使用Python制作一个题库的步骤包括:定义数据结构、设计数据录入程序、开发题库查询功能、实现题库管理功能(如添加、删除、修改题目)等。 其中,数据结构的设计是最重要的,因为它直接影响到后续程序开发的便利性和运行效率。
下面我们将详细介绍如何用Python制作一个题库,具体包括数据结构设计、题目录入、题库查询、题库管理等方面。
一、数据结构设计
在制作题库之前,首先需要设计一个合理的数据结构,以便存储和管理题目。题库通常包含题目、答案、题目类型、难度等级等信息。我们可以使用Python中的字典和列表来存储这些信息。
# 题库数据结构示例
question_bank = [
{
'id': 1,
'question': 'What is the capital of France?',
'answer': 'Paris',
'type': 'Geography',
'difficulty': 'Easy'
},
{
'id': 2,
'question': 'What is the square root of 64?',
'answer': '8',
'type': 'Math',
'difficulty': 'Medium'
}
]
二、题目录入
为了能够将题目录入题库,我们需要开发一个程序,用于接收用户输入的题目信息,并将其添加到题库中。
def add_question(question_bank):
question_id = len(question_bank) + 1
question = input('Enter the question: ')
answer = input('Enter the answer: ')
question_type = input('Enter the type: ')
difficulty = input('Enter the difficulty (Easy, Medium, Hard): ')
new_question = {
'id': question_id,
'question': question,
'answer': answer,
'type': question_type,
'difficulty': difficulty
}
question_bank.append(new_question)
print('Question added successfully!')
示例调用
add_question(question_bank)
三、题库查询
为了方便用户查询题库中的题目,我们可以开发一个查询功能,允许用户根据不同的条件(如题目类型、难度等级等)查询题目。
def search_questions(question_bank, search_type=None, search_difficulty=None):
results = []
for question in question_bank:
if search_type and question['type'] != search_type:
continue
if search_difficulty and question['difficulty'] != search_difficulty:
continue
results.append(question)
return results
示例调用
search_results = search_questions(question_bank, search_type='Math', search_difficulty='Medium')
for question in search_results:
print(f"ID: {question['id']}, Question: {question['question']}")
四、题库管理
除了添加和查询题目,题库管理还包括删除题目和修改题目信息。我们可以开发相应的函数来实现这些功能。
1、删除题目
def delete_question(question_bank, question_id):
for question in question_bank:
if question['id'] == question_id:
question_bank.remove(question)
print('Question deleted successfully!')
return
print('Question not found!')
示例调用
delete_question(question_bank, 1)
2、修改题目信息
def update_question(question_bank, question_id, new_question=None, new_answer=None, new_type=None, new_difficulty=None):
for question in question_bank:
if question['id'] == question_id:
if new_question:
question['question'] = new_question
if new_answer:
question['answer'] = new_answer
if new_type:
question['type'] = new_type
if new_difficulty:
question['difficulty'] = new_difficulty
print('Question updated successfully!')
return
print('Question not found!')
示例调用
update_question(question_bank, 2, new_question='What is 8 squared?', new_answer='64')
五、导入和导出题库
为了方便题库的保存和共享,我们可以添加导入和导出题库的功能。这里我们使用JSON格式来存储题库数据。
1、导出题库
import json
def export_question_bank(question_bank, filename='question_bank.json'):
with open(filename, 'w') as file:
json.dump(question_bank, file)
print('Question bank exported successfully!')
