如何用Python建立考试试题库
使用Python建立考试试题库可以帮助教育工作者和学生更高效地管理和使用题库。使用Python构建考试试题库的步骤包括:定义题库数据结构、选择题库存储方式、创建和管理题目、生成试卷等。其中,选择合适的题库存储方式尤为重要。常见的存储方式有CSV文件、数据库(如SQLite、MySQL)和JSON文件等。下面我们将详细介绍如何使用Python创建一个考试试题库。
一、定义题库数据结构
在构建考试试题库之前,首先需要定义题库的数据结构。这包括题目的类型、题目内容、选项、正确答案和其他相关信息。常见的题目类型有选择题、判断题、填空题和简答题等。以下是一个题目数据结构的示例:
class Question:
def __init__(self, question_id, question_type, content, options, answer):
self.question_id = question_id
self.question_type = question_type
self.content = content
self.options = options
self.answer = answer
在这个数据结构中,question_id
是题目的唯一标识,question_type
表示题目的类型(如选择题、判断题等),content
是题目的内容,options
是选择题的选项(对于非选择题,这一项可以为空),answer
是正确答案。
二、选择题库存储方式
题库的存储方式有多种选择,包括CSV文件、数据库和JSON文件等。不同的存储方式有不同的优缺点。
- CSV文件
CSV文件是一种简单的文本文件格式,适合存储结构化数据。使用CSV文件存储题库数据的优点是简单易用,缺点是对于复杂的数据结构支持较差。
import csv
def save_to_csv(questions, filename):
with open(filename, mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['question_id', 'question_type', 'content', 'options', 'answer'])
for question in questions:
writer.writerow([question.question_id, question.question_type, question.content, ','.join(question.options), question.answer])
def load_from_csv(filename):
questions = []
with open(filename, mode='r') as file:
reader = csv.reader(file)
next(reader) # Skip header row
for row in reader:
question_id, question_type, content, options, answer = row
options = options.split(',')
questions.append(Question(question_id, question_type, content, options, answer))
return questions
- 数据库(如SQLite、MySQL)
使用数据库存储题库数据的优点是可以处理大量数据,支持复杂的查询操作。缺点是需要安装和配置数据库。
import sqlite3
def create_database(db_name):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('''
CREATE TABLE IF NOT EXISTS questions (
question_id INTEGER PRIMARY KEY,
question_type TEXT,
content TEXT,
options TEXT,
answer TEXT
)
''')
conn.commit()
conn.close()
def save_to_database(questions, db_name):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
for question in questions:
cursor.execute('''
INSERT INTO questions (question_id, question_type, content, options, answer)
VALUES (?, ?, ?, ?, ?)
''', (question.question_id, question.question_type, question.content, ','.join(question.options), question.answer))
conn.commit()
conn.close()
def load_from_database(db_name):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('SELECT * FROM questions')
rows = cursor.fetchall()
questions = []
for row in rows:
question_id, question_type, content, options, answer = row
options = options.split(',')
questions.append(Question(question_id, question_type, content, options, answer))
conn.close()
return questions
- JSON文件
JSON文件是一种轻量级的数据交换格式,适合存储结构化数据。使用JSON文件存储题库数据的优点是易于读写和解析,缺点是对于非常大的数据集支持较差。
import json
def save_to_json(questions, filename):
data = []
for question in questions:
data.append({
'question_id': question.question_id,
