在Python中,可以通过几种方法将多个文本文件拼接在一起,包括使用文件操作、字符串操作和一些内置模块。最常用的方法包括:使用文件读取和写入操作、通过字符串操作将内容拼接在一起、使用os
模块处理文件路径等。下面将详细介绍这些方法,并提供示例代码。
一、使用文件读取和写入操作
这种方法是最直接的,通过读取多个文件的内容并将其写入一个新的文件中。以下是具体步骤:
- 打开目标文件(用于写入拼接后的内容)。
- 依次打开需要拼接的文件,读取它们的内容。
- 将读取的内容写入目标文件。
- 关闭所有文件。
下面是具体的代码示例:
# 指定需要拼接的文件列表
file_list = ['file1.txt', 'file2.txt', 'file3.txt']
指定目标文件
output_file = 'merged_file.txt'
打开目标文件
with open(output_file, 'w') as outfile:
# 依次打开需要拼接的文件并读取内容
for file_name in file_list:
with open(file_name, 'r') as infile:
content = infile.read()
outfile.write(content)
# 添加换行符,保证文件内容之间有分隔
outfile.write('\n')
二、使用字符串操作
如果文件内容较小,可以将所有内容读取到字符串中进行拼接,然后一次性写入目标文件。以下是具体步骤:
- 读取所有文件内容,并将其拼接成一个字符串。
- 将拼接后的字符串写入目标文件。
下面是具体的代码示例:
# 指定需要拼接的文件列表
file_list = ['file1.txt', 'file2.txt', 'file3.txt']
指定目标文件
output_file = 'merged_file.txt'
定义一个空字符串用于存储拼接后的内容
merged_content = ''
依次打开需要拼接的文件并读取内容
for file_name in file_list:
with open(file_name, 'r') as infile:
content = infile.read()
merged_content += content + '\n'
将拼接后的内容写入目标文件
with open(output_file, 'w') as outfile:
outfile.write(merged_content)
三、使用os
模块处理文件路径
在实际项目中,文件可能存储在不同的目录中。可以使用os
模块处理文件路径,确保文件路径正确。以下是具体步骤:
- 使用
os.path.join
拼接文件路径。 - 读取所有文件内容,并将其写入目标文件。
下面是具体的代码示例:
import os
指定文件目录和文件名列表
directory = 'path/to/files'
file_list = ['file1.txt', 'file2.txt', 'file3.txt']
指定目标文件
output_file = 'merged_file.txt'
打开目标文件
with open(output_file, 'w') as outfile:
# 依次打开需要拼接的文件并读取内容
for file_name in file_list:
file_path = os.path.join(directory, file_name)
with open(file_path, 'r') as infile:
content = infile.read()
outfile.write(content)
# 添加换行符,保证文件内容之间有分隔
outfile.write('\n')
四、处理大文件
对于非常大的文件,建议逐行读取和写入,以避免内存不足的问题。以下是具体步骤:
- 逐行读取每个文件的内容。
- 将读取的内容逐行写入目标文件。
下面是具体的代码示例:
# 指定需要拼接的文件列表
file_list = ['file1.txt', 'file2.txt', 'file3.txt']
指定目标文件
output_file = 'merged_file.txt'
打开目标文件
with open(output_file, 'w') as outfile:
# 依次打开需要拼接的文件并逐行读取内容
for file_name in file_list:
with open(file_name, 'r') as infile:
for line in infile:
outfile.write(line)
# 添加换行符,保证文件内容之间有分隔
outfile.write('\n')
五、使用glob
模块批量处理文件
如果需要拼接的文件数量较多且有规律的命名,可以使用glob
模块批量处理文件。以下是具体步骤:
- 使用
glob
模块匹配文件路径。 - 读取所有匹配文件的内容,并将其写入目标文件。
下面是具体的代码示例:
import glob
使用glob模块匹配文件路径
file_list = glob.glob('path/to/files/*.txt')
指定目标文件
output_file = 'merged_file.txt'
打开目标文件
with open(output_file, 'w') as outfile:
# 依次打开需要拼接的文件并读取内容
for file_path in file_list:
with open(file_path, 'r') as infile:
content = infile.read()
outfile.write(content)
# 添加换行符,保证文件内容之间有分隔
outfile.write('\n')
六、使用生成器节省内存
对于非常大的文件,使用生成器可以避免一次性读取大量数据导致内存不足。以下是具体步骤:
