在Python3中,可以通过调整请求库的超时时间来加长访问网址的时长。具体方法包括设置长时间的超时时间、使用会话对象重复请求、处理异常等。下面我们将详细描述如何实现这一点。
一、使用requests库设置超时时间
在Python3中,requests库是一个非常流行的HTTP库,它允许我们非常方便地发送HTTP请求。通过设置requests的timeout参数,我们可以控制请求的超时时间。下面是一个基本的示例:
import requests
url = 'http://example.com'
try:
response = requests.get(url, timeout=10) # 设置超时时间为10秒
print(response.content)
except requests.exceptions.Timeout:
print('请求超时')
except requests.exceptions.RequestException as e:
print(f'请求发生异常: {e}')
timeout参数可以是一个数字,表示等待时间的秒数,也可以是一个元组,分别设置连接和读取的超时时间。
response = requests.get(url, timeout=(5, 10)) # 连接超时5秒,读取超时10秒
二、使用会话对象重复请求
有时候我们需要在请求失败后自动重试,可以使用requests库中的Session对象来实现。Session对象允许我们跨请求保持某些参数,并可以通过挂钩机制实现自动重试。
import requests
from requests.adapters import HTTPAdapter
from requests.packages.urllib3.util.retry import Retry
url = 'http://example.com'
session = requests.Session()
retry = Retry(total=5, backoff_factor=1, status_forcelist=[500, 502, 503, 504])
adapter = HTTPAdapter(max_retries=retry)
session.mount('http://', adapter)
session.mount('https://', adapter)
try:
response = session.get(url, timeout=10)
print(response.content)
except requests.exceptions.RequestException as e:
print(f'请求发生异常: {e}')
在这个示例中,我们使用Retry对象来配置重试策略,包括总重试次数、退避因子和针对哪些HTTP状态码重试。
三、处理异常
在网络请求过程中,可能会遇到各种异常情况,例如连接超时、读取超时等。通过捕获这些异常,我们可以进行相应的处理,确保程序的健壮性。
import requests
url = 'http://example.com'
try:
response = requests.get(url, timeout=10)
response.raise_for_status() # 检查是否返回了成功的状态码
print(response.content)
except requests.exceptions.Timeout:
print('请求超时')
except requests.exceptions.HTTPError as e:
print(f'HTTP请求错误: {e}')
except requests.exceptions.RequestException as e:
print(f'请求发生异常: {e}')
通过捕获不同的异常类型,我们可以针对不同的异常情况采取不同的处理措施,例如重试请求、记录日志等。
四、使用异步请求
对于需要处理大量并发请求的情况,可以考虑使用异步请求库,例如aiohttp。aiohttp库支持异步HTTP请求,可以显著提高并发请求的效率。
import aiohttp
import asyncio
async def fetch(url):
async with aiohttp.ClientSession() as session:
try:
async with session.get(url, timeout=10) as response:
return await response.text()
except asyncio.TimeoutError:
print('请求超时')
url = 'http://example.com'
loop = asyncio.get_event_loop()
content = loop.run_until_complete(fetch(url))
print(content)
aiohttp库允许我们使用async/await语法进行异步编程,从而显著提高并发请求的效率。
五、使用代理服务器
在某些情况下,直接请求目标网址可能会受到限制或封锁,这时我们可以通过使用代理服务器来绕过这些限制。requests库支持通过设置proxies参数来使用代理服务器。
import requests
url = 'http://example.com'
proxies = {
'http': 'http://your_proxy_server:port',
'https': 'http://your_proxy_server:port',
}
try:
response = requests.get(url, proxies=proxies, timeout=10)
print(response.content)
except requests.exceptions.RequestException as e:
print(f'请求发生异常: {e}')
使用代理服务器时需要注意代理服务器的稳定性和可靠性,建议选择信誉良好的代理服务提供商。
六、使用连接池
对于频繁的HTTP请求,使用连接池可以显著提高请求的效率。requests库支持通过设置HTTPAdapter来使用连接池。
import requests
from requests.adapters import HTTPAdapter
url = 'http://example.com'
session = requests.Session()
adapter = HTTPAdapter(pool_connections=10, pool_maxsize=10)
