Python求两点特定长路径的方法有:计算欧几里得距离、使用曼哈顿距离、使用网络图算法。 其中,计算欧几里得距离是一种直接的方式,可以通过数学公式计算两点间的直线距离。
欧几里得距离是几何学中最常用的一种距离度量方式,可以在二维或多维空间中计算两点之间的直线距离。欧几里得距离的计算公式如下:
[
d = \sqrt{(x_2 – x_1)^2 + (y_2 – y_1)^2}
]
在Python中,我们可以使用math库中的sqrt函数来实现这个公式。
示例代码:
import math
def euclidean_distance(point1, point2):
return math.sqrt((point2[0] - point1[0])<strong>2 + (point2[1] - point1[1])</strong>2)
point1 = (1, 2)
point2 = (4, 6)
distance = euclidean_distance(point1, point2)
print(f"Euclidean distance: {distance}")
接下来,我们将详细探讨Python中求两点特定长路径的几种方法。
一、欧几里得距离
欧几里得距离不仅可以在二维空间中使用,也可以在三维或更高维的空间中使用。通过扩展公式,我们可以计算多维空间中的距离。
多维欧几里得距离
在多维空间中,欧几里得距离公式为:
[
d = \sqrt{\sum_{i=1}^{n}(x_{2i} – x_{1i})^2}
]
示例代码:
import math
def euclidean_distance_nd(point1, point2):
return math.sqrt(sum((point2[i] - point1[i])2 for i in range(len(point1))))
point1 = (1, 2, 3)
point2 = (4, 6, 8)
distance = euclidean_distance_nd(point1, point2)
print(f"Euclidean distance in 3D: {distance}")
使用NumPy计算欧几里得距离
NumPy库提供了高效的数组运算,可以方便地计算欧几里得距离。使用NumPy的linalg.norm函数可以快速求解。
示例代码:
import numpy as np
def euclidean_distance_np(point1, point2):
return np.linalg.norm(np.array(point2) - np.array(point1))
point1 = np.array([1, 2])
point2 = np.array([4, 6])
distance = euclidean_distance_np(point1, point2)
print(f"Euclidean distance using NumPy: {distance}")
二、曼哈顿距离
曼哈顿距离是一种不同于欧几里得距离的度量方式,它计算的是两点在各个维度上的绝对距离之和。在网格状的路径规划中,曼哈顿距离更为常用。
曼哈顿距离公式为:
[
d = \sum_{i=1}^{n} |x_{2i} – x_{1i}|
]
计算曼哈顿距离
示例代码:
def manhattan_distance(point1, point2):
return sum(abs(point2[i] - point1[i]) for i in range(len(point1)))
point1 = (1, 2)
point2 = (4, 6)
distance = manhattan_distance(point1, point2)
print(f"Manhattan distance: {distance}")
多维曼哈顿距离
同样,曼哈顿距离也可以扩展到多维空间。
示例代码:
def manhattan_distance_nd(point1, point2):
return sum(abs(point2[i] - point1[i]) for i in range(len(point1)))
point1 = (1, 2, 3)
point2 = (4, 6, 8)
distance = manhattan_distance_nd(point1, point2)
print(f"Manhattan distance in 3D: {distance}")
三、网络图算法
在一些复杂的应用场景中,如地图导航、路径规划等,我们需要使用网络图算法来求解两点间的特定路径。常用的网络图算法包括Dijkstra算法、A*算法和Floyd-Warshall算法等。
Dijkstra算法
Dijkstra算法是一种经典的单源最短路径算法,适用于无负权图。它通过贪心策略逐步扩展最短路径。
示例代码:
import heapq
def dijkstra(graph, start, end):
queue = [(0, start)]
distances = {vertex: float('inf') for vertex in graph}
distances[start] = 0
while queue:
current_distance, current_vertex = heapq.heappop(queue)
if current_distance > distances[current_vertex]:
continue
for neighbor, weight in graph[current_vertex].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(queue, (distance, neighbor))
return distances[end]
graph = {
'A': {'B': 1, 'C': 4},
'B': {'A': 1, 'C': 2, 'D': 5},
'C': {'A': 4, 'B': 2, 'D': 1},
'D': {'B': 5, 'C': 1}
}
start = 'A'
end = 'D'
distance = dijkstra(graph, start, end)
print(f"Dijkstra distance from {start} to {end}: {distance}")
A*算法
A*算法是一种启发式搜索算法,通过结合启发式函数和路径成本函数来找到两点间的最短路径。它通常比Dijkstra算法更高效。
示例代码:
import heapq
def heuristic(a, b):
return abs(a[0] - b[0]) + abs(a[1] - b[1])
def a_star(graph, start, end):
queue = [(0, start)]
g_scores = {start: 0}
f_scores = {start: heuristic(start, end)}
while queue:
current_f, current = heapq.heappop(queue)
if current == end:
return g_scores[current]
for neighbor, weight in graph[current].items():
tentative_g_score = g_scores[current] + weight
