在Python中,表示字符串的方法包括使用单引号、双引号、三重引号、转义字符等。 其中最常用的方式是使用单引号和双引号。三重引号主要用于表示多行字符串,而转义字符则用于在字符串中表示特殊字符。接下来,我们将详细介绍每种方法及其使用场景。
一、单引号和双引号
Python允许使用单引号 ('
) 和双引号 ("
) 来表示字符串。这两个引号可以互换使用,没有本质的区别。选择使用哪种引号主要取决于字符串内容中是否包含其他引号。
# 使用单引号表示字符串
single_quote_str = 'Hello, World!'
print(single_quote_str)
使用双引号表示字符串
double_quote_str = "Hello, World!"
print(double_quote_str)
当字符串中包含单引号时,使用双引号
str_with_single_quote = "It's a beautiful day!"
print(str_with_single_quote)
当字符串中包含双引号时,使用单引号
str_with_double_quote = 'He said, "Hello, World!"'
print(str_with_double_quote)
二、三重引号
三重引号('''
或 """
)用于表示多行字符串或包含复杂格式的字符串。在多行字符串中,可以保留换行符和空格,这对于表示长文本或文档字符串(docstring)非常有用。
# 使用三重单引号表示多行字符串
multi_line_str_single = '''This is a string
that spans multiple
lines.'''
print(multi_line_str_single)
使用三重双引号表示多行字符串
multi_line_str_double = """This is another string
that also spans multiple
lines."""
print(multi_line_str_double)
三、转义字符
转义字符是由反斜杠()引导的字符,用于表示一些特殊字符。例如,
\n
表示换行符,\t
表示制表符,\\
表示反斜杠本身。转义字符使得在字符串中包含特殊字符变得可能。
# 换行符
str_with_newline = "Hello,\nWorld!"
print(str_with_newline)
制表符
str_with_tab = "Hello,\tWorld!"
print(str_with_tab)
反斜杠
str_with_backslash = "This is a backslash: \\"
print(str_with_backslash)
包含引号
str_with_quotes = "He said, \"Hello, World!\""
print(str_with_quotes)
四、原始字符串
原始字符串(Raw String)通过在字符串前加上字母 r
或 R
来创建。这种字符串会忽略所有的转义字符,因此非常适合用于表示正则表达式或包含大量反斜杠的字符串。
# 原始字符串
raw_str = r"This is a raw string with backslashes: \\"
print(raw_str)
原始字符串表示正则表达式
regex_pattern = r"\d{3}-\d{2}-\d{4}"
print(regex_pattern)
五、格式化字符串
Python 提供了多种方法来格式化字符串,包括旧式的 %
操作符、新式的 str.format()
方法和现代的 f-strings(格式化字符串字面量)。f-strings 是在 Python 3.6 及更高版本中引入的一种简洁且强大的字符串格式化方式。
# 旧式格式化
name = "Alice"
age = 30
old_style = "My name is %s and I am %d years old." % (name, age)
print(old_style)
新式格式化
new_style = "My name is {} and I am {} years old.".format(name, age)
print(new_style)
f-strings
f_string = f"My name is {name} and I am {age} years old."
print(f_string)
六、字符串操作
Python 提供了丰富的字符串操作函数和方法,使得字符串处理变得非常方便。这些操作包括拼接、切片、查找、替换等。
1. 拼接字符串
可以使用 +
操作符或 join()
方法来拼接字符串。
# 使用 + 操作符拼接
str1 = "Hello, "
str2 = "World!"
concatenated_str = str1 + str2
print(concatenated_str)
使用 join() 方法拼接
str_list = ["Hello", "World"]
joined_str = " ".join(str_list)
print(joined_str)
2. 切片字符串
切片操作使得可以从字符串中提取子字符串。切片使用 :
语法,[start:end:step]
。
# 切片操作
s = "Hello, World!"
print(s[0:5]) # 输出 'Hello'
print(s[7:12]) # 输出 'World'
print(s[::2]) # 输出 'Hlo ol!'
3. 查找和替换
可以使用 find()
方法查找子字符串的位置,使用 replace()
方法替换子字符串。
# 查找子字符串
s = "Hello, World!"
position = s.find("World")
print(position) # 输出 7
替换子字符串
replaced_str = s.replace("World", "Python")
print(replaced_str) # 输出 'Hello, Python!'
4. 大小写转换
可以使用 upper()
方法将字符串转换为大写,使用 lower()
方法将字符串转换为小写。
# 大写转换
s = "Hello, World!"
upper_str = s.upper()
print(upper_str) # 输出 'HELLO, WORLD!'
小写转换
lower_str = s.lower()
print(lower_str) # 输出 'hello, world!'
七、字符串内置方法
Python 提供了许多字符串内置方法,使得字符串处理更加便捷。这些方法包括 strip()
、split()
、join()
、startswith()
、endswith()
等。
1. 去除空白字符
可以使用 strip()
、lstrip()
、rstrip()
方法去除字符串两端或一端的空白字符。
# 去除空白字符
s = " Hello, World! "
stripped_str = s.strip()
print(stripped_str) # 输出 'Hello, World!'
