如何用Python写一首诗,利用Python的随机生成功能、预定义词库、文本处理库(如NLTK)
使用Python写一首诗,可以通过以下几个步骤来实现:利用Python的随机生成功能、预定义词库、文本处理库(如NLTK)。其中,利用Python的随机生成功能是最为关键的一步,通过随机选择单词和短语,可以生成独特且富有创意的诗句。详细描述如下:
利用Python的随机生成功能,可以通过调用随机模块中的函数,如random.choice()
,从预定义的单词或短语列表中随机选择元素,组合成诗句。这种方法能够确保每次生成的诗句都是独一无二的。下面我们来详细介绍如何实现这一过程。
一、准备工作
在开始写诗之前,我们需要做一些准备工作,包括安装必要的库、创建词库等。
1、安装所需库
首先,确保你安装了Python和所需的库。可以使用pip来安装这些库:
pip install nltk
pip install numpy
2、创建词库
我们需要创建一个词库,包含不同类型的词汇,如名词、动词、形容词等。可以手动创建一个词库,也可以使用现有的词库,如NLTK中的词库。
二、随机生成诗句
接下来,我们将使用Python的随机生成功能,通过从词库中随机选择单词来生成诗句。
1、导入必要的库
首先,导入我们将要使用的库:
import random
import nltk
from nltk.corpus import wordnet as wn
nltk.download('wordnet')
2、定义词库
我们可以使用NLTK中的WordNet来获取不同类型的词汇,或者手动定义一个词库:
nouns = [synset.name().split('.')[0] for synset in wn.all_synsets('n')]
verbs = [synset.name().split('.')[0] for synset in wn.all_synsets('v')]
adjectives = [synset.name().split('.')[0] for synset in wn.all_synsets('a')]
3、生成诗句
使用随机选择函数,从词库中选择单词并组合成诗句:
def generate_line():
noun = random.choice(nouns)
verb = random.choice(verbs)
adjective = random.choice(adjectives)
line = f"The {adjective} {noun} {verb}s"
return line
def generate_poem(lines=4):
poem = "\n".join(generate_line() for _ in range(lines))
return poem
print(generate_poem())
三、优化生成的诗句
为了生成更加自然和优美的诗句,我们可以进一步优化生成的过程。
1、使用模板
使用预定义的模板,可以生成更加结构化的诗句:
templates = [
"The {adjective} {noun} {verb}s in the {noun}",
"A {noun} {verb}s with {adjective} grace",
"In the {noun}, a {adjective} {noun} {verb}s",
]
def generate_line():
template = random.choice(templates)
noun1 = random.choice(nouns)
noun2 = random.choice(nouns)
verb = random.choice(verbs)
adjective = random.choice(adjectives)
line = template.format(noun=noun1, verb=verb, adjective=adjective, noun2=noun2)
return line
2、引入韵律
可以通过引入韵律,使得诗句更加具有音乐性和美感。例如,我们可以使用NLTK的CMU Pronouncing Dictionary来实现韵律匹配。
from nltk.corpus import cmudict
nltk.download('cmudict')
prondict = cmudict.dict()
def get_rhyme(word):
if word in prondict:
return prondict[word][0][-1]
return None
def generate_rhyming_lines():
line1 = generate_line()
rhyme1 = get_rhyme(line1.split()[-1])
while True:
line2 = generate_line()
if get_rhyme(line2.split()[-1]) == rhyme1:
break
return line1, line2
def generate_poem(lines=4):
poem = []
for _ in range(lines // 2):
line1, line2 = generate_rhyming_lines()
poem.append(line1)
poem.append(line2)
return "\n".join(poem)
print(generate_poem())
四、添加语义关联
为了使生成的诗句在语义上更加连贯,我们可以引入语义关联。例如,可以使用WordNet的同义词和反义词。
def get_related_words(word, pos):
synsets = wn.synsets(word, pos=pos)
related_words = set()
for synset in synsets:
for lemma in synset.lemmas():
