要用Python创建一个页面搜索引擎,可以利用抓取网页内容、处理和索引数据、以及搜索算法等技术。下面详细介绍如何实现这些步骤,并重点讨论如何利用Python的多种库来完成这一任务。
一、抓取网页内容
抓取网页内容是创建搜索引擎的第一步。Python有许多库可以帮助你抓取网页内容,如BeautifulSoup、Scrapy、Requests等。
1、使用Requests库抓取网页
Requests是一个简单易用的HTTP库,可以用来发送HTTP请求,获取网页内容。
import requests
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
page_content = response.text
print(page_content)
2、使用BeautifulSoup解析网页内容
BeautifulSoup是一个强大的HTML和XML解析库,可以轻松解析和提取网页中的数据。
from bs4 import BeautifulSoup
soup = BeautifulSoup(page_content, 'html.parser')
提取网页标题
title = soup.title.string
print(title)
提取所有段落
paragraphs = soup.find_all('p')
for paragraph in paragraphs:
print(paragraph.text)
3、使用Scrapy进行高级抓取
Scrapy是一个功能强大的网页抓取和数据提取框架,适合大规模抓取任务。
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
title = response.css('title::text').get()
paragraphs = response.css('p::text').getall()
yield {
'title': title,
'paragraphs': paragraphs
}
二、处理和索引数据
抓取到网页内容后,需要对数据进行处理和索引,以便快速搜索。可以使用NLTK、spaCy等自然语言处理库,以及Whoosh、Elasticsearch等全文搜索库。
1、使用NLTK进行文本处理
NLTK是一个强大的自然语言处理库,可以用来进行分词、词性标注、提取关键词等操作。
import nltk
from nltk.tokenize import word_tokenize
nltk.download('punkt')
text = "This is a sample text for tokenization."
tokens = word_tokenize(text)
print(tokens)
2、使用Whoosh进行全文搜索索引
Whoosh是一个纯Python编写的全文搜索库,适合小型到中型的搜索应用。
from whoosh import index
from whoosh.fields import Schema, TEXT
定义索引的模式
schema = Schema(title=TEXT(stored=True), content=TEXT)
创建索引
index_dir = 'indexdir'
if not os.path.exists(index_dir):
os.mkdir(index_dir)
ix = index.create_in(index_dir, schema)
添加文档到索引
writer = ix.writer()
writer.add_document(title="First document", content="This is the content of the first document.")
writer.add_document(title="Second document", content="This is some more content, in the second document.")
writer.commit()
三、搜索算法
实现搜索算法是搜索引擎的核心。可以使用TF-IDF、BM25等算法来计算文档与查询的相关性。
1、实现简单的TF-IDF
TF-IDF(Term Frequency-Inverse Document Frequency)是一种常用的文本相似度计算方法。
from sklearn.feature_extraction.text import TfidfVectorizer
documents = [
"This is the first document.",
"This document is the second document.",
"And this is the third one.",
"Is this the first document?",
]
vectorizer = TfidfVectorizer()
tfidf_matrix = vectorizer.fit_transform(documents)
查询
query = "first document"
query_vec = vectorizer.transform([query])
计算相似度
