用Python灵活提取字段的方式主要包括正则表达式、字符串方法、pandas库、BeautifulSoup库等,其中最常用的方式是正则表达式,因为它提供了强大的文本匹配和提取功能。本文将详细介绍这些方式,并通过示例代码展示如何在不同场景中应用这些方法。
一、正则表达式
正则表达式是一种强大的文本匹配工具,可以用来查找和提取文本中的特定模式。在Python中,可以使用re
模块来处理正则表达式。以下是一些常见的使用场景和示例代码:
1.1 匹配固定格式的字段
import re
text = "My phone number is 123-456-7890."
pattern = r'\d{3}-\d{3}-\d{4}'
match = re.search(pattern, text)
if match:
print("Found phone number:", match.group())
1.2 提取多个字段
text = "Name: John Doe, Age: 30, Email: john.doe@example.com"
pattern = r'Name: (\w+ \w+), Age: (\d+), Email: (\S+)'
match = re.search(pattern, text)
if match:
name, age, email = match.groups()
print(f"Name: {name}, Age: {age}, Email: {email}")
1.3 使用捕获组提取字段
text = "Order ID: 12345, Product: Widget, Quantity: 10"
pattern = r'Order ID: (\d+), Product: (\w+), Quantity: (\d+)'
match = re.search(pattern, text)
if match:
order_id, product, quantity = match.groups()
print(f"Order ID: {order_id}, Product: {product}, Quantity: {quantity}")
二、字符串方法
Python的字符串方法也可以用来提取字段,特别是当字段的结构较为简单时。
2.1 使用split方法分割字符串
text = "Name: John Doe, Age: 30, Email: john.doe@example.com"
fields = text.split(', ')
for field in fields:
key, value = field.split(': ')
print(f"{key}: {value}")
2.2 使用strip和find方法
text = "Name: John Doe, Age: 30, Email: john.doe@example.com"
fields = ["Name", "Age", "Email"]
for field in fields:
start = text.find(field) + len(field) + 2
end = text.find(',', start)
if end == -1:
end = len(text)
value = text[start:end].strip()
print(f"{field}: {value}")
三、pandas库
当需要处理结构化数据(如CSV文件、Excel文件等)时,pandas库是一个非常强大的工具。它提供了灵活的数据处理和提取功能。
3.1 从CSV文件中提取字段
import pandas as pd
df = pd.read_csv('data.csv')
提取某一列
column_data = df['column_name']
print(column_data)
提取多列
multiple_columns = df[['column1', 'column2']]
print(multiple_columns)
3.2 使用条件过滤提取字段
filtered_data = df[df['age'] > 30]
print(filtered_data)
四、BeautifulSoup库
BeautifulSoup库主要用于解析和提取HTML和XML中的数据。它对于从网页中提取字段非常有用。
4.1 提取HTML中的字段
from bs4 import BeautifulSoup
html = """
<html>
<head><title>Test Page</title></head>
<body>
<p class="name">Name: John Doe</p>
<p class="age">Age: 30</p>
<p class="email">Email: john.doe@example.com</p>
</body>
</html>
"""
soup = BeautifulSoup(html, 'html.parser')
name = soup.find('p', class_='name').text.split(': ')[1]
age = soup.find('p', class_='age').text.split(': ')[1]
email = soup.find('p', class_='email').text.split(': ')[1]
print(f"Name: {name}, Age: {age}, Email: {email}")
4.2 提取表格中的字段
html = """
<table>
<tr><th>Name</th><th>Age</th><th>Email</th></tr>
<tr><td>John Doe</td><td>30</td><td>john.doe@example.com</td></tr>
<tr><td>Jane Doe</td><td>25</td><td>jane.doe@example.com</td></tr>
</table>
"""
soup = BeautifulSoup(html, 'html.parser')
table = soup.find('table')
rows = table.find_all('tr')[1:] # Skip the header row
for row in rows:
cols = row.find_all('td')
name = cols[0].text
age = cols[1].text
email = cols[2].text
print(f"Name: {name}, Age: {age}, Email: {email}")
