一、直接使用Python内置的文件操作方法如open()、read()、readlines()函数来在文件中搜索、使用正则表达式模块(re)来进行复杂的搜索、使用第三方库如PyPDF2、docx来搜索不同类型的文件。在详细的搜索中,正则表达式提供了强大的匹配能力,可以用于从简单到复杂的各种搜索需求,例如找到特定模式的文本。
Python提供了多种方法来搜索文件中的内容,这包括使用内置的文件操作方法和正则表达式模块,以及利用第三方库来处理不同类型的文件。正则表达式是一个特别强大的工具,可以帮助你在文本中找到特定的模式,从而实现复杂的搜索需求。
二、使用内置文件操作方法
Python提供了简单易用的文件操作方法,这些方法可以帮助你在文件中搜索特定的内容。
1、使用open()函数
open()
函数是Python中最基本的文件操作函数,它可以用来打开一个文件并返回一个文件对象。
with open('example.txt', 'r') as file:
content = file.read()
if 'search_term' in content:
print('Found!')
这段代码打开一个名为example.txt
的文件,并读取其内容。如果content
中包含search_term
,则打印Found!
。
2、使用readlines()函数
readlines()
函数将文件中的每一行作为一个列表元素返回,这样可以逐行进行搜索。
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
if 'search_term' in line:
print('Found!')
这种方法适用于文件内容较大且需要逐行处理的情况。
三、使用正则表达式(re模块)
正则表达式是一个强大的工具,可以帮助你匹配复杂的文本模式。
1、基本使用
re
模块提供了多个函数,例如re.search()
、re.findall()
等,可以用来搜索字符串中的模式。
import re
with open('example.txt', 'r') as file:
content = file.read()
if re.search(r'\bsearch_term\b', content):
print('Found!')
2、使用re.findall()
re.findall()
函数会返回所有匹配的子字符串,这在需要找到多个匹配项时非常有用。
import re
with open('example.txt', 'r') as file:
content = file.read()
matches = re.findall(r'\bsearch_term\b', content)
for match in matches:
print('Found:', match)
四、使用第三方库
对于不同类型的文件,例如PDF、Word文档等,Python提供了多个第三方库来处理这些文件。
1、使用PyPDF2库搜索PDF文件
PyPDF2
是一个流行的PDF处理库,可以用来读取和操作PDF文件。
import PyPDF2
with open('example.pdf', 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
for page_num in range(reader.numPages):
page = reader.getPage(page_num)
text = page.extractText()
if 'search_term' in text:
print(f'Found on page {page_num + 1}')
2、使用python-docx库搜索Word文档
python-docx
是一个用于处理Word文档的库。
from docx import Document
doc = Document('example.docx')
for para in doc.paragraphs:
if 'search_term' in para.text:
print('Found!')
五、结合多种方法
在实际应用中,可能需要结合多种方法来实现更复杂的搜索需求。例如,你可以先用内置的文件操作方法读取文件内容,然后使用正则表达式进行复杂的模式匹配,最后使用第三方库处理特定类型的文件。
1、综合示例
import re
from docx import Document
import PyPDF2
def search_in_text_file(file_path, search_term):
with open(file_path, 'r') as file:
content = file.read()
if re.search(r'\b{}\b'.format(re.escape(search_term)), content):
print(f'Found in {file_path}')
def search_in_pdf_file(file_path, search_term):
with open(file_path, 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
for page_num in range(reader.numPages):
page = reader.getPage(page_num)
text = page.extractText()
if search_term in text:
print(f'Found in {file_path} on page {page_num + 1}')
def search_in_docx_file(file_path, search_term):
doc = Document(file_path)
for para in doc.paragraphs:
if search_term in para.text:
print(f'Found in {file_path}')
def search_files(file_paths, search_term):
for file_path in file_paths:
if file_path.endswith('.txt'):
search_in_text_file(file_path, search_term)
elif file_path.endswith('.pdf'):
search_in_pdf_file(file_path, search_term)
elif file_path.endswith('.docx'):
search_in_docx_file(file_path, search_term)
示例文件路径列表
file_paths = ['example.txt', 'example.pdf', 'example.docx']
search_term = 'search_term'
search_files(file_paths, search_term)
这个综合示例展示了如何在不同类型的文件中搜索特定内容,包括文本文件、PDF文件和Word文档。
六、优化搜索性能
在处理大文件或需要进行大量搜索时,优化搜索性能是非常重要的。
1、使用生成器逐行读取文件
生成器可以帮助你在处理大文件时节省内存。
def search_in_large_file(file_path, search_term):
with open(file_path, 'r') as file:
for line in file:
if search_term in line:
print('Found!')
