使用Python从字符串中截取整数,主要有三种方法:正则表达式、列表解析、字符串方法。最常用且灵活的是正则表达式。 例如使用re
模块中的findall
函数。
import re
s = "The year is 2023 and the temperature is 20 degrees."
numbers = re.findall(r'\d+', s)
print(numbers) # Output: ['2023', '20']
正则表达式(Regular Expression)是一个强大的工具,用于匹配字符串中的特定模式。re
模块提供了丰富的函数和方法,可以帮助我们轻松地从字符串中提取数字。
一、正则表达式
正则表达式是处理复杂字符串匹配和提取任务的强大工具。在Python中,re
模块提供了全面的正则表达式支持。
1. 使用 re.findall
re.findall
函数可以找到字符串中所有符合特定模式的子串,并返回一个列表。
import re
def extract_integers(s):
return re.findall(r'\d+', s)
s = "The year is 2023 and the temperature is 20 degrees."
numbers = extract_integers(s)
print(numbers) # Output: ['2023', '20']
2. 使用 re.search
re.search
函数查找字符串中第一个符合模式的子串,并返回一个 Match
对象。
import re
def extract_first_integer(s):
match = re.search(r'\d+', s)
if match:
return match.group()
return None
s = "The year is 2023 and the temperature is 20 degrees."
number = extract_first_integer(s)
print(number) # Output: '2023'
3. 使用 re.finditer
re.finditer
函数返回一个迭代器,其中每个元素都是一个 Match
对象,表示字符串中每个符合模式的子串。
import re
def extract_all_integers(s):
return [match.group() for match in re.finditer(r'\d+', s)]
s = "The year is 2023 and the temperature is 20 degrees."
numbers = extract_all_integers(s)
print(numbers) # Output: ['2023', '20']
二、列表解析
列表解析是一种简洁的方式,可以结合字符串方法和条件表达式来提取整数。
1. 使用 str.split
str.split
方法将字符串拆分为一个列表,然后使用列表解析来过滤整数。
def extract_integers_using_split(s):
return [word for word in s.split() if word.isdigit()]
s = "The year is 2023 and the temperature is 20 degrees."
numbers = extract_integers_using_split(s)
print(numbers) # Output: ['2023', '20']
2. 使用 str.isdigit
可以遍历字符串中的每个字符,使用 str.isdigit
方法来检查字符是否为数字。
def extract_integers_using_isdigit(s):
result = []
num = ''
for char in s:
if char.isdigit():
num += char
elif num:
result.append(num)
num = ''
if num:
result.append(num)
return result
s = "The year is 2023 and the temperature is 20 degrees."
numbers = extract_integers_using_isdigit(s)
print(numbers) # Output: ['2023', '20']
三、字符串方法
字符串方法可以用于简单的提取任务,但通常不如正则表达式灵活。
1. 使用 str.translate
str.translate
方法可以删除字符串中的非数字字符,然后使用 str.split
方法提取整数。
def extract_integers_using_translate(s):
table = str.maketrans('', '', 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ ,.!')
return s.translate(table).split()
s = "The year is 2023 and the temperature is 20 degrees."
numbers = extract_integers_using_translate(s)
print(numbers) # Output: ['2023', '20']
2. 使用 filter
filter
函数可以用于筛选出字符串中的数字字符,然后将其连接起来形成整数。
def extract_integers_using_filter(s):
result = []
num = ''
for char in s:
if char.isdigit():
num += char
elif num:
result.append(num)
num = ''
if num:
result.append(num)
return result
s = "The year is 2023 and the temperature is 20 degrees."
numbers = extract_integers_using_filter(s)
print(numbers) # Output: ['2023', '20']
结论
从字符串中截取整数可以通过多种方式实现,具体方法的选择取决于任务的复杂性和字符串的结构。对于大多数情况,正则表达式是最灵活和强大的工具。列表解析和字符串方法适用于简单的提取任务。无论选择哪种方法,都需要考虑代码的可读性和性能,以确保提取操作高效且易于维护。
相关问答FAQs:
如何在Python中从字符串中提取整数?
在Python中,提取字符串中的整数可以使用正则表达式(re
模块)或者简单的字符串处理方法。使用正则表达式可以方便地匹配所有数字,而使用字符串方法则可以逐字符检查并提取数字。以下是一个示例:
import re
string = "在2023年,Python非常流行。"
numbers = re.findall(r'\d+', string)
print(numbers) # 输出:['2023']
是否可以从字符串中提取多个整数?
当然可以。使用正则表达式re.findall()
可以提取字符串中的所有整数。例如,输入字符串"我有3个苹果和5个橙子"可以提取出3和5,返回结果为['3', '5']
。如果需要将其转换为整数类型,可以使用map(int, numbers)
进行转换。
如何处理字符串中不存在整数的情况?
如果字符串中没有整数,re.findall()
将返回一个空列表。可以通过检查返回的结果来判断是否成功提取到整数。在实际应用中,可以根据需求选择适当的处理方式,比如返回默认值或抛出异常。示例代码如下:
string = "没有数字"
numbers = re.findall(r'\d+', string)
if not numbers:
print("没有找到任何整数。")
else:
print(numbers)
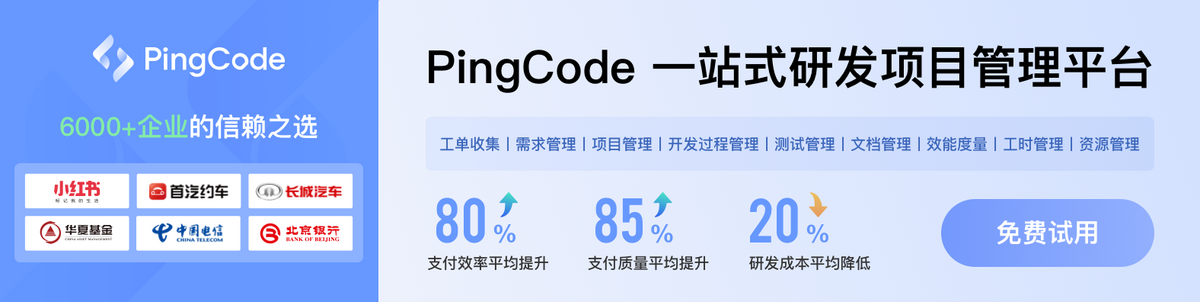