- 定义一个生成器函数逐行读取文件内容。
- 逐行写入目标文件。
下面是具体的代码示例:
# 定义生成器函数逐行读取文件内容
def file_line_reader(file_list):
for file_name in file_list:
with open(file_name, 'r') as infile:
for line in infile:
yield line
指定需要拼接的文件列表
file_list = ['file1.txt', 'file2.txt', 'file3.txt']
指定目标文件
output_file = 'merged_file.txt'
打开目标文件
with open(output_file, 'w') as outfile:
# 使用生成器逐行写入目标文件
for line in file_line_reader(file_list):
outfile.write(line)
# 添加换行符,保证文件内容之间有分隔
outfile.write('\n')
七、处理编码问题
在拼接文件时,可能会遇到不同文件的编码不一致的问题。可以使用codecs
模块指定编码进行读取和写入。以下是具体步骤:
- 使用
codecs
模块指定编码打开文件。 - 读取所有文件内容,并将其写入目标文件。
下面是具体的代码示例:
import codecs
指定需要拼接的文件列表
file_list = ['file1.txt', 'file2.txt', 'file3.txt']
指定目标文件
output_file = 'merged_file.txt'
指定文件编码
encoding = 'utf-8'
打开目标文件
with codecs.open(output_file, 'w', encoding) as outfile:
# 依次打开需要拼接的文件并读取内容
for file_name in file_list:
with codecs.open(file_name, 'r', encoding) as infile:
content = infile.read()
outfile.write(content)
# 添加换行符,保证文件内容之间有分隔
outfile.write('\n')
八、使用pandas
库进行数据处理
如果文件内容是表格数据,可以使用pandas
库进行处理。以下是具体步骤:
- 使用
pandas
读取所有文件内容。 - 将所有数据拼接成一个数据框。
- 将拼接后的数据框写入目标文件。
下面是具体的代码示例:
import pandas as pd
指定需要拼接的文件列表
file_list = ['file1.csv', 'file2.csv', 'file3.csv']
指定目标文件
output_file = 'merged_file.csv'
读取所有文件内容并拼接成一个数据框
df_list = [pd.read_csv(file_name) for file_name in file_list]
merged_df = pd.concat(df_list, ignore_index=True)
将拼接后的数据框写入目标文件
merged_df.to_csv(output_file, index=False)
通过以上方法,可以灵活地将多个文本文件拼接在一起。根据具体需求选择合适的方法,确保文件内容的完整性和正确性。希望这些方法和示例代码能够帮助您顺利完成文件拼接任务。
相关问答FAQs:
如何在Python中读取多个文本文件并将其内容拼接在一起?
在Python中,可以使用内置的文件操作功能读取多个文本文件的内容。首先,使用open()
函数打开每个文件,然后使用read()
方法获取文件内容。接着,将所有内容合并成一个字符串,最后可以将拼接后的内容写入到一个新的文本文件中。示例代码如下:
file_names = ['file1.txt', 'file2.txt', 'file3.txt']
combined_content = ''
for file_name in file_names:
with open(file_name, 'r') as file:
combined_content += file.read() + "\n" # 添加换行符以分隔各个文件内容
with open('combined_file.txt', 'w') as output_file:
output_file.write(combined_content)
在拼接文本文件时,如何处理文件编码问题?
在处理文本文件时,编码问题通常会导致读取错误或数据丢失。使用open()
函数时,可以通过encoding
参数指定文件编码,如utf-8
或latin-1
。确保所有文件的编码一致,以避免乱码或读取失败。以下是一个示例:
with open(file_name, 'r', encoding='utf-8') as file:
combined_content += file.read() + "\n"
拼接文本文件后,如何确保输出文件的格式和结构清晰?
为了确保输出文件的格式和结构清晰,可以在拼接时添加分隔符或者标题。例如,可以在每个文件的开头加入文件名作为标题,或在文件之间插入特定的分隔符。这样可以帮助后续阅读和分析拼接后的内容。代码示例:
for file_name in file_names:
combined_content += f'--- {file_name} ---\n' # 添加文件名作为标题
with open(file_name, 'r', encoding='utf-8') as file:
combined_content += file.read() + "\n"
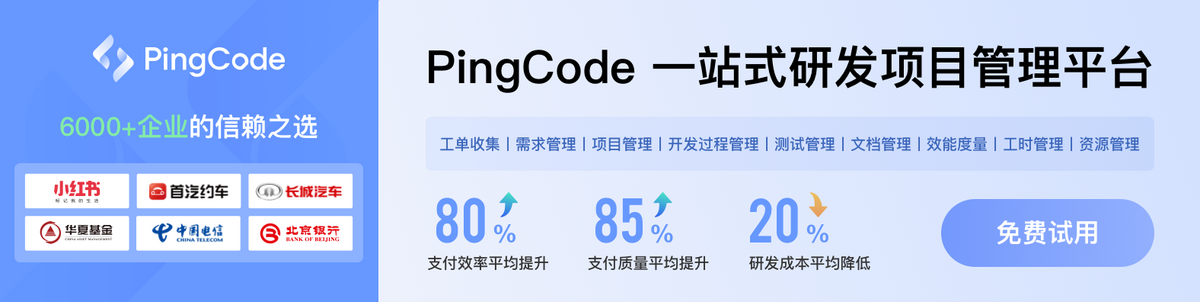