Python三角函数调用方法
在Python中,可以通过内置的math
模块来调用三角函数。常用的三角函数包括正弦(sin)、余弦(cos)和正切(tan)。这些函数可以直接通过导入math
模块并调用相关函数来实现。例如,使用math.sin()
计算正弦、使用math.cos()
计算余弦、使用math.tan()
计算正切。下面我们将详细介绍如何在Python中使用这些函数,以及一些相关的使用技巧和注意事项。
一、导入math模块
在使用任何数学函数之前,首先需要导入math
模块。可以通过以下代码实现:
import math
导入模块后,就可以使用math
模块中的各种数学函数了。
二、计算正弦值(sin)
正弦函数是一个非常常用的三角函数,用来计算一个角度的正弦值。在Python中,可以使用math.sin()
函数来计算正弦值。该函数接受一个弧度值作为参数,并返回该角度的正弦值。例如:
import math
angle_rad = math.radians(30) # 将角度转换为弧度
sin_value = math.sin(angle_rad)
print(f"The sine of 30 degrees is {sin_value}")
在上述示例中,我们首先使用math.radians()
函数将角度从度数转换为弧度,然后传递给math.sin()
函数来计算正弦值。
三、计算余弦值(cos)
余弦函数也是一个常用的三角函数,用来计算一个角度的余弦值。可以使用math.cos()
函数来计算余弦值。该函数同样接受一个弧度值作为参数,并返回该角度的余弦值。例如:
import math
angle_rad = math.radians(60) # 将角度转换为弧度
cos_value = math.cos(angle_rad)
print(f"The cosine of 60 degrees is {cos_value}")
同样,我们先将角度从度数转换为弧度,再传递给math.cos()
函数来计算余弦值。
四、计算正切值(tan)
正切函数用于计算一个角度的正切值。可以使用math.tan()
函数来计算正切值。该函数接受一个弧度值作为参数,并返回该角度的正切值。例如:
import math
angle_rad = math.radians(45) # 将角度转换为弧度
tan_value = math.tan(angle_rad)
print(f"The tangent of 45 degrees is {tan_value}")
在这个示例中,我们将角度从度数转换为弧度,然后传递给math.tan()
函数来计算正切值。
五、反三角函数的使用
除了基本的三角函数,math
模块还提供了反三角函数,用于计算一个值对应的角度。例如,math.asin()
用于计算反正弦,math.acos()
用于计算反余弦,math.atan()
用于计算反正切。它们的返回值是以弧度表示的角度。示例如下:
import math
value = 0.5
asin_value = math.asin(value)
acos_value = math.acos(value)
atan_value = math.atan(value)
print(f"The arcsine of 0.5 is {math.degrees(asin_value)} degrees")
print(f"The arccosine of 0.5 is {math.degrees(acos_value)} degrees")
print(f"The arctangent of 0.5 is {math.degrees(atan_value)} degrees")
在上述示例中,反三角函数的返回值通过math.degrees()
函数转换为度数,以便更直观地理解。
六、处理弧度与度数的转换
在使用三角函数时,可能需要在弧度和度数之间进行转换。math
模块提供了两个函数,math.radians()
和math.degrees()
,分别用于将度数转换为弧度和将弧度转换为度数。例如:
import math
angle_deg = 90
angle_rad = math.radians(angle_deg) # 将度数转换为弧度
converted_back_deg = math.degrees(angle_rad) # 将弧度转换回度数
print(f"{angle_deg} degrees is {angle_rad} radians")
print(f"{angle_rad} radians is {converted_back_deg} degrees")
通过这些转换函数,可以方便地在弧度和度数之间切换,确保计算的准确性。