'question_type': question.question_type,
'content': question.content,
'options': question.options,
'answer': question.answer
})
with open(filename, 'w') as file:
json.dump(data, file)
def load_from_json(filename):
with open(filename, 'r') as file:
data = json.load(file)
questions = []
for item in data:
questions.append(Question(item['question_id'], item['question_type'], item['content'], item['options'], item['answer']))
return questions
三、创建和管理题目
在定义了题库的数据结构并选择了存储方式之后,接下来就是创建和管理题目。可以通过编写Python代码来实现题目的添加、删除、修改和查询等操作。
def add_question(questions, question):
questions.append(question)
def delete_question(questions, question_id):
questions = [q for q in questions if q.question_id != question_id]
return questions
def update_question(questions, question_id, new_question):
for i, question in enumerate(questions):
if question.question_id == question_id:
questions[i] = new_question
break
def find_question(questions, question_id):
for question in questions:
if question.question_id == question_id:
return question
return None
四、生成试卷
通过从题库中随机抽取题目,可以生成一份试卷。可以根据需要指定生成的试卷包含的题目数量和类型。
import random
def generate_exam(questions, num_questions):
return random.sample(questions, num_questions)
五、综合示例
下面是一个综合示例,演示了如何使用上述代码构建一个简单的考试试题库,并生成一份试卷。
# 定义题目
questions = [
Question(1, '选择题', 'Python是什么语言?', ['编程语言', '操作系统', '数据库', '网络协议'], '编程语言'),
Question(2, '判断题', 'Python是动态类型语言。', [], '正确'),
Question(3, '填空题', 'Python的创始人是____。', [], 'Guido van Rossum'),
Question(4, '简答题', '请简述Python的特点。', [], '易读性强,简洁,支持多种编程范式等')
]
保存题库到CSV文件
save_to_csv(questions, 'questions.csv')
从CSV文件加载题库
loaded_questions = load_from_csv('questions.csv')
添加新题目
new_question = Question(5, '选择题', 'Python的最新版本是?', ['3.8', '3.9', '3.10', '3.11'], '3.11')
add_question(loaded_questions, new_question)
删除题目
loaded_questions = delete_question(loaded_questions, 2)
更新题目
updated_question = Question(1, '选择题', 'Python是一种什么语言?', ['编程语言', '操作系统', '数据库', '网络协议'], '编程语言')
update_question(loaded_questions, 1, updated_question)
查找题目
question = find_question(loaded_questions, 3)
print(question.content)
生成试卷
exam = generate_exam(loaded_questions, 3)
for q in exam:
print(q.content)
综上所述,使用Python构建考试试题库的步骤包括定义题库数据结构、选择题库存储方式、创建和管理题目、生成试卷等。通过合理的数据结构设计和存储方式选择,可以实现题库的高效管理和使用。希望这篇文章能帮助你更好地理解和实现Python考试试题库的构建。
相关问答FAQs:
1. 如何创建一个简单的Python试题库?
要创建一个简单的Python试题库,您可以使用字典或列表来存储试题和答案。首先定义试题的结构,例如每个试题可以包含题目、选项和正确答案。接着,编写函数来添加新试题、查询试题以及检查用户的答案。利用CSV或JSON格式,可以轻松地将试题保存到文件中,以便后续使用。
2. Python试题库可以实现哪些功能?
一个完整的Python试题库可以实现多种功能,包括但不限于:随机抽取试题、计时考试、自动评分、生成考试报告和导出试题为文件等。此外,您还可以添加用户管理功能,以便不同用户可以访问不同的试题集,满足不同需求。
3. 如何提高Python试题库的用户体验?
为了提升用户体验,可以考虑以下几点:设计友好的用户界面,使用图形界面库如Tkinter或Flask来创建网页应用;提供多种题型,如选择题、填空题和简答题;加入提示和解析功能,以帮助用户理解错误的原因;并提供成绩分析,帮助用户找到自己的薄弱环节。这些都能使试题库更加吸引人和实用。
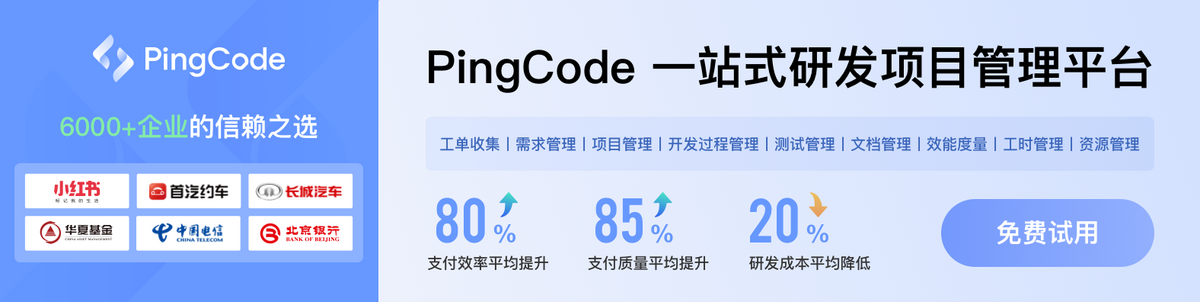