去除左侧空白字符
lstripped_str = s.lstrip()
print(lstripped_str) # 输出 'Hello, World! '
去除右侧空白字符
rstripped_str = s.rstrip()
print(rstripped_str) # 输出 ' Hello, World!'
2. 分割字符串
可以使用 split()
方法根据指定分隔符分割字符串。
# 分割字符串
s = "Hello, World!"
split_str = s.split(", ")
print(split_str) # 输出 ['Hello', 'World!']
3. 判断字符串的开始和结束
可以使用 startswith()
和 endswith()
方法判断字符串是否以指定子字符串开始或结束。
# 判断字符串的开始
s = "Hello, World!"
print(s.startswith("Hello")) # 输出 True
print(s.startswith("World")) # 输出 False
判断字符串的结束
print(s.endswith("World!")) # 输出 True
print(s.endswith("Hello")) # 输出 False
八、字符串的编码和解码
在处理不同编码的字符串时,可以使用 encode()
方法将字符串编码为字节对象,使用 decode()
方法将字节对象解码为字符串。
# 编码字符串
s = "Hello, World!"
encoded_str = s.encode("utf-8")
print(encoded_str) # 输出 b'Hello, World!'
解码字节对象
decoded_str = encoded_str.decode("utf-8")
print(decoded_str) # 输出 'Hello, World!'
九、字符串的其他操作
Python 还提供了其他许多有用的字符串操作方法,例如 count()
用于统计子字符串出现的次数,find()
和 rfind()
用于查找子字符串的位置,format()
用于字符串格式化等。
# 统计子字符串出现的次数
s = "Hello, World! Hello, Python!"
count = s.count("Hello")
print(count) # 输出 2
查找子字符串的位置
position = s.find("Python")
print(position) # 输出 20
反向查找子字符串的位置
rposition = s.rfind("Hello")
print(rposition) # 输出 13
十、字符串的对齐操作
Python 提供了对齐字符串的方法,包括 center()
、ljust()
和 rjust()
。
# 字符串居中对齐
s = "Hello"
centered_str = s.center(20, "-")
print(centered_str) # 输出 '-------Hello--------'
字符串左对齐
left_justified_str = s.ljust(20, "-")
print(left_justified_str) # 输出 'Hello---------------'
字符串右对齐
right_justified_str = s.rjust(20, "-")
print(right_justified_str) # 输出 '---------------Hello'
结论
在Python中表示字符串的方法多种多样,包括使用单引号、双引号、三重引号以及转义字符等。不同的方法适用于不同的场景,例如单引号和双引号适用于简单字符串,三重引号适用于多行字符串,转义字符用于表示特殊字符。此外,Python提供了丰富的字符串操作方法,包括拼接、切片、查找、替换、格式化等,使得字符串处理变得非常方便。掌握这些方法和操作,将极大地提升编写Python代码的效率和灵活性。
相关问答FAQs:
在Python中,字符串可以用哪些方式定义?
在Python中,字符串可以用单引号(')、双引号(")或三重引号('''或""")来定义。单引号和双引号的使用没有区别,选择哪一种主要取决于个人偏好或字符串内容。如果字符串中包含引号,使用不同类型的引号可以避免转义。例如,字符串"Hello, I'm Python!"使用双引号定义,而'He said, "Hello!"'则使用单引号定义。三重引号特别适合用于多行字符串。
如何在Python中处理字符串中的转义字符?
在Python中,转义字符以反斜杠(\)开头,能够在字符串中插入特殊字符。例如,使用'可以在单引号字符串中插入单引号,使用\n可以插入换行符。处理转义字符时,确保了解它们的用途,以便正确显示字符串内容。
如何在Python中对字符串进行操作和处理?
Python提供了多种字符串操作方法。例如,可以使用len()
函数获取字符串长度,使用str.lower()
和str.upper()
方法进行大小写转换,使用str.split()
方法将字符串拆分成列表。此外,字符串还支持拼接,使用+
操作符可以将多个字符串连接在一起。了解这些基本操作将帮助用户高效地处理和格式化字符串。
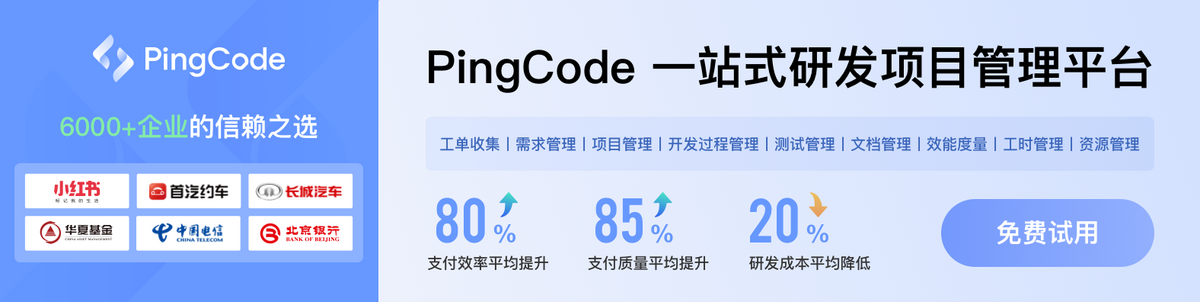