七、使用三角函数进行实际应用
三角函数在实际应用中非常广泛,例如计算三角形的边长、角度、物理模拟等。下面是一个使用三角函数计算直角三角形斜边长度的示例:
import math
def calculate_hypotenuse(a, b):
return math.sqrt(a<strong>2 + b</strong>2)
a = 3
b = 4
hypotenuse = calculate_hypotenuse(a, b)
print(f"The hypotenuse of a right triangle with sides {a} and {b} is {hypotenuse}")
在这个示例中,我们定义了一个函数calculate_hypotenuse()
,使用毕达哥拉斯定理计算直角三角形的斜边长度。
八、三角函数的绘图应用
三角函数在数据可视化中也非常有用。例如,可以使用matplotlib
库绘制三角函数的图像。以下是一个绘制正弦函数图像的示例:
import math
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 2 * math.pi, 1000) # 生成从0到2π的1000个点
y = np.sin(x) # 计算每个点的正弦值
plt.plot(x, y)
plt.title("Sine Function")
plt.xlabel("x (radians)")
plt.ylabel("sin(x)")
plt.grid(True)
plt.show()
在这个示例中,我们使用numpy
生成一系列从0到2π的点,然后使用math.sin()
计算每个点的正弦值,并使用matplotlib
绘制图像。
九、三角函数的性能优化
在一些性能敏感的应用中,可能需要优化三角函数的计算速度。可以使用预计算和查找表的方法来提高性能。例如,预先计算常用角度的三角函数值并存储在一个数组中,使用时直接查找即可:
import math
预计算常用角度的正弦值
sin_table = {angle: math.sin(math.radians(angle)) for angle in range(0, 361)}
def fast_sin(angle):
return sin_table[angle % 360]
angle = 30
sin_value = fast_sin(angle)
print(f"The fast sine of {angle} degrees is {sin_value}")
在这个示例中,我们预先计算了0到360度的正弦值,并存储在一个字典中。通过查找表来获取正弦值,比每次计算都调用math.sin()
更快。
十、总结
在Python中,调用三角函数非常简单,只需导入math
模块并使用相关函数即可。常用的三角函数包括math.sin()
、math.cos()
和math.tan()
,而反三角函数包括math.asin()
、math.acos()
和math.atan()
。此外,还可以使用math.radians()
和math.degrees()
进行弧度和度数的转换。通过这些函数,可以轻松实现各种三角函数的计算,并应用于实际问题中。希望本文能帮助你更好地理解和使用Python中的三角函数。
相关问答FAQs:
如何在Python中使用三角函数库?
在Python中,三角函数主要通过math
模块来实现。你可以使用import math
导入这个模块,然后调用如math.sin()
, math.cos()
, 和 math.tan()
等函数来计算正弦、余弦和正切值。确保输入的角度是以弧度为单位,如果需要将角度转换为弧度,可以使用math.radians()
函数。
Python中的三角函数支持哪些角度单位?
Python的math
模块主要使用弧度作为角度单位。如果你想使用度数,可以利用math.radians()
将度数转换为弧度。例如,math.sin(math.radians(30))
可以计算30度的正弦值。相反,如果你需要将弧度转换为度数,可以使用math.degrees()
函数。
在Python中如何处理三角函数的反函数?
Python的math
模块还提供了一些三角函数的反函数,如math.asin()
, math.acos()
, 和 math.atan()
,它们分别用于计算反正弦、反余弦和反正切值。这些函数的返回值也是以弧度为单位,如果需要度数,可以用math.degrees()
进行转换。例如,math.degrees(math.asin(0.5))
会返回30度。
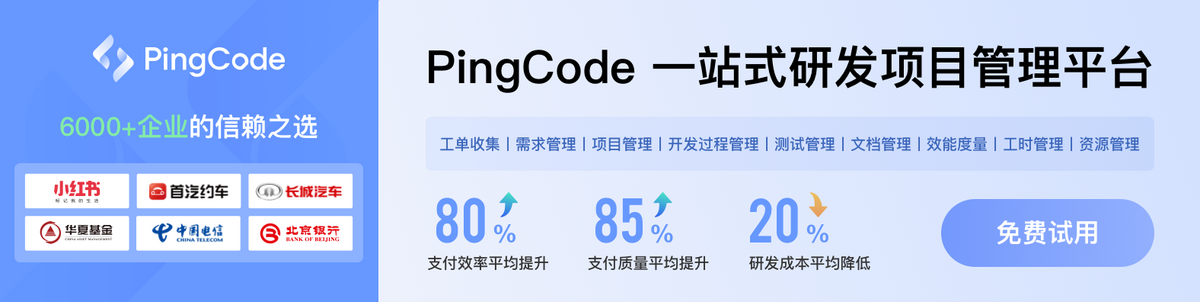