if neighbor not in g_scores or tentative_g_score < g_scores[neighbor]:
g_scores[neighbor] = tentative_g_score
f_scores[neighbor] = tentative_g_score + heuristic(neighbor, end)
heapq.heappush(queue, (f_scores[neighbor], neighbor))
return float('inf')
graph = {
(0, 0): {(1, 0): 1, (0, 1): 1},
(1, 0): {(0, 0): 1, (1, 1): 1},
(0, 1): {(0, 0): 1, (1, 1): 1},
(1, 1): {(1, 0): 1, (0, 1): 1}
}
start = (0, 0)
end = (1, 1)
distance = a_star(graph, start, end)
print(f"A* distance from {start} to {end}: {distance}")
Floyd-Warshall算法
Floyd-Warshall算法是一种多源最短路径算法,适用于任意权重的图。它通过动态规划的方式计算所有节点对之间的最短路径。
示例代码:
def floyd_warshall(graph):
nodes = list(graph.keys())
distances = {node: {node: float('inf') for node in nodes} for node in nodes}
for node in nodes:
distances[node][node] = 0
for start, neighbors in graph.items():
for neighbor, weight in neighbors.items():
distances[start][neighbor] = weight
for k in nodes:
for i in nodes:
for j in nodes:
if distances[i][j] > distances[i][k] + distances[k][j]:
distances[i][j] = distances[i][k] + distances[k][j]
return distances
graph = {
'A': {'B': 1, 'C': 4},
'B': {'A': 1, 'C': 2, 'D': 5},
'C': {'A': 4, 'B': 2, 'D': 1},
'D': {'B': 5, 'C': 1}
}
distances = floyd_warshall(graph)
start = 'A'
end = 'D'
distance = distances[start][end]
print(f"Floyd-Warshall distance from {start} to {end}: {distance}")
通过上述几种方法,我们可以在Python中求解两点间的特定长路径。根据实际应用场景的不同,可以选择适合的算法和方法。
四、其他距离度量方法
除了欧几里得距离和曼哈顿距离,还有其他常用的距离度量方法,如切比雪夫距离、汉明距离等。这些距离度量方法在不同的应用场景中有着广泛的应用。
切比雪夫距离
切比雪夫距离用于度量棋盘上的移动距离,它计算的是各维度上坐标差的最大值。
切比雪夫距离公式为:
[
d = \max_{i=1}^{n} |x_{2i} – x_{1i}|
]
示例代码:
def chebyshev_distance(point1, point2):
return max(abs(point2[i] - point1[i]) for i in range(len(point1)))
point1 = (1, 2)
point2 = (4, 6)
distance = chebyshev_distance(point1, point2)
print(f"Chebyshev distance: {distance}")
汉明距离
汉明距离用于度量两个字符串或序列之间的差异,它计算的是对应位置上不同字符的数量。
汉明距离公式为:
[
d = \sum_{i=1}^{n} [x_{1i} \ne x_{2i}]
]
示例代码:
def hamming_distance(str1, str2):
if len(str1) != len(str2):
raise ValueError("Strings must be of the same length")
return sum(c1 != c2 for c1, c2 in zip(str1, str2))
str1 = "karolin"
str2 = "kathrin"
distance = hamming_distance(str1, str2)
print(f"Hamming distance: {distance}")
余弦相似度
余弦相似度用于度量两个向量之间的夹角余弦值,常用于文本相似度计算。其值在-1到1之间,值越大表示越相似。
余弦相似度公式为:
[
\cos(\theta) = \frac{\vec{A} \cdot \vec{B}}{||\vec{A}|| \cdot ||\vec{B}||}
]
示例代码:
import numpy as np
def cosine_similarity(vec1, vec2):
dot_product = np.dot(vec1, vec2)
norm_vec1 = np.linalg.norm(vec1)
norm_vec2 = np.linalg.norm(vec2)
return dot_product / (norm_vec1 * norm_vec2)
vec1 = np.array([1, 2, 3])
vec2 = np.array([4, 5, 6])
similarity = cosine_similarity(vec1, vec2)
print(f"Cosine similarity: {similarity}")
通过以上几种距离度量方法,我们可以根据不同的应用场景选择合适的方法来求解两点间的特定长路径。在实际应用中,结合多种方法往往能获得更好的效果。
相关问答FAQs:
如何在Python中计算两点之间的特定长度路径?
在Python中,可以使用图论算法来计算两点之间的特定长度路径。一种常用的方法是利用Dijkstra算法或A*算法,结合边的权重来限制路径长度。可以使用NetworkX库来构建图和计算路径,确保选择的路径符合特定长度。
可以使用哪些库来实现路径计算?
在Python中,NetworkX是最常用的图处理库,提供了丰富的图算法和数据结构,适合用于路径计算。此外,Scipy库中的空间数据处理功能也可以用来处理路径和距离计算。根据需求,可以选择合适的库来实现特定的路径计算功能。
如何处理路径计算中的权重和约束条件?
在路径计算中,边的权重通常代表了距离、时间或成本等因素。可以在构建图时为每条边指定权重,使用图算法时加入约束条件,如路径长度限制、经过特定点等。通过调整算法的参数和图的结构,可以实现复杂的路径计算需求。
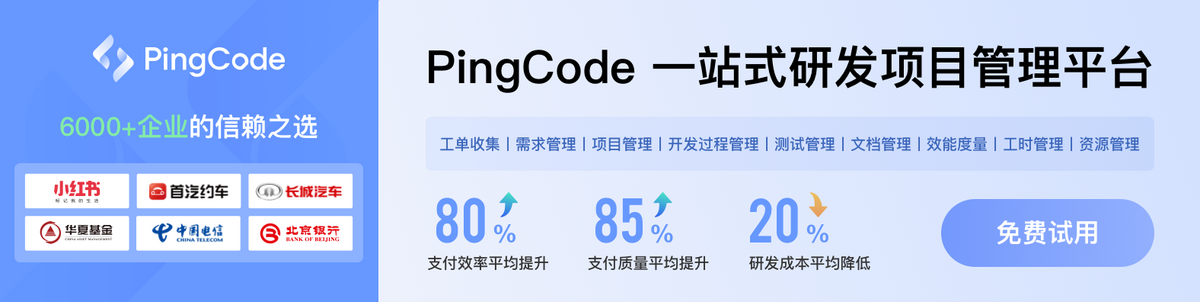