示例调用
export_question_bank(question_bank)
2、导入题库
def import_question_bank(filename='question_bank.json'):
with open(filename, 'r') as file:
question_bank = json.load(file)
print('Question bank imported successfully!')
return question_bank
示例调用
question_bank = import_question_bank()
六、用户交互界面
为了提供更好的用户体验,我们可以开发一个简单的用户交互界面,允许用户通过菜单选项来执行不同的操作。
def main():
question_bank = []
while True:
print("\nQuestion Bank Menu")
print("1. Add Question")
print("2. Search Questions")
print("3. Delete Question")
print("4. Update Question")
print("5. Export Question Bank")
print("6. Import Question Bank")
print("7. Exit")
choice = input("Enter your choice: ")
if choice == '1':
add_question(question_bank)
elif choice == '2':
search_type = input("Enter question type to search (or leave blank): ")
search_difficulty = input("Enter question difficulty to search (or leave blank): ")
results = search_questions(question_bank, search_type, search_difficulty)
for question in results:
print(f"ID: {question['id']}, Question: {question['question']}")
elif choice == '3':
question_id = int(input("Enter question ID to delete: "))
delete_question(question_bank, question_id)
elif choice == '4':
question_id = int(input("Enter question ID to update: "))
new_question = input("Enter new question (or leave blank): ")
new_answer = input("Enter new answer (or leave blank): ")
new_type = input("Enter new type (or leave blank): ")
new_difficulty = input("Enter new difficulty (or leave blank): ")
update_question(question_bank, question_id, new_question, new_answer, new_type, new_difficulty)
elif choice == '5':
filename = input("Enter filename to export (or leave blank for default): ")
if not filename:
filename = 'question_bank.json'
export_question_bank(question_bank, filename)
elif choice == '6':
filename = input("Enter filename to import (or leave blank for default): ")
if not filename:
filename = 'question_bank.json'
question_bank = import_question_bank(filename)
elif choice == '7':
break
else:
print("Invalid choice, please try again.")
if __name__ == '__main__':
main()
通过以上步骤,我们可以使用Python制作一个简单的题库。这个题库支持题目录入、题库查询、题库管理、导入导出等功能,并提供了一个简单的用户交互界面。你可以根据实际需求进一步扩展和完善这个程序。
相关问答FAQs:
如何选择合适的数据库来存储题库数据?
选择数据库时,可以考虑使用SQLite、MySQL或PostgreSQL等,根据项目规模和需求来决定。如果题库较小,SQLite是个不错的选择,简单易用且无需设置服务器。而对于大型题库项目,MySQL或PostgreSQL提供更强大的功能和并发处理能力。
在Python中如何实现题目的增删改查功能?
可以利用Python的ORM框架如SQLAlchemy或Django ORM来实现题目的增删改查(CRUD)功能。这些框架提供了简单易用的接口,能够轻松地与数据库进行交互。同时,确保在数据操作时进行必要的输入验证,以提高数据的安全性和完整性。
如何为题库添加用户交互功能?
用户交互功能可以通过构建一个简单的命令行界面或图形用户界面(GUI)来实现。使用Python的Tkinter库可以创建GUI,或者使用Flask或Django构建一个Web应用,让用户能够方便地浏览题目、提交答案和查看成绩等。这些交互功能将大大提升用户体验,使题库更加实用。
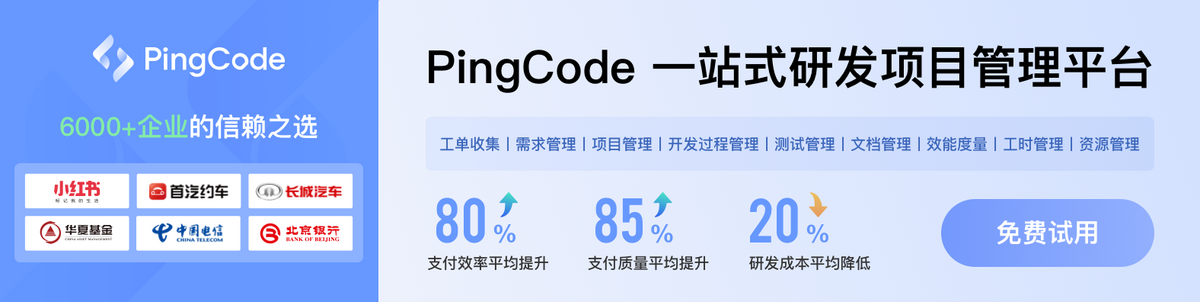