在Python中输入一个坐标的方式有很多种,常用的方式包括:直接输入坐标值、使用input函数获取用户输入、从文件读取数据。其中,使用input函数获取用户输入是一种灵活且常用的方法。我们可以使用input函数提示用户输入坐标值,并对输入的数据进行处理和验证,从而得到有效的坐标值。这种方法的灵活性在于可以处理任意格式的用户输入,并且可以根据需求进行相应的处理和验证。
一、直接输入坐标值
直接输入坐标值是最简单的方式,适用于程序中已经确定的坐标。例如:
# 直接输入坐标值
x = 5
y = 10
coordinate = (x, y)
print("The coordinate is:", coordinate)
这种方式适用于坐标值已经确定的情况,代码简洁明了,但灵活性较差。
二、使用input函数获取用户输入
使用input函数获取用户输入是一种灵活且常用的方法。可以根据用户输入的字符串进行解析和处理,从而得到坐标值。例如:
# 使用input函数获取用户输入
x = float(input("Enter the x coordinate: "))
y = float(input("Enter the y coordinate: "))
coordinate = (x, y)
print("The coordinate is:", coordinate)
这种方式可以灵活处理用户输入,适用于需要动态获取坐标值的情况。需要注意的是,需要对用户输入的数据进行验证,确保输入的坐标值是有效的。
三、从文件读取数据
从文件中读取数据也是一种常用的方法,适用于坐标数据保存在文件中的情况。例如:
# 从文件中读取坐标数据
with open('coordinates.txt', 'r') as file:
line = file.readline()
x, y = map(float, line.split())
coordinate = (x, y)
print("The coordinate is:", coordinate)
这种方式适用于大批量坐标数据的处理,可以方便地从文件中读取数据并进行处理。
四、使用函数封装
为了提高代码的复用性和可读性,可以将获取坐标值的代码封装成函数。例如:
def get_coordinate():
x = float(input("Enter the x coordinate: "))
y = float(input("Enter the y coordinate: "))
return (x, y)
coordinate = get_coordinate()
print("The coordinate is:", coordinate)
这种方式提高了代码的复用性和可读性,适用于需要多次获取坐标值的情况。
五、使用图形界面获取坐标值
在一些图形化的应用程序中,可以使用图形界面获取坐标值。例如使用Tkinter库:
import tkinter as tk
def get_coordinate():
def on_click(event):
coordinate = (event.x, event.y)
print("The coordinate is:", coordinate)
root.destroy()
root = tk.Tk()
root.bind("<Button-1>", on_click)
root.mainloop()
get_coordinate()
这种方式适用于图形化应用程序,用户体验较好。
六、使用第三方库获取坐标值
在一些特殊应用场景下,可以使用第三方库获取坐标值。例如使用Pygame库:
import pygame
def get_coordinate():
pygame.init()
screen = pygame.display.set_mode((640, 480))
pygame.display.set_caption("Click to get coordinate")
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONDOWN:
coordinate = event.pos
print("The coordinate is:", coordinate)
running = False
pygame.quit()
get_coordinate()
这种方式适用于游戏开发等需要获取鼠标点击坐标的场景。
七、使用正则表达式验证用户输入
为了提高用户输入的可靠性,可以使用正则表达式对用户输入进行验证。例如:
import re
def get_coordinate():
pattern = re.compile(r'^\s*([-+]?\d*\.?\d+)\s*,\s*([-+]?\d*\.?\d+)\s*$')
while True:
user_input = input("Enter the coordinate (x, y): ")
match = pattern.match(user_input)
if match:
x, y = map(float, match.groups())
return (x, y)
else:
print("Invalid input. Please enter the coordinate in the format (x, y).")
