在 Python 中在 C 盘建立文件的方法有多种,比如使用 open()
函数、os
模块或者 pathlib
模块。 其中,常见的方法包括:使用内置的 open()
函数、调用 os
模块中的 makedirs()
和 pathlib
模块中的 Path
类。下面将详细介绍如何使用这些方法在 C 盘建立文件,并结合实际代码示例进行说明。
一、使用 open()
函数创建文件
使用 open()
函数是最常见也是最简单的方法,可以用来打开一个文件,如果文件不存在则会自动创建一个文件。
# 使用 open() 函数在 C 盘创建一个文件
file_path = r"C:\example_file.txt"
with open(file_path, 'w') as file:
file.write("This is an example file.")
在上面的代码中,open()
函数的第一个参数是文件路径,第二个参数 'w'
表示以写入模式打开文件。如果文件不存在,open()
函数会自动创建这个文件。file.write()
用于向文件中写入内容。
二、使用 os
模块创建文件和目录
os
模块提供了与操作系统进行交互的功能,可以用来创建文件和目录。
1. 创建文件
import os
file_path = r"C:\example_file.txt"
with open(file_path, 'w') as file:
file.write("This is an example file created using os module.")
2. 创建目录
如果你需要在 C 盘创建一个目录,然后在这个目录中创建文件,可以使用 os.makedirs()
函数。
import os
创建目录
directory_path = r"C:\example_directory"
if not os.path.exists(directory_path):
os.makedirs(directory_path)
在目录中创建文件
file_path = os.path.join(directory_path, "example_file.txt")
with open(file_path, 'w') as file:
file.write("This is an example file in a new directory.")
三、使用 pathlib
模块创建文件和目录
pathlib
模块提供了面向对象的文件系统路径操作接口,可以用来更加直观地处理文件和目录。
1. 创建文件
from pathlib import Path
file_path = Path(r"C:\example_file.txt")
file_path.write_text("This is an example file created using pathlib module.")
2. 创建目录
如果你需要在 C 盘创建一个目录,然后在这个目录中创建文件,可以使用 Path
类的 mkdir()
方法。
from pathlib import Path
创建目录
directory_path = Path(r"C:\example_directory")
directory_path.mkdir(parents=True, exist_ok=True)
在目录中创建文件
file_path = directory_path / "example_file.txt"
file_path.write_text("This is an example file in a new directory created using pathlib module.")
四、示例:综合应用
下面是一个综合应用示例,展示如何使用 os
模块和 pathlib
模块在 C 盘创建一个目录,并在该目录中创建多个文件。
import os
from pathlib import Path
使用 os 模块创建目录
os_directory_path = r"C:\os_example_directory"
if not os.path.exists(os_directory_path):
os.makedirs(os_directory_path)
在目录中创建文件
os_file_path = os.path.join(os_directory_path, "os_example_file.txt")
with open(os_file_path, 'w') as file:
file.write("This is an example file in a new directory created using os module.")
使用 pathlib 模块创建目录
pathlib_directory_path = Path(r"C:\pathlib_example_directory")
pathlib_directory_path.mkdir(parents=True, exist_ok=True)
在目录中创建文件
pathlib_file_path = pathlib_directory_path / "pathlib_example_file.txt"
pathlib_file_path.write_text("This is an example file in a new directory created using pathlib module.")
在这个示例中,我们首先使用 os
模块创建了一个目录 C:\os_example_directory
,然后在该目录中创建了一个文件 os_example_file.txt
并写入了内容。接下来,我们使用 pathlib
模块创建了另一个目录 C:\pathlib_example_directory
,并在该目录中创建了一个文件 pathlib_example_file.txt
并写入了内容。
通过上述方法,您可以在 Python 中轻松地在 C 盘上创建文件和目录。这些方法不仅简单易用,而且非常灵活,能够满足各种文件系统操作的需求。
相关问答FAQs:
如何在Python中指定C盘路径创建文件?
在Python中,可以通过指定完整的路径来创建文件。使用内置的open()
函数,您可以设置C盘的文件路径,例如:open("C:\\your_folder\\your_file.txt", "w")
。确保您有权限在指定路径下创建文件,并且该文件夹已存在。
创建文件时如何处理可能的错误?
在文件创建过程中,可能会遇到权限问题或文件夹不存在的情况。为了处理这些错误,可以使用try...except
语句来捕获异常。例如:
try:
with open("C:\\your_folder\\your_file.txt", "w") as file:
file.write("Hello, World!")
except IOError as e:
print(f"创建文件时发生错误:{e}")
这样可以确保程序在遇到问题时不会崩溃,并能给出相应的反馈。
在C盘创建文件后,如何验证文件是否成功创建?
在文件创建后,可以使用os.path
模块中的exists()
方法来检查文件是否存在。示例代码如下:
import os
file_path = "C:\\your_folder\\your_file.txt"
if os.path.exists(file_path):
print("文件创建成功!")
else:
print("文件未找到,创建可能失败。")
这种方法能够帮助您确认文件的创建情况。
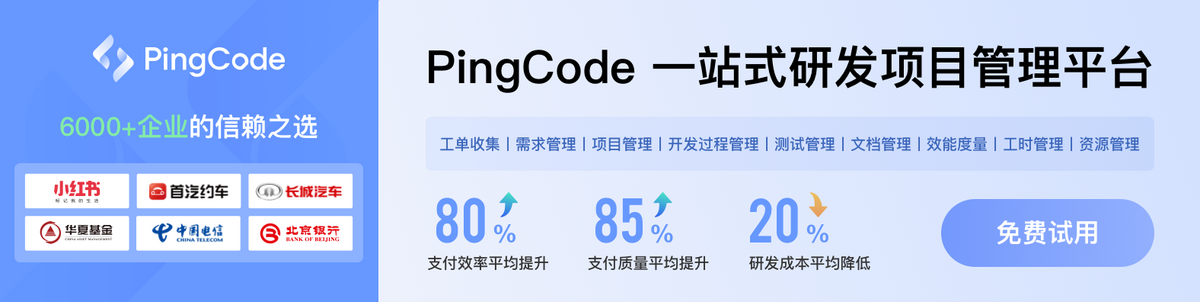