2、并行搜索
对于大量文件,可以使用并行搜索来加快搜索速度。例如,你可以使用concurrent.futures
模块来实现多线程或多进程搜索。
import concurrent.futures
def search_file(file_path, search_term):
if file_path.endswith('.txt'):
search_in_text_file(file_path, search_term)
elif file_path.endswith('.pdf'):
search_in_pdf_file(file_path, search_term)
elif file_path.endswith('.docx'):
search_in_docx_file(file_path, search_term)
file_paths = ['example1.txt', 'example2.pdf', 'example3.docx']
search_term = 'search_term'
with concurrent.futures.ThreadPoolExecutor() as executor:
executor.map(lambda file_path: search_file(file_path, search_term), file_paths)
这个示例展示了如何使用多线程并行搜索多个文件,从而提高搜索效率。
七、总结
在Python中搜索文件内容有多种方法,包括使用内置的文件操作方法、正则表达式和第三方库。根据不同的文件类型和搜索需求,可以选择最合适的方法来实现高效的搜索。正则表达式提供了强大的匹配能力,可以处理复杂的搜索需求;而对于特定类型的文件,例如PDF和Word文档,使用相应的第三方库可以大大简化操作。通过结合多种方法和优化搜索性能,你可以实现更高效、更精确的搜索。
相关问答FAQs:
如何在Python中读取文件内容进行搜索?
在Python中,可以使用内置的open()
函数读取文件内容。读取文件后,可以使用字符串方法如in
或find()
来查找特定的关键词。例如,可以将文件内容加载到一个字符串中,然后使用if keyword in content
来判断关键词是否存在。
有没有推荐的Python库可以帮助文件搜索?
是的,os
和fnmatch
库可以帮助在文件系统中搜索特定类型的文件。此外,re
库可以用于正则表达式搜索,使得查找更为灵活和强大。使用这些库可以轻松实现对文件内容的深度搜索。
如何优化文件搜索的效率?
在处理大文件时,可以考虑逐行读取文件,而不是一次性加载整个文件。这可以通过使用with open(filename) as file:
语句实现。这样可以在内存使用上更加高效,同时也能提高搜索速度,尤其是在大文件中查找时。
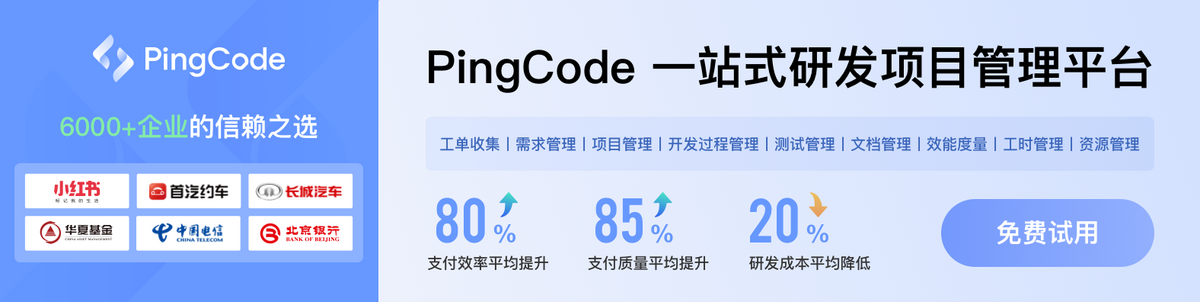