要用Python控制微博粉丝数,主要有以下几种方法:使用微博API接口、使用Web scraping技术、编写自动化脚本操作。
其中,使用微博API接口是最为推荐的方法,因为它是官方提供的方式,安全性和稳定性较高。具体来说,微博API提供了丰富的接口,可以用来获取粉丝列表、关注用户、取消关注等操作。
一、使用微博API接口
微博API是新浪微博官方提供的一套开发接口,开发者可以通过这些接口与微博进行交互,进行数据获取和操作。要使用微博API接口,首先需要注册微博开发者账号,创建应用,并获取应用的App Key和App Secret。
- 注册和创建应用
首先,登录新浪微博开放平台,注册成为开发者。然后创建一个新应用,填写应用的基本信息,提交审核。审核通过后,你将获得应用的App Key和App Secret。
- 获取授权
要使用微博API接口,需要获取用户的授权。用户授权后,应用将获得一个Access Token,用于后续的API调用。可以使用OAuth2.0协议来实现授权过程。具体的流程如下:
- 引导用户访问授权页面,用户登录并授权后,微博会重定向到回调地址,并带上授权码(Authorization Code)。
- 使用授权码换取Access Token。
Python代码示例:
import requests
Step 1: Get Authorization Code
auth_url = "https://api.weibo.com/oauth2/authorize"
params = {
"client_id": "your_app_key",
"redirect_uri": "your_redirect_uri",
"response_type": "code"
}
response = requests.get(auth_url, params=params)
print("Visit this URL to authorize the application:", response.url)
Step 2: Get Access Token
auth_code = input("Enter the authorization code: ")
token_url = "https://api.weibo.com/oauth2/access_token"
data = {
"client_id": "your_app_key",
"client_secret": "your_app_secret",
"grant_type": "authorization_code",
"code": auth_code,
"redirect_uri": "your_redirect_uri"
}
response = requests.post(token_url, data=data)
access_token = response.json().get("access_token")
print("Access Token:", access_token)
- 使用API接口
获取到Access Token后,可以使用微博API接口进行各种操作。以下是一个获取粉丝列表的示例:
# Get Followers List
followers_url = "https://api.weibo.com/2/friendships/followers.json"
params = {
"access_token": access_token,
"uid": "your_user_id",
"count": 50
}
response = requests.get(followers_url, params=params)
followers = response.json().get("users")
for follower in followers:
print(follower["screen_name"])
二、使用Web scraping技术
如果微博API接口无法满足需求,可以考虑使用Web scraping技术,通过模拟浏览器访问微博网页,解析网页内容来获取数据。需要注意的是,Web scraping可能违反微博的使用条款,而且微博会不断更新网页结构,导致代码需要频繁维护。
- 安装必要的Python库
pip install requests beautifulsoup4 lxml selenium
- 使用Selenium模拟浏览器操作
Selenium是一个用于自动化测试网页的工具,可以用来模拟用户操作,如登录微博、关注用户等。
Python代码示例:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
Initialize WebDriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
Open Weibo login page
driver.get("https://weibo.com/login.php")
Login to Weibo
username = driver.find_element_by_name("username")
password = driver.find_element_by_name("password")
username.send_keys("your_username")
password.send_keys("your_password")
password.send_keys(Keys.RETURN)
Wait for the page to load
time.sleep(5)
Navigate to the user's followers page
driver.get("https://weibo.com/u/your_user_id/followers")
Wait for the page to load
time.sleep(5)
Scrape followers list
followers = driver.find_elements_by_css_selector(".follow_item .name")
for follower in followers:
print(follower.text)
Close the browser
driver.quit()
三、编写自动化脚本操作
可以编写Python脚本,结合微博API接口和Web scraping技术,实现自动化操作。例如,可以编写一个脚本,定期关注和取消关注特定用户,以控制微博粉丝数。
import requests
import time
access_token = "your_access_token"
def follow_user(user_id):
follow_url = "https://api.weibo.com/2/friendships/create.json"
params = {
"access_token": access_token,
"uid": user_id
}
response = requests.post(follow_url, params=params)
return response.json()
def unfollow_user(user_id):
unfollow_url = "https://api.weibo.com/2/friendships/destroy.json"
params = {
"access_token": access_token,
"uid": user_id
}
response = requests.post(unfollow_url, params=params)
return response.json()
Follow and unfollow users to control followers count
user_ids = ["user_id_1", "user_id_2", "user_id_3"]
while True:
for user_id in user_ids:
follow_user(user_id)
time.sleep(10) # Wait for 10 seconds before unfollowing
unfollow_user(user_id)
time.sleep(10) # Wait for 10 seconds before following the next user
总结来说,使用Python控制微博粉丝数的主要方法有:使用微博API接口、使用Web scraping技术、编写自动化脚本操作。其中,使用微博API接口是最为推荐的方法,因为它是官方提供的方式,安全性和稳定性较高。通过合理使用这些方法,可以实现对微博粉丝数的控制和管理。
此外,需要注意的是,在使用这些技术时,要遵守微博的使用条款和隐私政策,避免恶意操作和滥用行为。希望这篇文章对你有所帮助,祝你在微博运营中取得成功!
相关问答FAQs:
如何使用Python自动化管理微博粉丝互动?
使用Python可以通过微博API进行粉丝互动管理,例如评论、转发和点赞等。通过编写脚本,你可以定期与粉丝进行互动,增加粉丝的黏性,从而间接影响粉丝增长。推荐使用requests
库进行API调用,并结合time
库进行定时任务设置。
怎样使用Python分析微博粉丝数据?
利用Python的数据分析库如Pandas和Matplotlib,可以对微博粉丝数据进行深入分析。通过抓取相关数据,清洗并可视化,可以洞察粉丝增长趋势、活跃度等,从而优化微博运营策略,促进粉丝数增长。
是否可以使用Python进行微博粉丝管理的批量操作?
可以实现批量操作。例如,通过编写Python脚本,利用微博API同时关注、取消关注多个用户,或批量发送私信和评论。不过,在进行这些操作时,务必遵守微博平台的使用规定,以避免账号被封禁的风险。
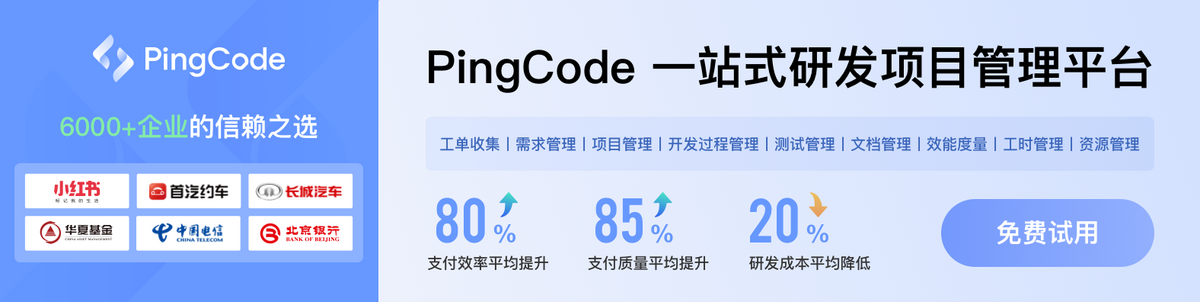