from sklearn.metrics.pairwise import cosine_similarity
similarity_scores = cosine_similarity(query_vec, tfidf_matrix)
print(similarity_scores)
2、使用Whoosh进行搜索
使用Whoosh可以方便地进行搜索,并返回相关的文档。
from whoosh.qparser import QueryParser
打开索引
ix = index.open_dir(index_dir)
查询
with ix.searcher() as searcher:
query = QueryParser("content", ix.schema).parse("first document")
results = searcher.search(query)
for result in results:
print(result['title'])
四、展示搜索结果
最后一步是展示搜索结果。可以使用Flask、Django等Web框架来创建一个简单的Web界面,展示搜索结果。
1、使用Flask展示搜索结果
Flask是一个轻量级的Web框架,适合快速开发Web应用。
from flask import Flask, request, render_template
from whoosh.qparser import QueryParser
from whoosh import index
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/search', methods=['POST'])
def search():
query = request.form['query']
ix = index.open_dir('indexdir')
with ix.searcher() as searcher:
query = QueryParser("content", ix.schema).parse(query)
results = searcher.search(query)
return render_template('results.html', results=results)
if __name__ == '__main__':
app.run(debug=True)
2、创建HTML模板
创建简单的HTML模板来展示搜索界面和结果。
<!-- templates/index.html -->
<!DOCTYPE html>
<html>
<head>
<title>Search Engine</title>
</head>
<body>
<form action="/search" method="post">
<input type="text" name="query" placeholder="Enter your search query">
<input type="submit" value="Search">
</form>
</body>
</html>
<!-- templates/results.html -->
<!DOCTYPE html>
<html>
<head>
<title>Search Results</title>
</head>
<body>
<h1>Search Results</h1>
<ul>
{% for result in results %}
<li>{{ result['title'] }}</li>
{% endfor %}
</ul>
</body>
</html>
通过以上步骤,你已经创建了一个简单的Python页面搜索引擎。从抓取网页内容、处理和索引数据,到实现搜索算法和展示搜索结果,整个过程使用了Python的多种库和技术。根据需要,你可以进一步优化和扩展搜索引擎的功能,如添加更多的搜索算法、优化性能、支持更多的网页格式等。
相关问答FAQs:
如何使用Python构建一个简单的页面搜索引擎?
构建一个页面搜索引擎可以通过使用Python的网络请求和解析库来完成。你可以使用requests
库获取网页内容,使用BeautifulSoup
来解析HTML。首先,安装这两个库,然后抓取你感兴趣的网站,提取出页面中的文本信息,并将其存储在一个数据结构中。为了实现搜索功能,可以使用字符串匹配或更复杂的文本检索算法(如TF-IDF)来找到用户查询的相关结果。
在Python中实现网页爬虫需要注意哪些问题?
在实现网页爬虫时,遵循网站的robots.txt
协议是非常重要的,这样可以确保你不会违反任何网站的爬取规则。此外,避免发送过于频繁的请求,以免对目标网站造成负担。合理设置请求间隔,使用异常处理来应对网络问题,以及对抓取的数据进行清理和去重,也是维护爬虫健康运行的关键要素。
如何优化Python搜索引擎的搜索速度和准确性?
要优化搜索速度,可以考虑使用索引技术,将抓取到的数据存储在数据库中,利用数据库的搜索能力来加快查询速度。对于准确性,可以实施自然语言处理(NLP)技术,例如使用分词和词干提取,结合关键词权重算法(如BM25)来提升搜索结果的相关性。同时,收集用户反馈和行为数据,可以持续改进搜索算法,从而提升用户体验。
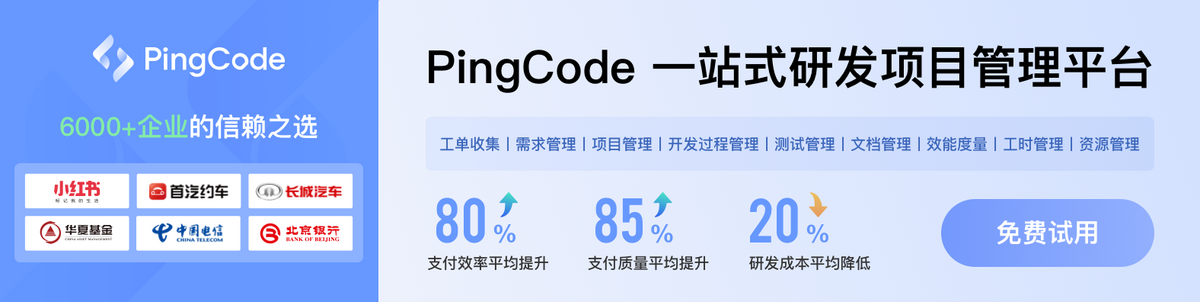