session.mount('http://', adapter)
session.mount('https://', adapter)
try:
response = session.get(url, timeout=10)
print(response.content)
except requests.exceptions.RequestException as e:
print(f'请求发生异常: {e}')
通过配置连接池的大小,我们可以更高效地管理HTTP连接,减少连接建立和释放的开销。
七、使用多线程
在某些情况下,使用多线程可以提高HTTP请求的并发性能。通过使用threading库,我们可以轻松地实现多线程HTTP请求。
import requests
import threading
def fetch(url):
try:
response = requests.get(url, timeout=10)
print(response.content)
except requests.exceptions.RequestException as e:
print(f'请求发生异常: {e}')
url = 'http://example.com'
threads = []
for i in range(5):
thread = threading.Thread(target=fetch, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
使用多线程时需要注意线程安全和资源竞争问题,建议使用线程池或其他并发控制机制进行管理。
八、设置请求头
有时目标服务器可能会对特定的请求头进行限制,这时我们可以通过设置自定义的请求头来模拟合法的请求。
import requests
url = 'http://example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
}
try:
response = requests.get(url, headers=headers, timeout=10)
print(response.content)
except requests.exceptions.RequestException as e:
print(f'请求发生异常: {e}')
通过设置合适的请求头,我们可以模拟合法的浏览器请求,从而提高请求成功的概率。
九、使用cookies
有时目标服务器可能会对cookies进行验证,这时我们可以通过设置cookies来模拟合法的请求。requests库支持通过cookies参数设置cookies。
import requests
url = 'http://example.com'
cookies = {
'sessionid': 'your_session_id',
}
try:
response = requests.get(url, cookies=cookies, timeout=10)
print(response.content)
except requests.exceptions.RequestException as e:
print(f'请求发生异常: {e}')
通过设置合适的cookies,我们可以模拟合法的会话请求,从而提高请求成功的概率。
十、总结
通过上述方法,我们可以在Python3中实现加长访问网址的时长,从而提高HTTP请求的成功率和稳定性。在实际应用中,建议根据具体需求选择合适的方法,并结合多种技术手段进行优化,以达到最佳效果。
在实际应用中,我们还可以结合日志记录、性能监控、异常告警等技术手段,进一步提高HTTP请求的可靠性和可维护性。通过不断优化和改进,我们可以构建出更加健壮、高效的HTTP请求系统。
相关问答FAQs:
如何使用Python3设置访问网址的超时时间?
在Python3中,您可以使用requests
库的timeout
参数来设置访问网址的超时时间。例如,您可以在请求时指定一个超时值,以防止请求长时间挂起。以下是一个简单的例子:
import requests
try:
response = requests.get('https://example.com', timeout=10) # 设置超时时间为10秒
print(response.content)
except requests.exceptions.Timeout:
print("请求超时,请稍后再试。")
通过这种方式,您可以有效地管理请求的超时行为。
如何在Python3中处理长时间的网络请求?
处理长时间的网络请求可以通过异步编程来优化。使用asyncio
库结合aiohttp
库可以实现异步请求,这样可以在等待网络响应的同时执行其他代码。例如:
import aiohttp
import asyncio
async def fetch(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
async def main():
html = await fetch('https://example.com')
print(html)
asyncio.run(main())
这种方法可以提高程序的效率,特别是在需要处理多个请求时。
是否有其他方法可以延长Python3中访问网址的有效时间?
除了设置超时,还可以通过增加请求重试次数来延长访问网址的有效时间。您可以使用requests
库的Session
对象并结合Retry
策略来实现。例如:
from requests.adapters import HTTPAdapter
from requests.packages.urllib3.util.retry import Retry
import requests
session = requests.Session()
retry = Retry(total=5, backoff_factor=1)
adapter = HTTPAdapter(max_retries=retry)
session.mount('http://', adapter)
session.mount('https://', adapter)
response = session.get('https://example.com')
print(response.content)
通过这种方式,即使在遇到临时网络问题时,您的请求也能得到更好的处理。
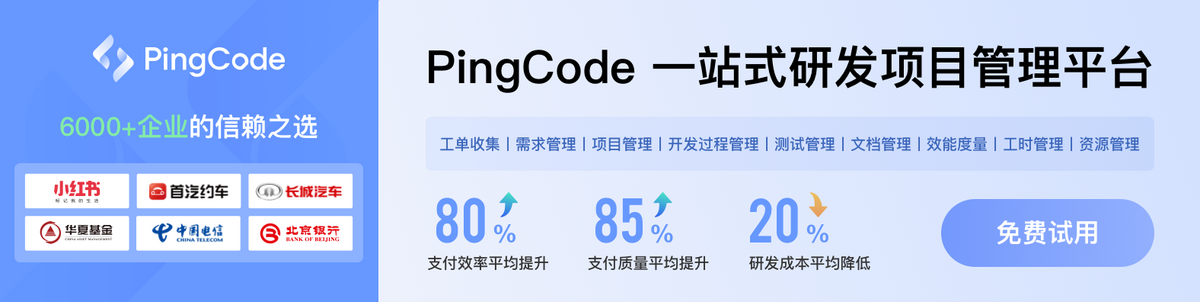