related_words.add(lemma.name())
if lemma.antonyms():
related_words.add(lemma.antonyms()[0].name())
return list(related_words)
def generate_semantic_line():
noun = random.choice(nouns)
related_adjectives = get_related_words(noun, wn.NOUN)
if related_adjectives:
adjective = random.choice(related_adjectives)
else:
adjective = random.choice(adjectives)
verb = random.choice(verbs)
line = f"The {adjective} {noun} {verb}s"
return line
五、综合实现
将上述所有优化综合起来,实现一个完整的诗句生成程序:
import random
import nltk
from nltk.corpus import wordnet as wn
from nltk.corpus import cmudict
nltk.download('wordnet')
nltk.download('cmudict')
nouns = [synset.name().split('.')[0] for synset in wn.all_synsets('n')]
verbs = [synset.name().split('.')[0] for synset in wn.all_synsets('v')]
adjectives = [synset.name().split('.')[0] for synset in wn.all_synsets('a')]
templates = [
"The {adjective} {noun} {verb}s in the {noun2}",
"A {noun} {verb}s with {adjective} grace",
"In the {noun}, a {adjective} {noun2} {verb}s",
]
prondict = cmudict.dict()
def get_rhyme(word):
if word in prondict:
return prondict[word][0][-1]
return None
def get_related_words(word, pos):
synsets = wn.synsets(word, pos=pos)
related_words = set()
for synset in synsets:
for lemma in synset.lemmas():
related_words.add(lemma.name())
if lemma.antonyms():
related_words.add(lemma.antonyms()[0].name())
return list(related_words)
def generate_line():
template = random.choice(templates)
noun1 = random.choice(nouns)
noun2 = random.choice(nouns)
verb = random.choice(verbs)
related_adjectives = get_related_words(noun1, wn.NOUN)
if related_adjectives:
adjective = random.choice(related_adjectives)
else:
adjective = random.choice(adjectives)
line = template.format(noun=noun1, verb=verb, adjective=adjective, noun2=noun2)
return line
def generate_rhyming_lines():
line1 = generate_line()
rhyme1 = get_rhyme(line1.split()[-1])
while True:
line2 = generate_line()
if get_rhyme(line2.split()[-1]) == rhyme1:
break
return line1, line2
def generate_poem(lines=4):
poem = []
for _ in range(lines // 2):
line1, line2 = generate_rhyming_lines()
poem.append(line1)
poem.append(line2)
return "\n".join(poem)
print(generate_poem())
通过这种方式,我们可以利用Python生成结构化、有韵律且语义连贯的诗句,进一步感受编程与艺术结合的魅力。
相关问答FAQs:
如何选择主题来创作Python诗歌?
选择主题是诗歌创作的重要一步。可以考虑自然、爱情、时间等永恒的话题,或是日常生活中的细微观察。使用Python,您可以随机生成主题,甚至从文本文件中读取关键词,作为灵感来源。
Python中是否有现成的库可以帮助生成诗歌?
是的,Python有一些库可以帮助生成诗歌,例如textgenrnn
和markovify
。这些库利用机器学习和马尔可夫链算法,能够根据输入的文本生成新的诗句。您可以通过训练这些模型,使其更符合您的风格和主题。
在Python中如何处理韵律和节奏?
处理韵律和节奏可以通过分析诗句的音节来实现。可以使用nltk
库中的音节分割功能,帮助您确定每行的音节数。通过这些工具,您可以确保诗歌在音韵上和谐,增加其艺术性和可读性。
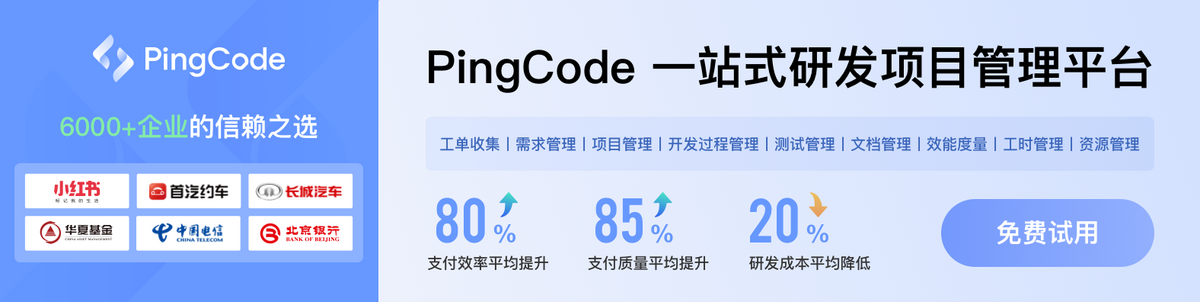