Python 正则表达式的使用非常方便、功能强大、能够处理复杂的字符串匹配需求。
首先,要使用 Python 正则表达式,您需要导入内置的 re
模块。其次,理解和编写正则表达式模式是关键。正则表达式可以用来查找、匹配、替换和拆分字符串中的特定模式。
以下是详细介绍如何在 Python 中使用正则表达式来找到特定的内容:
一、导入 re
模块
在开始使用正则表达式之前,需要导入 Python 内置的 re
模块:
import re
二、编写正则表达式模式
正则表达式模式是一种用来描述字符组合的字符串。以下是一些常用的模式:
.
匹配任意字符(除换行符)。\d
匹配一个数字字符,相当于[0-9]
。\D
匹配一个非数字字符,相当于[^0-9]
。\w
匹配一个字母数字字符,相当于[a-zA-Z0-9_]
。\W
匹配一个非字母数字字符,相当于[^a-zA-Z0-9_]
。\s
匹配一个空白字符,相当于[ \t\n\r\f\v]
。\S
匹配一个非空白字符,相当于[^ \t\n\r\f\v]
。
三、基本方法
-
re.match()
re.match()
尝试从字符串的起始位置匹配一个模式。如果匹配成功,则返回一个匹配对象;否则返回None
。pattern = r'\d+'
string = '123abc'
match = re.match(pattern, string)
if match:
print('Match found:', match.group())
else:
print('No match')
-
re.search()
re.search()
扫描整个字符串并返回第一个成功匹配的对象。pattern = r'\d+'
string = 'abc123def'
match = re.search(pattern, string)
if match:
print('Search found:', match.group())
else:
print('No match')
-
re.findall()
re.findall()
返回字符串中所有不重叠的匹配对象列表。pattern = r'\d+'
string = 'abc123def456'
matches = re.findall(pattern, string)
print('Findall found:', matches)
-
re.finditer()
re.finditer()
返回一个匹配对象的迭代器。pattern = r'\d+'
string = 'abc123def456'
matches = re.finditer(pattern, string)
for match in matches:
print('Finditer found:', match.group())
四、正则表达式的高级用法
-
捕获组
使用括号
()
可以在正则表达式中创建捕获组,以便在匹配时提取子模式。pattern = r'(\d+)-(\d+)-(\d+)'
string = '123-456-789'
match = re.match(pattern, string)
if match:
print('Full match:', match.group())
print('Group 1:', match.group(1))
print('Group 2:', match.group(2))
print('Group 3:', match.group(3))
-
非捕获组
使用
(?:...)
可以创建非捕获组,这对于仅需要匹配而不需要提取的情况非常有用。pattern = r'(?:\d+)-(\d+)-(\d+)'
string = '123-456-789'
match = re.match(pattern, string)
if match:
print('Full match:', match.group())
print('Group 1:', match.group(1))
print('Group 2:', match.group(2))
-
零宽断言
零宽断言包括正向先行断言
(?=...)
和正向后发断言(?<=...)
,用于匹配某个位置前或后的特定模式。# 正向先行断言
pattern = r'\d+(?=abc)'
string = '123abc456'
match = re.search(pattern, string)
if match:
print('Positive lookahead found:', match.group())
正向后发断言
pattern = r'(?<=abc)\d+'
string = 'abc123def456'
match = re.search(pattern, string)
if match:
print('Positive lookbehind found:', match.group())
-
替换
使用
re.sub()
可以替换字符串中所有符合正则表达式的子串。pattern = r'\d+'
string = 'abc123def456'
replaced_string = re.sub(pattern, 'NUMBER', string)
print('Replaced string:', replaced_string)
-
拆分
使用
re.split()
可以根据正则表达式分割字符串。pattern = r'\d+'
string = 'abc123def456'
split_list = re.split(pattern, string)
print('Split list:', split_list)
五、常见的正则表达式应用场景
-
匹配电子邮件地址
pattern = r'[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+'
string = 'Please contact us at support@example.com for further information.'
match = re.search(pattern, string)
if match:
print('Email found:', match.group())
-
匹配电话号码
pattern = r'\(?\d{3}\)?-?\s*\d{3}-?\s*\d{4}'
string = 'You can reach me at (123) 456-7890 or 987-654-3210.'
matches = re.findall(pattern, string)
print('Phone numbers found:', matches)
-
匹配 URL
pattern = r'https?://(?:www\.)?\w+\.\w+(?:/\w+)*'
string = 'Visit us at https://www.example.com or http://example.org.'
