在Python 3中,有几种方法可以将数据类型转换为数字,包括使用内置函数int()
、float()
和complex()
。这些函数可以将字符串、浮点数或其他数字类型转换为整数、浮点数或复数。以下是详细介绍这些方法以及使用示例的详细描述。
一、使用 int()
函数
int()
函数可以将一个字符串或浮点数转换为整数。如果传入的参数是一个浮点数,int()
将其截断(不是四舍五入),如果是字符串,则该字符串必须表示一个整数。
# Example of converting string to integer
num_str = "123"
num_int = int(num_str)
print(num_int) # Output: 123
Example of converting float to integer
num_float = 123.45
num_int = int(num_float)
print(num_int) # Output: 123
注意:int()
不能将包含小数的字符串直接转换为整数,会引发 ValueError
。
二、使用 float()
函数
float()
函数将一个字符串或整数转换为浮点数。如果传入的参数是字符串,该字符串必须表示一个浮点数或整数。
# Example of converting string to float
num_str = "123.45"
num_float = float(num_str)
print(num_float) # Output: 123.45
Example of converting integer to float
num_int = 123
num_float = float(num_int)
print(num_float) # Output: 123.0
三、使用 complex()
函数
complex()
函数将一个字符串或数字转换为复数。如果传入的是两个参数,分别表示实部和虚部;如果传入一个字符串,则该字符串必须表示一个复数。
# Example of converting string to complex
num_str = "123+45j"
num_complex = complex(num_str)
print(num_complex) # Output: (123+45j)
Example of converting two numbers to complex
real_part = 123
imaginary_part = 45
num_complex = complex(real_part, imaginary_part)
print(num_complex) # Output: (123+45j)
四、使用 numpy
模块中的数据类型转换
如果你正在处理大量数据或需要进行科学计算,numpy
模块提供了非常高效的数组和数据类型转换方法。numpy
提供的 astype()
方法可以将数组中的元素转换为指定的类型。
import numpy as np
Example of creating a numpy array and converting its type
arr = np.array([1.2, 3.4, 5.6])
arr_int = arr.astype(int)
print(arr_int) # Output: [1 3 5]
Example of converting numpy array elements to float
arr_int = np.array([1, 2, 3])
arr_float = arr_int.astype(float)
print(arr_float) # Output: [1. 2. 3.]
五、处理异常情况
在数据类型转换过程中,常常会遇到无法直接转换的数据类型,这时需要进行异常处理,确保程序不会因错误而中断。
# Example of handling exceptions during conversion
def convert_to_int(value):
try:
return int(value)
except ValueError:
print(f"ValueError: Cannot convert {value} to integer.")
return None
Example of handling float conversion exceptions
def convert_to_float(value):
try:
return float(value)
except ValueError:
print(f"ValueError: Cannot convert {value} to float.")
return None
Testing the functions
print(convert_to_int("123")) # Output: 123
print(convert_to_int("abc")) # Output: ValueError: Cannot convert abc to integer. None
print(convert_to_float("123.45")) # Output: 123.45
print(convert_to_float("abc")) # Output: ValueError: Cannot convert abc to float. None
六、总结
在Python 3中,将数据类型转换为数字的方法包括使用内置函数 int()
、float()
和 complex()
,以及 numpy
模块中的 astype()
方法。这些方法可以处理不同的数据类型,并将其转换为整数、浮点数或复数。在实际应用中,处理数据类型转换时也要注意异常处理,以确保程序的健壮性和稳定性。
通过掌握这些方法和技巧,你可以在Python编程中更加灵活地处理数据类型转换,提升代码的可读性和执行效率。
相关问答FAQs:
如何在Python3中将字符串转换为数字类型?
在Python3中,可以使用内置函数int()
或float()
来将字符串转换为相应的数字类型。如果字符串表示一个整数,可以使用int()
将其转换为整数;如果字符串表示一个小数,则可以使用float()
将其转换为浮点数。例如,num = int("123")
将字符串"123"转换为整数123,而num = float("123.45")
则将字符串"123.45"转换为浮点数123.45。
在Python3中,如何检查一个变量的类型?
您可以使用内置函数type()
来检查变量的类型。调用type(variable)
将返回该变量的数据类型。例如,num = 123
,通过print(type(num))
将输出<class 'int'>
,表明num
是整数类型。对于浮点数或字符串,操作类似,只需将相应变量传递给type()
即可。
如何处理Python3中数字类型转换时的错误?
在进行数字类型转换时,可能会遇到ValueError
,例如将无法转换为数字的字符串传递给int()
或float()
。为了安全地处理这些情况,可以使用try
和except
语句。例如:
try:
num = int("abc") # 这将引发ValueError
except ValueError:
print("无法将字符串转换为数字")
这种方式允许您捕捉并处理转换失败的情况,从而避免程序崩溃。
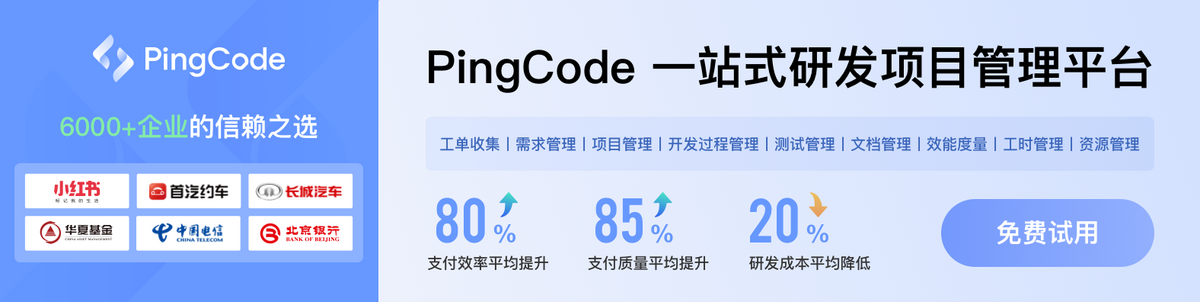