Python在浏览器中进行搜索的方法有:使用webbrowser模块、利用selenium自动化工具、通过requests库与BeautifulSoup结合进行搜索。在这些方法中,利用selenium自动化工具是最为常见和高效的方式,因为它不仅能模拟用户操作,还能处理动态页面。
使用selenium自动化工具进行浏览器搜索是一个非常强大的方法。首先,你需要安装selenium库和浏览器驱动程序(例如ChromeDriver)。然后,通过简单的Python代码,你可以打开浏览器、输入搜索关键字并提交搜索请求。selenium还支持各种浏览器,如Chrome、Firefox等,并且可以模拟用户的所有操作,如点击、填写表单、滚动页面等。
一、利用webbrowser模块进行搜索
webbrowser模块是Python标准库中的一个模块,可以使用它在默认浏览器中打开一个URL。
import webbrowser
def search_with_webbrowser(query):
url = f"https://www.google.com/search?q={query}"
webbrowser.open(url)
search_query = "python selenium tutorial"
search_with_webbrowser(search_query)
这个方法非常简单,但它的功能有限,只能打开浏览器并执行搜索,无法进行复杂的交互操作。
二、使用selenium自动化工具
selenium是一个功能强大的Web应用测试工具,可以用来自动化浏览器操作。
1、安装selenium和浏览器驱动
首先,安装selenium库:
pip install selenium
然后,下载与浏览器版本匹配的浏览器驱动程序(例如ChromeDriver),并确保其路径在环境变量中。
2、使用selenium进行搜索
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
def search_with_selenium(query):
# 初始化webdriver
driver = webdriver.Chrome()
# 打开浏览器并访问Google
driver.get("https://www.google.com")
# 找到搜索框并输入搜索关键字
search_box = driver.find_element_by_name("q")
search_box.send_keys(query)
search_box.send_keys(Keys.RETURN)
# 等待搜索结果加载
time.sleep(2)
# 打印搜索结果页面的标题
print(driver.title)
# 关闭浏览器
driver.quit()
search_query = "python selenium tutorial"
search_with_selenium(search_query)
3、处理动态页面和更多操作
selenium不仅能进行简单的搜索,还能处理复杂的操作,如点击按钮、填写表单、处理弹窗等。以下是一个更复杂的示例,展示如何使用selenium处理动态页面:
def advanced_search_with_selenium(query):
driver = webdriver.Chrome()
driver.get("https://www.google.com")
search_box = driver.find_element_by_name("q")
search_box.send_keys(query)
search_box.send_keys(Keys.RETURN)
time.sleep(2)
# 找到并点击第一个搜索结果链接
first_result = driver.find_element_by_css_selector("h3")
first_result.click()
# 等待页面加载
time.sleep(2)
# 打印当前页面的标题
print(driver.title)
driver.quit()
search_query = "python selenium advanced tutorial"
advanced_search_with_selenium(search_query)
三、通过requests库和BeautifulSoup进行搜索
requests库用于发送HTTP请求,BeautifulSoup用于解析HTML文档,可以结合这两个库进行网页搜索。但这种方法只能获取静态页面内容,无法处理动态内容。
1、安装requests和BeautifulSoup
pip install requests beautifulsoup4
2、使用requests和BeautifulSoup进行搜索
import requests
from bs4 import BeautifulSoup
def search_with_requests(query):
url = f"https://www.google.com/search?q={query}"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
# 打印搜索结果的标题
for result in soup.find_all("h3"):
print(result.get_text())
search_query = "python requests tutorial"
search_with_requests(search_query)
四、selenium与BeautifulSoup结合使用
selenium和BeautifulSoup可以结合使用,以应对复杂的动态页面解析需求。首先使用selenium获取动态加载的页面,然后使用BeautifulSoup解析页面内容。
1、selenium与BeautifulSoup结合示例
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
from bs4 import BeautifulSoup
def search_with_selenium_and_bs(query):
driver = webdriver.Chrome()
driver.get("https://www.google.com")
search_box = driver.find_element_by_name("q")
search_box.send_keys(query)
search_box.send_keys(Keys.RETURN)
time.sleep(2)
# 获取页面源代码
page_source = driver.page_source
# 使用BeautifulSoup解析页面源代码
soup = BeautifulSoup(page_source, "html.parser")
# 打印搜索结果的标题
for result in soup.find_all("h3"):
print(result.get_text())
driver.quit()
search_query = "python selenium BeautifulSoup tutorial"
search_with_selenium_and_bs(search_query)
五、总结
通过这几种方法,我们可以在Python中实现浏览器搜索的功能。webbrowser模块适用于简单的搜索操作,requests库和BeautifulSoup适用于静态页面的解析,而selenium自动化工具则是最强大的,适用于处理复杂的动态页面和用户交互。根据具体需求选择合适的方法,可以有效地提高工作效率。
相关问答FAQs:
如何使用Python自动在浏览器中执行搜索操作?
利用Python的Selenium库,可以模拟用户在浏览器中的操作,自动执行搜索。首先,安装Selenium库和相应的浏览器驱动。然后,编写一个简单的脚本,启动浏览器,打开搜索引擎页面,输入搜索关键词并提交。这样,Python就能在浏览器中完成搜索任务。
Python是否支持多种浏览器进行搜索?
是的,Python可以通过Selenium支持多种浏览器进行搜索,如Chrome、Firefox、Safari等。用户只需下载相应的浏览器驱动,并在代码中指定所使用的浏览器类型,就能够在不同的浏览器中执行搜索操作。
在使用Python进行浏览器搜索时,有哪些常见错误需要注意?
常见错误包括驱动程序与浏览器版本不兼容、未正确安装Selenium库、元素定位失败等。确保驱动程序与所用浏览器的版本匹配,且在编写脚本时,使用合适的方法来定位搜索框和提交按钮,能够有效减少错误发生的几率。
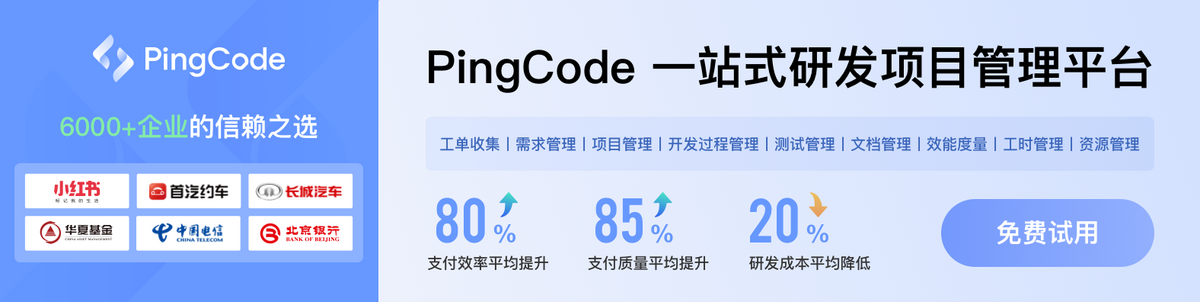