五、JSON处理
JSON是一种常用的数据格式,Python提供了json
模块来处理JSON数据。
5.1 提取JSON中的字段
import json
json_data = '''
{
"name": "John Doe",
"age": 30,
"email": "john.doe@example.com",
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA"
}
}
'''
data = json.loads(json_data)
name = data['name']
age = data['age']
email = data['email']
street = data['address']['street']
print(f"Name: {name}, Age: {age}, Email: {email}, Street: {street}")
5.2 处理复杂JSON结构
json_data = '''
{
"orders": [
{"order_id": 12345, "product": "Widget", "quantity": 10},
{"order_id": 12346, "product": "Gadget", "quantity": 5}
]
}
'''
data = json.loads(json_data)
for order in data['orders']:
order_id = order['order_id']
product = order['product']
quantity = order['quantity']
print(f"Order ID: {order_id}, Product: {product}, Quantity: {quantity}")
六、XPath与lxml库
XPath是一种用于在XML文档中定位节点的语言。lxml
库可以结合XPath来处理和提取XML数据。
6.1 提取XML中的字段
from lxml import etree
xml = '''
<root>
<person>
<name>John Doe</name>
<age>30</age>
<email>john.doe@example.com</email>
</person>
<person>
<name>Jane Doe</name>
<age>25</age>
<email>jane.doe@example.com</email>
</person>
</root>
'''
tree = etree.fromstring(xml)
names = tree.xpath('//person/name/text()')
ages = tree.xpath('//person/age/text()')
emails = tree.xpath('//person/email/text()')
for name, age, email in zip(names, ages, emails):
print(f"Name: {name}, Age: {age}, Email: {email}")
七、使用第三方API
在一些特定的应用场景中,可能需要从第三方API中提取数据。以下示例展示了如何使用Python的requests
库从API中提取数据。
7.1 提取API返回的JSON数据
import requests
response = requests.get('https://api.example.com/data')
data = response.json()
for item in data:
name = item['name']
age = item['age']
email = item['email']
print(f"Name: {name}, Age: {age}, Email: {email}")
八、数据库查询
在处理存储在数据库中的数据时,可以使用SQL查询来提取所需的字段。以下示例展示了如何使用SQLite数据库进行查询。
8.1 提取数据库中的字段
import sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute('SELECT name, age, email FROM users')
rows = cursor.fetchall()
for row in rows:
name, age, email = row
print(f"Name: {name}, Age: {age}, Email: {email}")
conn.close()
九、总结
在本文中,我们介绍了多种使用Python提取字段的方法,包括正则表达式、字符串方法、pandas库、BeautifulSoup库、JSON处理、XPath与lxml库、第三方API和数据库查询。每种方法都有其适用的场景和优势,具体选择哪种方法取决于数据的结构和提取需求。希望这些示例能够帮助读者在实际项目中更加灵活地处理和提取数据。
相关问答FAQs:
提取字段时,Python中有哪些常用的库和工具?
Python提供了多种库和工具来灵活提取字段。其中,最常用的包括正则表达式(re模块)、Pandas库、Beautiful Soup(用于解析HTML和XML文件)以及JSON模块(用于处理JSON数据)。这些工具各有特点,正则表达式适合处理复杂的字符串模式,Pandas能够高效处理表格数据,而Beautiful Soup则非常适合从网页中提取信息。
在处理CSV文件时,如何使用Python提取特定字段?
处理CSV文件时,Pandas库提供了非常便利的功能。通过使用read_csv
函数,可以轻松读取CSV文件,并将其转换为DataFrame对象。提取特定字段只需通过列名索引,例如dataframe['column_name']
,即可获取相应的列数据。此外,Pandas支持多种数据处理功能,如过滤、分组和聚合,能够帮助用户灵活操作数据。
如何使用正则表达式在文本中提取特定模式的字段?
使用Python的re模块,可以通过定义正则表达式模式来提取特定字段。首先,使用re.compile()
编译正则表达式,然后利用re.findall()
或re.search()
函数在文本中查找匹配项。例如,若要提取邮箱地址,可以使用模式r'[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,6}'
,这样可以找到文本中的所有邮箱地址,并灵活处理它们。
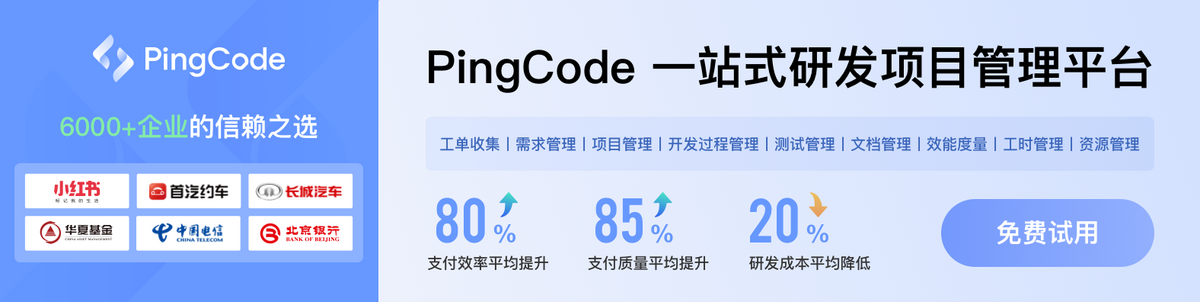