Python抓取网页源代码并保存到本地的步骤包括:使用请求库获取网页内容、保存内容到文件、处理不同类型的网页内容、以及处理异常情况。 下面将详细介绍如何实现这些步骤。
一、安装和导入所需库
在开始之前,需要确保已经安装了必要的Python库。对于网页抓取,我们通常使用requests
库,而对于处理和解析HTML内容,我们可以使用BeautifulSoup
库。首先,确保你已经安装了这些库:
pip install requests
pip install beautifulsoup4
然后在你的Python脚本中导入这些库:
import requests
from bs4 import BeautifulSoup
二、抓取网页源代码
要抓取网页源代码,你需要知道网页的URL。下面是一个基本的例子,展示了如何使用requests
库来获取网页的HTML内容:
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
else:
print(f'Failed to retrieve webpage. Status code: {response.status_code}')
这里,我们使用requests.get()
方法来发送HTTP GET请求,并检查返回的状态码是否为200(成功)。
三、保存网页源代码到本地文件
一旦你获取了网页的HTML内容,就可以将其保存到本地文件中。我们可以使用Python的内置open()
函数来创建和写入文件:
with open('webpage.html', 'w', encoding='utf-8') as file:
file.write(html_content)
这里,我们使用with open()
来打开一个文件,以写入模式('w'
)打开文件,并指定编码为utf-8
,然后将HTML内容写入文件。
四、处理不同类型的网页内容
有时候,你可能需要处理不同类型的网页内容,例如动态网页内容或需要登录的网页。对于动态网页内容,可以使用Selenium
库来模拟浏览器操作:
pip install selenium
然后使用以下代码:
from selenium import webdriver
url = 'http://example.com'
driver = webdriver.Chrome() # 或者使用其他浏览器驱动,例如 Firefox
driver.get(url)
html_content = driver.page_source
driver.quit()
with open('webpage_dynamic.html', 'w', encoding='utf-8') as file:
file.write(html_content)
这里,我们使用Selenium
来打开一个浏览器窗口,访问URL,并获取动态生成的网页内容。
五、处理异常情况
在实际使用过程中,可能会遇到各种异常情况,比如网络连接错误、网页不存在等。因此,最好在代码中添加异常处理机制:
import requests
from requests.exceptions import RequestException
url = 'http://example.com'
try:
response = requests.get(url)
response.raise_for_status()
except RequestException as e:
print(f'Error retrieving webpage: {e}')
else:
with open('webpage.html', 'w', encoding='utf-8') as file:
file.write(response.text)
在这个例子中,我们使用了try
和except
块来捕获和处理请求过程中可能发生的异常。
六、总结
通过上述步骤,你可以使用Python抓取网页源代码并保存到本地文件中。这些步骤包括:安装和导入所需库、抓取网页源代码、保存网页源代码到本地文件、处理不同类型的网页内容、以及处理异常情况。掌握这些技能,你就可以高效地进行网页抓取和数据采集。
相关问答FAQs:
如何使用Python抓取网页源代码并保存为HTML文件?
可以使用Python的requests
库来抓取网页源代码。首先,安装requests
库,然后使用requests.get()
方法获取页面内容。获取到的内容可以通过文件操作将其保存为HTML文件。以下是一个简单的示例代码:
import requests
url = 'http://example.com' # 替换为目标网页URL
response = requests.get(url)
with open('page_source.html', 'w', encoding='utf-8') as file:
file.write(response.text)
在使用Python抓取网页时,如何处理网络请求的错误?
在抓取网页时可能会遇到各种网络错误,如超时或404错误。可以通过使用try-except
语句来捕获这些错误,并根据错误类型做出相应处理。示例代码如下:
import requests
url = 'http://example.com' # 替换为目标网页URL
try:
response = requests.get(url, timeout=10)
response.raise_for_status() # 检查请求是否成功
with open('page_source.html', 'w', encoding='utf-8') as file:
file.write(response.text)
except requests.exceptions.RequestException as e:
print(f"请求错误: {e}")
如何在抓取网页源代码时处理动态内容?
很多网页使用JavaScript动态加载内容,直接抓取HTML可能无法获取这些数据。可以考虑使用Selenium
库,模拟浏览器行为来抓取完整网页内容。使用Selenium
可以控制浏览器加载页面,等待JavaScript执行后,再获取页面源代码。以下是一个示例:
from selenium import webdriver
driver = webdriver.Chrome() # 确保已安装ChromeDriver
driver.get('http://example.com') # 替换为目标网页URL
html = driver.page_source
with open('dynamic_page_source.html', 'w', encoding='utf-8') as file:
file.write(html)
driver.quit()
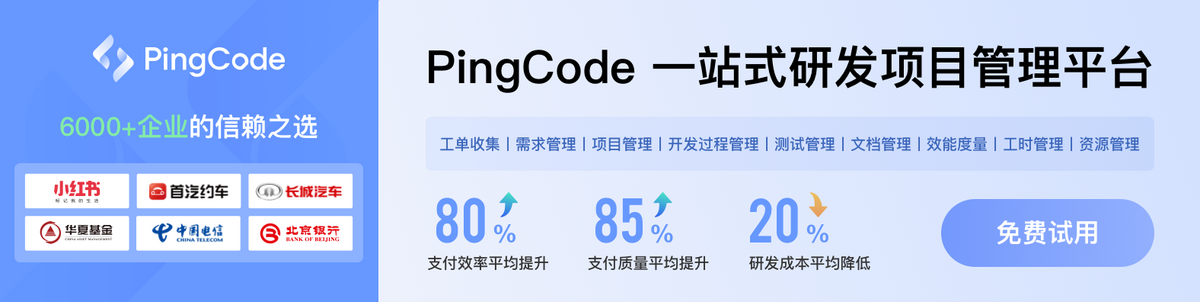