在 Python 中设置循环输入密码的方法有很多,主要取决于具体的需求和应用场景。常见的方法包括使用 while
循环、for
循环、以及结合条件判断来实现。可以使用 while
循环、设置最大尝试次数、引入时间延迟来增强安全性。下面将详细介绍使用 while
循环来实现循环输入密码的方法。
一、使用 while
循环
使用 while
循环是实现循环输入密码的最常见方法。通过 while
循环和条件判断,可以让用户多次尝试输入密码,直到输入正确或达到最大尝试次数。
# 定义正确的密码
correct_password = "secret123"
初始化输入密码的变量
input_password = ""
使用 while 循环让用户多次输入密码
while input_password != correct_password:
input_password = input("请输入密码:")
if input_password == correct_password:
print("密码正确,登录成功!")
else:
print("密码错误,请重试。")
二、设置最大尝试次数
为了防止用户无限次尝试密码,可以设置最大尝试次数。如果用户在规定的次数内未能输入正确密码,则提示用户并终止程序。
# 定义正确的密码
correct_password = "secret123"
初始化输入密码的变量
input_password = ""
设置最大尝试次数
max_attempts = 3
attempts = 0
使用 while 循环让用户多次输入密码
while input_password != correct_password and attempts < max_attempts:
input_password = input("请输入密码:")
attempts += 1
if input_password == correct_password:
print("密码正确,登录成功!")
else:
if attempts < max_attempts:
print("密码错误,请重试。")
else:
print("尝试次数过多,账户已锁定。")
三、引入时间延迟
为了增加安全性,可以在每次输入错误密码后引入时间延迟。这样可以防止暴力破解密码。
import time
定义正确的密码
correct_password = "secret123"
初始化输入密码的变量
input_password = ""
设置最大尝试次数
max_attempts = 3
attempts = 0
使用 while 循环让用户多次输入密码
while input_password != correct_password and attempts < max_attempts:
input_password = input("请输入密码:")
attempts += 1
if input_password == correct_password:
print("密码正确,登录成功!")
else:
if attempts < max_attempts:
print("密码错误,请重试。")
# 引入时间延迟
time.sleep(2 attempts)
else:
print("尝试次数过多,账户已锁定。")
四、使用 for
循环
除了 while
循环,还可以使用 for
循环来实现循环输入密码的功能。
# 定义正确的密码
correct_password = "secret123"
设置最大尝试次数
max_attempts = 3
使用 for 循环让用户多次输入密码
for attempt in range(max_attempts):
input_password = input("请输入密码:")
if input_password == correct_password:
print("密码正确,登录成功!")
break
else:
if attempt < max_attempts - 1:
print("密码错误,请重试。")
else:
print("尝试次数过多,账户已锁定。")
五、结合条件判断和函数
通过将上述逻辑封装到函数中,可以提高代码的可重用性和可读性。
import time
def check_password(correct_password, max_attempts=3):
attempts = 0
while attempts < max_attempts:
input_password = input("请输入密码:")
if input_password == correct_password:
print("密码正确,登录成功!")
return True
else:
attempts += 1
if attempts < max_attempts:
print("密码错误,请重试。")
time.sleep(2 attempts)
else:
print("尝试次数过多,账户已锁定。")
return False
调用函数进行密码验证
correct_password = "secret123"
check_password(correct_password)
六、使用 getpass
模块隐藏输入
在实际应用中,为了增强安全性,可以使用 getpass
模块隐藏用户输入的密码。
import getpass
import time
def check_password(correct_password, max_attempts=3):
attempts = 0
while attempts < max_attempts:
input_password = getpass.getpass("请输入密码:")
if input_password == correct_password:
print("密码正确,登录成功!")
return True
else:
attempts += 1
if attempts < max_attempts:
print("密码错误,请重试。")
time.sleep(2 attempts)
else:
print("尝试次数过多,账户已锁定。")
return False
调用函数进行密码验证
correct_password = "secret123"
check_password(correct_password)
七、综合实现示例
综合上述方法,可以实现一个完整的密码验证系统,既能设置最大尝试次数,又能隐藏输入,并引入时间延迟。
import getpass
import time
def check_password(correct_password, max_attempts=3):
attempts = 0
while attempts < max_attempts:
input_password = getpass.getpass("请输入密码:")
if input_password == correct_password:
print("密码正确,登录成功!")
return True
else:
attempts += 1
if attempts < max_attempts:
print("密码错误,请重试。")
time.sleep(2 attempts)
else:
print("尝试次数过多,账户已锁定。")
return False
调用函数进行密码验证
correct_password = "secret123"
check_password(correct_password)
通过以上几种方法,可以在 Python 中实现循环输入密码的功能。使用 while
循环、设置最大尝试次数、引入时间延迟可以有效提高密码验证的安全性和用户体验。根据具体需求和应用场景,选择合适的方法进行实现。
相关问答FAQs:
在Python中如何处理用户输入的密码以确保安全性?
在处理密码输入时,建议使用getpass
模块,这样可以隐藏用户输入的密码,避免在终端显示。使用getpass.getpass()
函数可以安全地获取用户输入的密码,而不暴露在控制台上。确保在接收密码后进行适当的哈希处理,以提高安全性。
如何在Python中限制用户输入密码的次数?
为了防止用户无限次尝试输入密码,可以设置一个最大尝试次数的限制。例如,可以使用一个计数器在循环中跟踪输入次数,一旦超过预设的次数,就可以退出循环并提示用户输入次数已用完。这种做法可以保护系统免受暴力破解攻击。
在Python中如何验证密码的正确性?
在验证密码时,可以将用户输入的密码与存储的哈希值进行比较。使用hashlib
库可以生成密码的哈希值,并与数据库中存储的哈希值进行匹配。确保使用安全的哈希算法(如SHA-256)来提高密码的安全性。
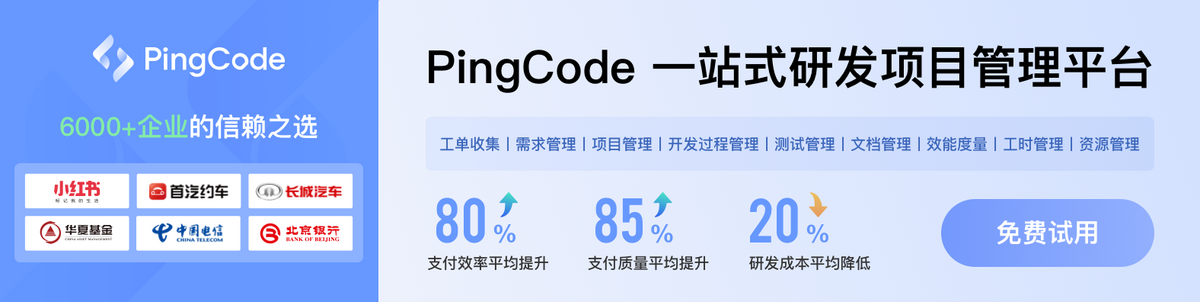