判断文本文件中有乱码的方法包括:使用特定编码读取文件、统计不可打印字符的数量、利用正则表达式检测、尝试多种编码进行读取。 其中,使用特定编码读取文件是最常见的方法之一。通过使用特定编码读取文件,可以判断文件是否能够正常解码,从而识别文件中是否存在乱码。下面将详细介绍这一方法。
使用特定编码读取文件:
在Python中,我们可以使用内置的open
函数来读取文件,并指定编码方式。常见的编码方式包括utf-8
、latin-1
等。我们可以尝试使用这些编码方式读取文件,并捕获解码过程中可能出现的异常。如果出现异常,则说明文件中可能存在乱码。以下是一个示例代码:
def check_for_garbled_text(file_path, encoding='utf-8'):
try:
with open(file_path, 'r', encoding=encoding) as file:
file.read()
print("File read successfully with encoding:", encoding)
return False
except UnicodeDecodeError:
print("Garbled text detected with encoding:", encoding)
return True
file_path = 'path/to/your/file.txt'
if check_for_garbled_text(file_path):
print("The file contains garbled text.")
else:
print("The file does not contain garbled text.")
在上述代码中,函数check_for_garbled_text
尝试使用指定的编码方式读取文件。如果文件能够成功读取,则表示文件中没有乱码;否则,会捕获UnicodeDecodeError
异常,表示文件中可能存在乱码。
接下来,我们将深入探讨其他判断文本文件中有乱码的方法。
统计不可打印字符的数量:
不可打印字符通常是乱码的一个标志。通过统计文件中不可打印字符的数量,可以判断文件是否包含乱码。Python内置的string
模块提供了printable
属性,可以用来检查字符是否可打印。以下是一个示例代码:
import string
def count_non_printable_chars(file_path, encoding='utf-8'):
non_printable_count = 0
with open(file_path, 'r', encoding=encoding) as file:
content = file.read()
for char in content:
if char not in string.printable:
non_printable_count += 1
return non_printable_count
file_path = 'path/to/your/file.txt'
non_printable_count = count_non_printable_chars(file_path)
if non_printable_count > 0:
print(f"The file contains {non_printable_count} non-printable characters.")
else:
print("The file does not contain any non-printable characters.")
在上述代码中,函数count_non_printable_chars
统计文件中不可打印字符的数量。如果数量大于0,则表示文件中可能存在乱码。
利用正则表达式检测:
正则表达式是一种强大的文本处理工具,可以用来检测文件中是否存在乱码。例如,可以使用正则表达式来匹配文件中是否存在连续的特殊字符或其他异常模式。以下是一个示例代码:
import re
def detect_garbled_text_with_regex(file_path, encoding='utf-8'):
pattern = re.compile(r'[^\w\s,.!?;:()\"\']{2,}')
with open(file_path, 'r', encoding=encoding) as file:
content = file.read()
matches = pattern.findall(content)
return matches
file_path = 'path/to/your/file.txt'
matches = detect_garbled_text_with_regex(file_path)
if matches:
print(f"The file contains garbled text: {matches}")
else:
print("The file does not contain any garbled text.")
在上述代码中,函数detect_garbled_text_with_regex
使用正则表达式来检测文件中是否存在连续的特殊字符。如果匹配到的模式不为空,则表示文件中可能存在乱码。
尝试多种编码进行读取:
有时候,文件中的乱码是由于使用了错误的编码方式读取文件造成的。可以尝试使用多种编码方式读取文件,并比较读取结果的合理性。例如,可以尝试使用utf-8
、latin-1
等编码方式读取文件,并检查文件内容是否符合预期。以下是一个示例代码:
def try_multiple_encodings(file_path, encodings=['utf-8', 'latin-1']):
for encoding in encodings:
try:
with open(file_path, 'r', encoding=encoding) as file:
content = file.read()
print(f"File read successfully with encoding: {encoding}")
print("Sample content:", content[:100])
return encoding
except UnicodeDecodeError:
print(f"Failed to read file with encoding: {encoding}")
return None
file_path = 'path/to/your/file.txt'
encoding = try_multiple_encodings(file_path)
if encoding:
print(f"The file was successfully read with encoding: {encoding}")
else:
print("Failed to read the file with all specified encodings.")
在上述代码中,函数try_multiple_encodings
尝试使用多种编码方式读取文件,并打印出读取成功的编码方式及文件内容样本。如果所有编码方式均失败,则表示文件中可能存在乱码。
通过上述方法,可以有效判断文本文件中是否存在乱码。根据实际情况选择合适的方法,可以帮助我们更好地处理和分析文本文件。
相关问答FAQs:
如何识别文本文件中的乱码?
识别文本文件中的乱码通常涉及检查文件的编码方式。如果文件的实际编码与读取时指定的编码不匹配,可能会导致乱码。建议使用Python的chardet
库进行编码检测,这样可以更准确地判断文件的编码格式,从而避免乱码。
在Python中,有哪些方法可以读取并检查文本文件的内容?
在Python中,可以使用内置的open()
函数来读取文本文件。使用with
语句打开文件后,可以逐行读取文件内容并检查每行是否包含非可打印字符,这些字符可能指示乱码的存在。此外,使用正则表达式也可以帮助识别意外的字符模式。
如何处理文本文件中的乱码问题?
处理乱码问题的有效方法包括重新编码文件。例如,可以使用iconv
命令行工具或Python中的codecs
模块来转换文件编码。此外,删除或替换无法识别的字符也是一种常见的解决方案。确保在处理文件时备份原始文件,以防数据丢失。
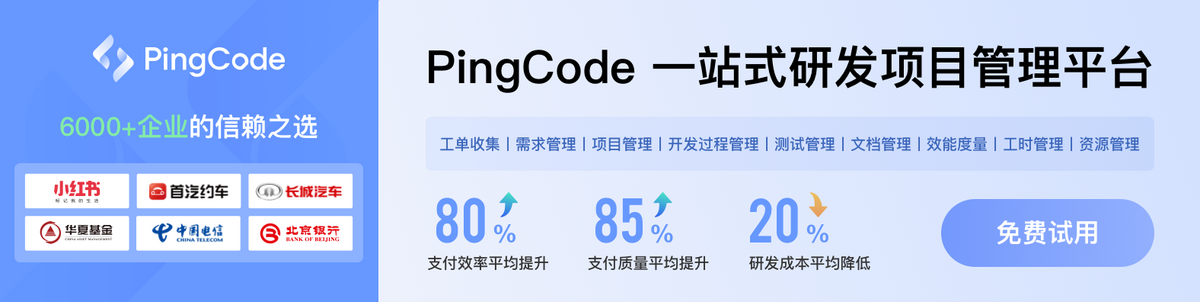