使用Python输出文本进度条的步骤包括:定义进度条函数、计算完成百分比、更新进度条、使用循环调用函数。 在这里,我们将重点介绍如何定义一个简单的进度条函数,并在实际应用中使用它。
在编写Python程序时,常常需要处理大数据集或执行长时间运行的任务。为了让用户了解程序的进度,显示一个进度条是非常有用的。通过以下几个步骤,我们可以轻松实现这一功能。
一、定义进度条函数
首先,我们需要定义一个函数,这个函数负责显示进度条。这个函数应该接受当前进度和总任务量作为参数,并在控制台中打印进度条。
import sys
import time
def print_progress_bar(iteration, total, prefix='', suffix='', decimals=1, length=50, fill='█'):
"""
Call in a loop to create terminal progress bar
:param iteration: Current iteration
:param total: Total iterations
:param prefix: Prefix string
:param suffix: Suffix string
:param decimals: Positive number of decimals in percent complete
:param length: Character length of bar
:param fill: Bar fill character
"""
percent = ("{0:." + str(decimals) + "f}").format(100 * (iteration / float(total)))
filled_length = int(length * iteration // total)
bar = fill * filled_length + '-' * (length - filled_length)
print(f'\r{prefix} |{bar}| {percent}% {suffix}', end='\r')
# Print New Line on Complete
if iteration == total:
print()
二、计算完成百分比
在进度条函数中,我们需要计算当前进度的百分比,并将其格式化为指定的小数位数。通过iteration
和total
参数计算完成百分比。
percent = ("{0:." + str(decimals) + "f}").format(100 * (iteration / float(total)))
三、更新进度条
根据当前进度和总任务量,计算进度条的填充长度,并更新进度条的显示内容。使用填充字符和空白字符构建进度条。
filled_length = int(length * iteration // total)
bar = fill * filled_length + '-' * (length - filled_length)
四、使用循环调用函数
在实际应用中,我们需要在一个循环中调用进度条函数,以显示任务的进度。以下是一个示例,用于模拟长时间任务的进度显示。
import time
A List of Items
items = list(range(0, 57))
l = len(items)
Initial call to print 0% progress
print_progress_bar(0, l, prefix='Progress:', suffix='Complete', length=50)
for i, item in enumerate(items):
# Do stuff...
time.sleep(0.1)
# Update Progress Bar
print_progress_bar(i + 1, l, prefix='Progress:', suffix='Complete', length=50)
五、进阶:多任务进度条
如果你需要同时显示多个任务的进度条,可以使用一个类来管理这些进度条。以下是一个示例,展示如何实现多任务进度条。
import threading
class MultiProgressBar:
def __init__(self, total_tasks):
self.total_tasks = total_tasks
self.current_task = 0
self.lock = threading.Lock()
def update(self, task_id, iteration, total, prefix='', suffix='', decimals=1, length=50, fill='█'):
with self.lock:
percent = ("{0:." + str(decimals) + "f}").format(100 * (iteration / float(total)))
filled_length = int(length * iteration // total)
bar = fill * filled_length + '-' * (length - filled_length)
print(f'\rTask {task_id + 1}/{self.total_tasks} |{bar}| {percent}% {suffix}', end='\r')
if iteration == total:
print()
def task_function(task_id, mpb):
total = 100
for i in range(total):
time.sleep(0.05)
mpb.update(task_id, i + 1, total, prefix='Progress:', suffix='Complete', length=50)
if __name__ == "__main__":
total_tasks = 3
mpb = MultiProgressBar(total_tasks)
threads = []
for task_id in range(total_tasks):
t = threading.Thread(target=task_function, args=(task_id, mpb))
threads.append(t)
t.start()
for t in threads:
t.join()
通过以上步骤,你可以轻松地在Python程序中实现进度条功能。无论是单任务还是多任务,进度条都可以为用户提供实时反馈,提高程序的用户体验。
相关问答FAQs:
如何在Python中创建动态进度条?
要创建动态进度条,可以使用sys.stdout
与time.sleep()
结合。通过在循环中输出带有一定格式的文本,可以实现进度条的效果。例如,使用print
函数打印进度符号,并通过\r
回到行首更新进度条。
使用Python进度条库有什么优势?
使用专门的进度条库如tqdm
,可以大大简化代码,提高可读性和可维护性。该库提供了丰富的功能,比如自动计算进度、估算剩余时间等,用户只需简单地将其包裹在迭代器外部即可。
在多线程或多进程环境中如何实现进度条?
在多线程或多进程的情况下,可以使用threading
或multiprocessing
模块结合进度条库来跟踪进度。通过共享变量或使用队列,可以在主线程中更新进度条,确保多个线程或进程的进度能够同步显示。
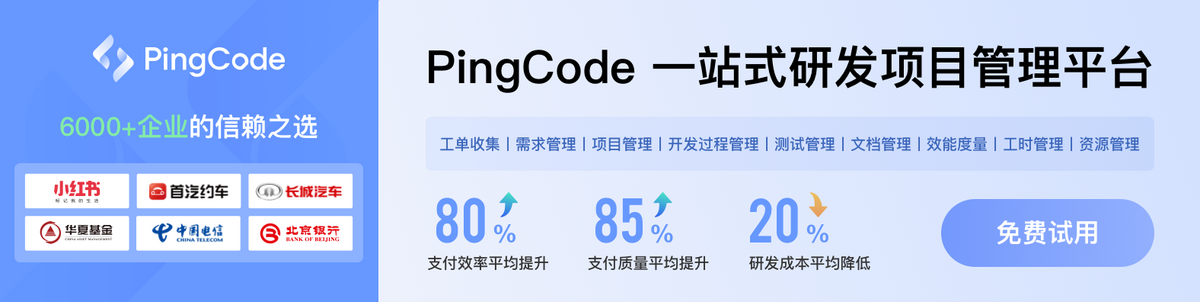