matches = re.findall(pattern, string)
print('URLs found:', matches)
-
匹配日期
pattern = r'\b\d{4}-\d{2}-\d{2}\b'
string = 'The event is scheduled on 2023-10-25.'
match = re.search(pattern, string)
if match:
print('Date found:', match.group())
六、正则表达式调试工具
使用正则表达式调试工具可以帮助您测试和调试正则表达式模式。以下是一些常用的在线工具:
七、性能优化
-
预编译正则表达式
对于需要频繁使用的正则表达式,可以使用
re.compile()
预编译正则表达式模式以提高性能。pattern = re.compile(r'\d+')
matches = pattern.findall('abc123def456')
print('Precompiled findall found:', matches)
-
避免使用复杂的模式
简化正则表达式模式可以提高匹配速度。
# 复杂模式
pattern = r'(\d{3}|\(\d{3}\))(-|\s)?\d{3}(-|\s)\d{4}'
简化模式
pattern = r'\(?\d{3}\)?-?\s*\d{3}-?\s*\d{4}'
-
使用原始字符串
使用原始字符串(即在字符串前加
r
)可以避免转义字符的双重处理。pattern = r'\d+'
八、常见问题和解决方案
-
正则表达式匹配不到预期的结果
- 检查正则表达式是否正确书写。
- 使用正则表达式调试工具进行测试。
- 确保匹配模式和字符串一致。
-
正则表达式匹配速度慢
- 简化正则表达式模式。
- 预编译正则表达式。
- 避免过于复杂的匹配模式。
-
无法匹配多行字符串
- 使用
re.DOTALL
标志使.
匹配包括换行符在内的所有字符。 - 使用
re.MULTILINE
标志使^
和$
匹配每一行的开头和结尾。
pattern = re.compile(r'^abc', re.MULTILINE)
string = 'abc\nabc\nabc'
matches = pattern.findall(string)
print('Multiline findall found:', matches)
- 使用
通过本文的介绍,您应该对 Python 正则表达式的基本用法和高级技巧有了较为全面的了解。掌握这些技能将帮助您在处理字符串数据时更加得心应手。
相关问答FAQs:
如何在Python中使用正则表达式进行模式匹配?
在Python中,可以使用re
模块来进行正则表达式的操作。通过re.search()
、re.match()
和re.findall()
等函数,可以在字符串中查找特定的模式。re.search()
会在字符串中查找第一个匹配项,re.match()
会从字符串的起始位置开始匹配,而re.findall()
则会返回所有匹配的结果。使用这些函数时,需要传入正则表达式和目标字符串。
如何编写复杂的正则表达式以提高匹配精度?
编写复杂的正则表达式时,可以利用字符类、量词、分组和反向引用等功能。例如,使用[a-zA-Z]
来匹配字母,使用+
来表示一个或多个字符,使用(...)
进行分组匹配。通过组合这些元素,可以构建出更精确的匹配模式。同时,使用re.compile()
可以将正则表达式编译成一个对象,以便于多次使用。
在Python中如何处理正则表达式的匹配结果?
正则表达式的匹配结果通常是一个Match
对象,其中包含了匹配的详细信息。可以通过group()
方法获取匹配的字符串,通过start()
和end()
方法获取匹配的起始和结束位置。如果使用re.findall()
,则返回的是一个列表,包含了所有匹配的结果。理解这些返回值有助于对匹配结果进行后续处理和分析。
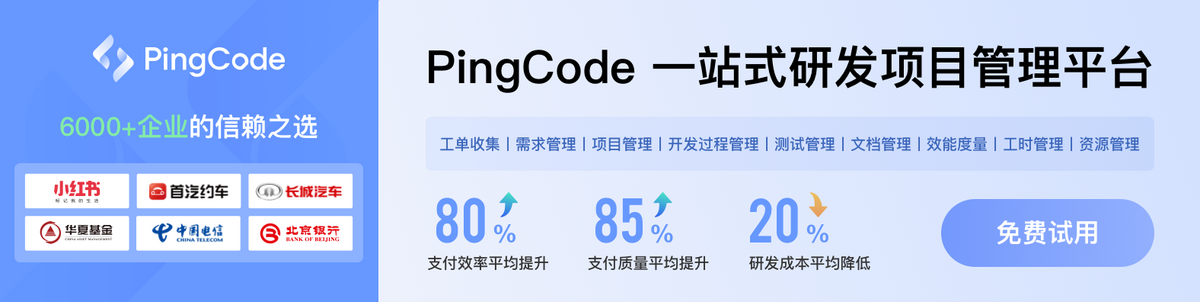