorry, you've used all {max_attempts} attempts. The number was {number_to_guess}.")
return False, attempts
return True, attempts
2、允许用户选择继续游戏
我们可以让用户在游戏结束后选择是否继续玩游戏。
import random
def guess_number(min_value=1, max_value=100, max_attempts=10):
number_to_guess = random.randint(min_value, max_value)
attempts = 0
guess = None
while guess != number_to_guess and attempts < max_attempts:
try:
guess = int(input(f"Guess the number between {min_value} and {max_value}: "))
except ValueError:
print("Invalid input! Please enter a valid integer.")
continue
attempts += 1
if guess < number_to_guess:
print("Too low!")
elif guess > number_to_guess:
print("Too high!")
else:
print("Congratulations! You've guessed the number!")
if guess != number_to_guess:
print(f"Sorry, you've used all {max_attempts} attempts. The number was {number_to_guess}.")
return False, attempts
return True, attempts
if __name__ == "__main__":
while True:
success, attempts = guess_number(min_value=1, max_value=100, max_attempts=5)
if success:
print(f"You've guessed the number in {attempts} attempts!")
else:
print(f"Better luck next time! You used {attempts} attempts.")
play_again = input("Do you want to play again? (yes/no): ").lower()
if play_again != 'yes':
break
四、总结
通过本文的讲解,我们成功地将一个简单的猜数游戏代码封装成了一个函数,并且逐步添加了参数、返回值、输入验证和继续游戏选项。这不仅提高了代码的可重用性和可维护性,还让代码变得更加健壮和用户友好。希望通过这篇文章,您能更好地理解如何将一个简单的Python脚本转变为一个功能齐全的函数,并在实际编程中灵活运用这些技巧。
相关问答FAQs:
如何在Python中实现一个数值猜测游戏的函数?
可以通过定义一个函数来实现猜数游戏,该函数可以接收一个参数来设置数字范围。在函数内部,使用随机数生成器随机选择一个数字,并通过循环提示用户输入猜测,直到用户猜对为止。可以使用input()
函数获取用户的输入,并利用条件判断给出提示。
如何提高猜数游戏的用户体验?
可以通过提供更多的反馈来提升用户体验。例如,告知用户猜测的数值是高于还是低于目标数字。此外,可以增加猜测次数的限制,给出用户在游戏中的得分或时间限制,以增加挑战性和趣味性。
如何在猜数游戏中添加难度选择功能?
可以在函数中添加一个难度选择的参数,允许用户选择简单、中等或困难的模式。根据不同的难度,设置不同的数字范围或限制猜测次数。例如,简单模式可以选择1到10的范围,而困难模式可以选择1到100的范围。通过这种方式,用户可以根据自己的喜好选择合适的挑战。
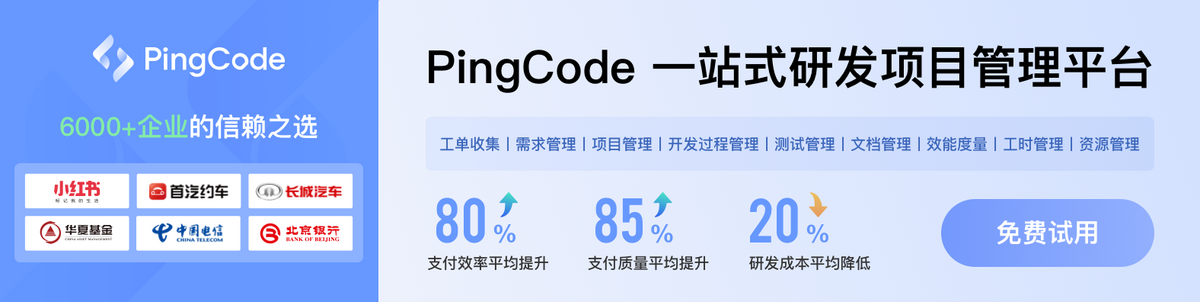