coordinate = get_coordinate()
print("The coordinate is:", coordinate)
这种方式可以有效验证用户输入的格式,确保输入的坐标值是有效的。
八、使用类封装坐标处理
为了更好地组织代码,可以使用类封装坐标的处理。例如:
class Coordinate:
def __init__(self, x=0, y=0):
self.x = x
self.y = y
def input(self):
self.x = float(input("Enter the x coordinate: "))
self.y = float(input("Enter the y coordinate: "))
def __str__(self):
return f"({self.x}, {self.y})"
coordinate = Coordinate()
coordinate.input()
print("The coordinate is:", coordinate)
这种方式提高了代码的组织性和可维护性,适用于复杂应用程序的开发。
九、使用JSON格式存储和读取坐标
在一些应用场景下,使用JSON格式存储和读取坐标是一个很好的选择。例如:
import json
def save_coordinate(coordinate, filename):
with open(filename, 'w') as file:
json.dump(coordinate, file)
def load_coordinate(filename):
with open(filename, 'r') as file:
coordinate = json.load(file)
return coordinate
coordinate = (5, 10)
save_coordinate(coordinate, 'coordinate.json')
loaded_coordinate = load_coordinate('coordinate.json')
print("The loaded coordinate is:", loaded_coordinate)
这种方式适用于需要持久化存储坐标数据的场景,可以方便地进行数据的存储和读取。
十、使用列表或字典存储多个坐标
在一些应用场景下,需要处理多个坐标,可以使用列表或字典存储坐标。例如:
# 使用列表存储多个坐标
coordinates = [(1, 2), (3, 4), (5, 6)]
print("The coordinates are:", coordinates)
使用字典存储多个坐标
coordinates_dict = {'point1': (1, 2), 'point2': (3, 4), 'point3': (5, 6)}
print("The coordinates dictionary is:", coordinates_dict)
这种方式适用于需要处理多个坐标的情况,可以方便地进行坐标的存储和管理。
结论
在Python中输入一个坐标有多种方式,具体选择哪种方式取决于应用场景的需求。直接输入坐标值适用于坐标已经确定的情况,使用input函数获取用户输入适用于需要动态获取坐标值的情况,从文件读取数据适用于大批量坐标数据的处理,使用函数封装可以提高代码的复用性和可读性,使用图形界面获取坐标值适用于图形化应用程序,使用第三方库获取坐标值适用于特殊应用场景,使用正则表达式验证用户输入可以提高用户输入的可靠性,使用类封装坐标处理可以提高代码的组织性和可维护性,使用JSON格式存储和读取坐标适用于需要持久化存储坐标数据的场景,使用列表或字典存储多个坐标适用于需要处理多个坐标的情况。根据具体的应用场景选择合适的方式,可以有效地实现坐标的输入和处理。
相关问答FAQs:
如何在Python中输入一个坐标?
在Python中,可以使用内置的input()
函数来接收用户的坐标输入。通常,你可以提示用户输入x和y坐标,例如:
x = float(input("请输入x坐标: "))
y = float(input("请输入y坐标: "))
这样的代码将会使用户能够输入浮点数形式的坐标值。
输入坐标时需要注意哪些数据类型?
输入坐标时,通常需要确保用户输入的是数值类型,如整数或浮点数。为了处理可能的输入错误,可以使用try...except
语句来捕获异常,确保程序的稳定性。示例代码如下:
try:
x = float(input("请输入x坐标: "))
y = float(input("请输入y坐标: "))
except ValueError:
print("请输入有效的数字。")
有没有推荐的库可以处理坐标输入与计算?
若需要进行更复杂的坐标计算或处理,可以考虑使用numpy
或pandas
等库。它们提供了强大的数据处理能力,能够让你更容易地管理和操作坐标数据。例如,使用numpy
可以轻松地进行向量运算和坐标转换。
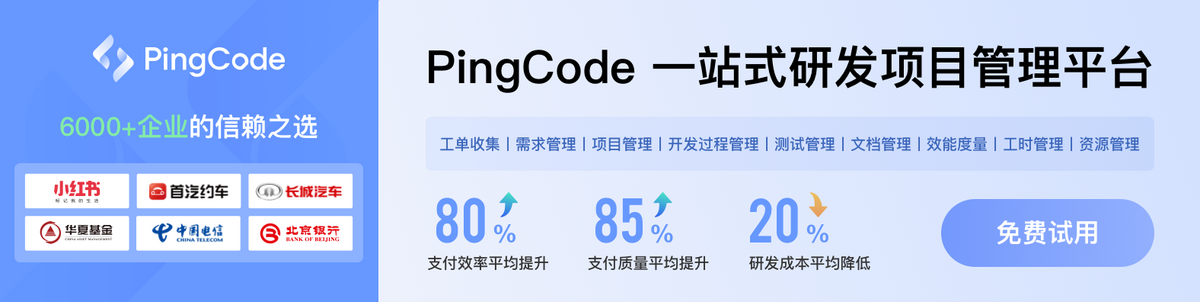